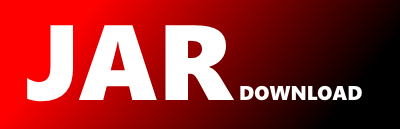
org.gradle.api.internal.changedetection.state.CurrentTaskExecution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.internal.changedetection.state;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSortedMap;
import com.google.common.collect.ImmutableSortedSet;
import org.gradle.api.NonNullApi;
import org.gradle.api.internal.OverlappingOutputs;
import org.gradle.internal.id.UniqueId;
import javax.annotation.Nullable;
@NonNullApi
public class CurrentTaskExecution extends AbstractTaskExecution {
private final ImmutableSet declaredOutputFilePaths;
private ImmutableSortedMap outputFilesSnapshot;
private final ImmutableSortedMap inputFilesSnapshot;
private FileCollectionSnapshot discoveredInputFilesSnapshot;
private final OverlappingOutputs detectedOverlappingOutputs;
private Boolean successful;
public CurrentTaskExecution(
UniqueId buildInvocationId,
ImplementationSnapshot taskImplementation,
ImmutableList taskActionImplementations,
ImmutableSortedMap inputProperties,
ImmutableSortedSet outputPropertyNames,
ImmutableSet declaredOutputFilePaths,
ImmutableSortedMap inputFilesSnapshot,
FileCollectionSnapshot discoveredInputFilesSnapshot,
ImmutableSortedMap outputFilesSnapshot,
@Nullable OverlappingOutputs detectedOverlappingOutputs
) {
super(buildInvocationId, taskImplementation, taskActionImplementations, inputProperties, outputPropertyNames);
this.declaredOutputFilePaths = declaredOutputFilePaths;
this.outputFilesSnapshot = outputFilesSnapshot;
this.inputFilesSnapshot = inputFilesSnapshot;
this.discoveredInputFilesSnapshot = discoveredInputFilesSnapshot;
this.detectedOverlappingOutputs = detectedOverlappingOutputs;
}
/**
* Returns the absolute path of every declared output file and directory.
* The returned set includes potentially missing files as well, and does
* not include the resolved contents of directories.
*/
public ImmutableSet getDeclaredOutputFilePaths() {
return declaredOutputFilePaths;
}
@Override
public boolean isSuccessful() {
return successful;
}
public void setSuccessful(boolean successful) {
this.successful = successful;
}
@Override
public ImmutableSortedMap getOutputFilesSnapshot() {
return outputFilesSnapshot;
}
public void setOutputFilesSnapshot(ImmutableSortedMap outputFilesSnapshot) {
this.outputFilesSnapshot = outputFilesSnapshot;
}
@Override
public ImmutableSortedMap getInputFilesSnapshot() {
return inputFilesSnapshot;
}
@Override
public FileCollectionSnapshot getDiscoveredInputFilesSnapshot() {
return discoveredInputFilesSnapshot;
}
public void setDiscoveredInputFilesSnapshot(FileCollectionSnapshot discoveredInputFilesSnapshot) {
this.discoveredInputFilesSnapshot = discoveredInputFilesSnapshot;
}
@Nullable
public OverlappingOutputs getDetectedOverlappingOutputs() {
return detectedOverlappingOutputs;
}
public HistoricalTaskExecution archive() {
return new HistoricalTaskExecution(
getBuildInvocationId(),
getTaskImplementation(),
getTaskActionImplementations(),
getInputProperties(),
getOutputPropertyNamesForCacheKey(),
inputFilesSnapshot,
discoveredInputFilesSnapshot,
outputFilesSnapshot,
successful
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy