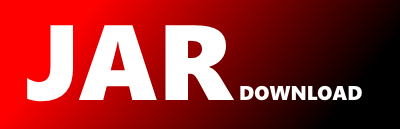
org.gradle.language.java.internal.JavaLanguagePluginServiceRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.language.java.internal;
import org.gradle.api.internal.cache.StringInterner;
import org.gradle.api.internal.component.ArtifactType;
import org.gradle.api.internal.component.ComponentTypeRegistry;
import org.gradle.api.internal.file.FileOperations;
import org.gradle.api.internal.tasks.compile.incremental.IncrementalCompilerFactory;
import org.gradle.api.internal.tasks.compile.incremental.cache.GeneralCompileCaches;
import org.gradle.api.internal.tasks.compile.processing.AnnotationProcessorDetector;
import org.gradle.api.internal.tasks.compile.tooling.JavaCompileTaskSuccessResultPostProcessor;
import org.gradle.api.logging.Logging;
import org.gradle.api.logging.configuration.LoggingConfiguration;
import org.gradle.api.logging.configuration.ShowStacktrace;
import org.gradle.cache.internal.FileContentCacheFactory;
import org.gradle.initialization.BuildEventConsumer;
import org.gradle.internal.hash.FileHasher;
import org.gradle.internal.hash.StreamHasher;
import org.gradle.internal.operations.BuildOperationExecutor;
import org.gradle.internal.service.ServiceRegistration;
import org.gradle.internal.service.scopes.AbstractPluginServiceRegistry;
import org.gradle.internal.snapshot.FileSystemSnapshotter;
import org.gradle.jvm.JvmLibrary;
import org.gradle.language.java.artifact.JavadocArtifact;
import org.gradle.tooling.events.OperationType;
import org.gradle.tooling.internal.provider.BuildClientSubscriptions;
import org.gradle.tooling.internal.provider.SubscribableBuildActionRunnerRegistration;
import java.util.Collections;
import static java.util.Collections.emptyList;
public class JavaLanguagePluginServiceRegistry extends AbstractPluginServiceRegistry {
@Override
public void registerGlobalServices(ServiceRegistration registration) {
registration.addProvider(new JavaGlobalScopeServices());
}
@Override
public void registerGradleServices(ServiceRegistration registration) {
registration.addProvider(new JavaGradleScopeServices());
}
@Override
public void registerProjectServices(ServiceRegistration registration) {
registration.addProvider(new JavaProjectScopeServices());
}
private static class JavaGlobalScopeServices {
SubscribableBuildActionRunnerRegistration createJavaSubscribableBuildActionRunnerRegistration(final JavaCompileTaskSuccessResultPostProcessor factory) {
return new SubscribableBuildActionRunnerRegistration() {
@Override
public Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy