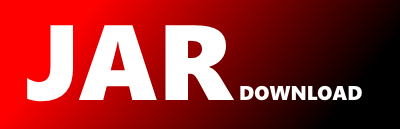
org.gradle.api.internal.tasks.compile.daemon.AbstractDaemonCompiler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.internal.tasks.compile.daemon;
import com.google.common.collect.Lists;
import org.gradle.api.tasks.WorkResult;
import org.gradle.api.tasks.compile.BaseForkOptions;
import org.gradle.internal.UncheckedException;
import org.gradle.language.base.internal.compile.CompileSpec;
import org.gradle.language.base.internal.compile.Compiler;
import org.gradle.workers.internal.DaemonForkOptions;
import org.gradle.workers.internal.DefaultWorkResult;
import org.gradle.workers.internal.SimpleActionExecutionSpec;
import org.gradle.workers.internal.Worker;
import org.gradle.workers.internal.WorkerFactory;
import javax.inject.Inject;
import java.util.Set;
import java.util.concurrent.Callable;
import static org.gradle.process.internal.util.MergeOptionsUtil.mergeHeapSize;
import static org.gradle.process.internal.util.MergeOptionsUtil.normalized;
public abstract class AbstractDaemonCompiler implements Compiler {
private final Compiler delegate;
private final WorkerFactory workerFactory;
public AbstractDaemonCompiler(Compiler delegate, WorkerFactory workerFactory) {
this.delegate = delegate;
this.workerFactory = workerFactory;
}
public Compiler getDelegate() {
return delegate;
}
@Override
public WorkResult execute(T spec) {
DaemonForkOptions daemonForkOptions = toDaemonForkOptions(spec);
Worker worker = workerFactory.getWorker(daemonForkOptions);
DefaultWorkResult result = worker.execute(new SimpleActionExecutionSpec(CompilerCallable.class, "compiler daemon", new Object[] {delegate, spec}));
if (result.isSuccess()) {
return result;
} else {
throw UncheckedException.throwAsUncheckedException(result.getException());
}
}
protected abstract DaemonForkOptions toDaemonForkOptions(T spec);
protected BaseForkOptions mergeForkOptions(BaseForkOptions left, BaseForkOptions right) {
BaseForkOptions merged = new BaseForkOptions();
merged.setMemoryInitialSize(mergeHeapSize(left.getMemoryInitialSize(), right.getMemoryInitialSize()));
merged.setMemoryMaximumSize(mergeHeapSize(left.getMemoryMaximumSize(), right.getMemoryMaximumSize()));
Set mergedJvmArgs = normalized(left.getJvmArgs());
mergedJvmArgs.addAll(normalized(right.getJvmArgs()));
merged.setJvmArgs(Lists.newArrayList(mergedJvmArgs));
return merged;
}
private static class CompilerCallable implements Callable {
private final Compiler compiler;
private final T compileSpec;
@Inject
public CompilerCallable(Compiler compiler, T compileSpec) {
this.compiler = compiler;
this.compileSpec = compileSpec;
}
@Override
public WorkResult call() throws Exception {
return compiler.execute(compileSpec);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy