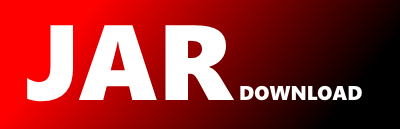
org.gradle.integtests.tooling.r40.PluginApplicationBuildProgressCrossVersionSpec.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.integtests.tooling.r40
import org.gradle.integtests.tooling.fixture.ProgressEvents
import org.gradle.integtests.tooling.fixture.TargetGradleVersion
import org.gradle.integtests.tooling.fixture.ToolingApiSpecification
import org.gradle.tooling.BuildException
import org.gradle.tooling.ProjectConnection
import org.gradle.util.Requires
import org.gradle.util.TestPrecondition
import spock.lang.Issue
import static org.gradle.integtests.fixtures.RepoScriptBlockUtil.gradlePluginRepositoryMirrorUrl
@TargetGradleVersion(">=4.0 <5.1")
class PluginApplicationBuildProgressCrossVersionSpec extends ToolingApiSpecification {
def "generates plugin application events for single project build"() {
given:
def events = ProgressEvents.create()
settingsFile << "rootProject.name = 'single'"
buildFile << """
apply plugin: 'java'
"""
when:
withConnection {
ProjectConnection connection -> connection.newBuild().addProgressListener(events).run()
}
then:
events.assertIsABuild()
def configureRootProject = events.operation("Configure project :")
def applyBuildGradle = events.operation("Apply script build.gradle to root project 'single'")
def help = events.operation("Apply plugin org.gradle.help-tasks to root project 'single'")
def java = events.operation("Apply plugin org.gradle.java to root project 'single'")
def javaBase = events.operation("Apply plugin org.gradle.api.plugins.JavaBasePlugin to root project 'single'")
def base = events.operation("Apply plugin org.gradle.api.plugins.BasePlugin to root project 'single'")
help.parent == configureRootProject
java.parent == applyBuildGradle
javaBase.parent == java
base.parent == javaBase
}
def "generates plugin application events for core plugin applied through plugins dsl"() {
given:
def events = ProgressEvents.create()
settingsFile << "rootProject.name = 'single'"
buildFile << """
plugins {
id 'java'
}
"""
when:
withConnection {
ProjectConnection connection -> connection.newBuild().addProgressListener(events).run()
}
then:
events.assertIsABuild()
def configureRootProject = events.operation("Configure project :")
def applyBuildGradle = events.operation("Apply script build.gradle to root project 'single'")
def help = events.operation("Apply plugin org.gradle.help-tasks to root project 'single'")
def java = events.operation("Apply plugin org.gradle.java to root project 'single'")
def javaBase = events.operation("Apply plugin org.gradle.api.plugins.JavaBasePlugin to root project 'single'")
def base = events.operation("Apply plugin org.gradle.api.plugins.BasePlugin to root project 'single'")
help.parent == configureRootProject
java.parent == applyBuildGradle
javaBase.parent == java
base.parent == javaBase
}
@Requires(TestPrecondition.JDK8_OR_LATER)
@Issue('https://github.com/gradle/gradle-private/issues/1341')
def "generates plugin application events for community plugin applied through plugins dsl"() {
given:
def events = ProgressEvents.create()
settingsFile << "rootProject.name = 'single'"
buildFile << """
plugins {
id "org.gradle.hello-world" version "0.2"
}
"""
when:
withConnection {
ProjectConnection connection -> connection.newBuild().withArguments("-Dorg.gradle.internal.plugins.portal.url.override=${gradlePluginRepositoryMirrorUrl()}").addProgressListener(events).run()
}
then:
events.assertIsABuild()
def configureRootProject = events.operation("Configure project :")
def applyBuildGradle = events.operation("Apply script build.gradle to root project 'single'")
def help = events.operation("Apply plugin org.gradle.help-tasks to root project 'single'")
def helloWorld = events.operation("Apply plugin org.gradle.hello-world to root project 'single'")
help.parent == configureRootProject
helloWorld.parent == applyBuildGradle
helloWorld.descriptor.name == "Apply plugin org.gradle.hello-world"
}
def "generates plugin application events for plugin applied in settings script"() {
given:
def events = ProgressEvents.create()
settingsFile << """
rootProject.name = 'single'
apply plugin: ExamplePlugin
class ExamplePlugin implements Plugin
© 2015 - 2025 Weber Informatics LLC | Privacy Policy