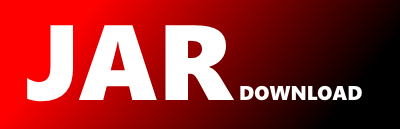
org.gradle.tooling.internal.consumer.loader.DefaultToolingImplementationLoaderTest.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.tooling.internal.consumer.loader
import org.gradle.initialization.BuildCancellationToken
import org.gradle.internal.actor.ActorFactory
import org.gradle.internal.classloader.ClasspathUtil
import org.gradle.internal.classpath.ClassPath
import org.gradle.internal.classpath.DefaultClassPath
import org.gradle.internal.logging.progress.ProgressLoggerFactory
import org.gradle.test.fixtures.file.TestNameTestDirectoryProvider
import org.gradle.tooling.internal.consumer.ConnectionParameters
import org.gradle.tooling.internal.consumer.Distribution
import org.gradle.tooling.internal.consumer.connection.NoToolingApiConnection
import org.gradle.tooling.internal.consumer.connection.ParameterAcceptingConsumerConnection
import org.gradle.tooling.internal.consumer.connection.PhasedActionAwareConsumerConnection
import org.gradle.tooling.internal.consumer.connection.TestExecutionConsumerConnection
import org.gradle.tooling.internal.consumer.connection.UnsupportedOlderVersionConnection
import org.gradle.tooling.internal.protocol.BuildActionRunner
import org.gradle.tooling.internal.protocol.BuildExceptionVersion1
import org.gradle.tooling.internal.protocol.BuildOperationParametersVersion1
import org.gradle.tooling.internal.protocol.BuildParameters
import org.gradle.tooling.internal.protocol.BuildParametersVersion1
import org.gradle.tooling.internal.protocol.BuildResult
import org.gradle.tooling.internal.protocol.ConfigurableConnection
import org.gradle.tooling.internal.protocol.ConnectionMetaDataVersion1
import org.gradle.tooling.internal.protocol.ConnectionVersion4
import org.gradle.tooling.internal.protocol.InternalBuildAction
import org.gradle.tooling.internal.protocol.InternalBuildActionExecutor
import org.gradle.tooling.internal.protocol.InternalBuildActionFailureException
import org.gradle.tooling.internal.protocol.InternalBuildActionVersion2
import org.gradle.tooling.internal.protocol.InternalBuildCancelledException
import org.gradle.tooling.internal.protocol.InternalBuildProgressListener
import org.gradle.tooling.internal.protocol.InternalCancellableConnection
import org.gradle.tooling.internal.protocol.InternalCancellationToken
import org.gradle.tooling.internal.protocol.InternalConnection
import org.gradle.tooling.internal.protocol.InternalParameterAcceptingConnection
import org.gradle.tooling.internal.protocol.InternalPhasedAction
import org.gradle.tooling.internal.protocol.InternalPhasedActionConnection
import org.gradle.tooling.internal.protocol.InternalUnsupportedModelException
import org.gradle.tooling.internal.protocol.ModelBuilder
import org.gradle.tooling.internal.protocol.ModelIdentifier
import org.gradle.tooling.internal.protocol.PhasedActionResultListener
import org.gradle.tooling.internal.protocol.ProjectVersion3
import org.gradle.tooling.internal.protocol.ShutdownParameters
import org.gradle.tooling.internal.protocol.StoppableConnection
import org.gradle.tooling.internal.protocol.exceptions.InternalUnsupportedBuildArgumentException
import org.gradle.tooling.internal.protocol.test.InternalTestExecutionConnection
import org.gradle.tooling.internal.protocol.test.InternalTestExecutionRequest
import org.gradle.util.GradleVersion
import org.junit.Rule
import org.slf4j.Logger
import spock.lang.Specification
class DefaultToolingImplementationLoaderTest extends Specification {
@Rule
public final TestNameTestDirectoryProvider tmpDir = new TestNameTestDirectoryProvider()
Distribution distribution = Mock()
ProgressLoggerFactory loggerFactory = Mock()
InternalBuildProgressListener progressListener = Mock()
ConnectionParameters connectionParameters = Stub() {
getVerboseLogging() >> true
}
File userHomeDir = Mock()
final BuildCancellationToken cancellationToken = Mock()
final loader = new DefaultToolingImplementationLoader()
def "locates connection implementation using meta-inf service then instantiates and configures the connection"() {
given:
distribution.getToolingImplementationClasspath(loggerFactory, progressListener, userHomeDir, cancellationToken) >> DefaultClassPath.of(
getToolingApiResourcesDir(connectionImplementation),
ClasspathUtil.getClasspathForClass(TestConnection.class),
ClasspathUtil.getClasspathForClass(ActorFactory.class),
ClasspathUtil.getClasspathForClass(Logger.class),
ClasspathUtil.getClasspathForClass(GroovyObject.class),
ClasspathUtil.getClasspathForClass(GradleVersion.class))
when:
def consumerConnection = loader.create(distribution, loggerFactory, progressListener, connectionParameters, cancellationToken).delegate
then:
consumerConnection.delegate.class != connectionImplementation //different classloaders
consumerConnection.delegate.class.name == connectionImplementation.name
consumerConnection.delegate.configured
where:
connectionImplementation | adapter
TestConnection.class | PhasedActionAwareConsumerConnection.class
TestR44Connection.class | ParameterAcceptingConsumerConnection.class
TestR26Connection.class | TestExecutionConsumerConnection.class
}
def "locates connection implementation using meta-inf service for deprecated connection"() {
given:
distribution.getToolingImplementationClasspath(loggerFactory, progressListener, userHomeDir, cancellationToken) >> DefaultClassPath.of(
getToolingApiResourcesDir(connectionImplementation),
ClasspathUtil.getClasspathForClass(TestConnection.class),
ClasspathUtil.getClasspathForClass(ActorFactory.class),
ClasspathUtil.getClasspathForClass(Logger.class),
ClasspathUtil.getClasspathForClass(GroovyObject.class),
ClasspathUtil.getClasspathForClass(GradleVersion.class))
when:
def adaptedConnection = loader.create(distribution, loggerFactory, progressListener, connectionParameters, cancellationToken)
then:
adaptedConnection.class == UnsupportedOlderVersionConnection.class
where:
connectionImplementation | _
TestR10M3Connection.class | _
TestR10M8Connection.class | _
TestR12Connection.class | _
TestR16Connection.class | _
TestR18Connection.class | _
TestR21Connection.class | _
TestR22Connection.class | _
}
private getToolingApiResourcesDir(Class implementation) {
tmpDir.file("META-INF/services/org.gradle.tooling.internal.protocol.ConnectionVersion4") << implementation.name
return tmpDir.testDirectory;
}
def "creates broken connection when resource not found"() {
def loader = new DefaultToolingImplementationLoader()
given:
distribution.getToolingImplementationClasspath(loggerFactory, progressListener, userHomeDir, cancellationToken) >> ClassPath.EMPTY
expect:
loader.create(distribution, loggerFactory, progressListener, connectionParameters, cancellationToken) instanceof NoToolingApiConnection
}
}
class TestMetaData implements ConnectionMetaDataVersion1 {
private final String version;
TestMetaData(String version) {
this.version = version
}
String getVersion() {
return version
}
String getDisplayName() {
throw new UnsupportedOperationException()
}
}
class TestConnection extends TestR44Connection implements InternalPhasedActionConnection {
@Override
BuildResult> run(InternalPhasedAction internalPhasedAction, PhasedActionResultListener listener, InternalCancellationToken cancellationToken, BuildParameters operationParameters)
throws BuildExceptionVersion1, InternalUnsupportedBuildArgumentException, InternalBuildActionFailureException, InternalBuildCancelledException, IllegalStateException {
throw new UnsupportedOperationException()
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('4.8')
}
}
class TestR44Connection extends TestR26Connection implements InternalParameterAcceptingConnection {
@Override
BuildResult run(InternalBuildActionVersion2 action, InternalCancellationToken cancellationToken, BuildParameters operationParameters)
throws BuildExceptionVersion1, InternalUnsupportedBuildArgumentException, InternalBuildActionFailureException, IllegalStateException {
throw new UnsupportedOperationException();
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('4.4')
}
}
class TestR26Connection extends TestR22Connection implements InternalTestExecutionConnection {
@Override
BuildResult> runTests(InternalTestExecutionRequest testExecutionRequest, InternalCancellationToken cancellationToken, BuildParameters operationParameters) {
throw new UnsupportedOperationException()
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('2.6')
}
}
class TestR22Connection extends TestR21Connection implements StoppableConnection {
@Override
void shutdown(ShutdownParameters parameters) {
throw new UnsupportedOperationException()
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('2.2')
}
}
class TestR21Connection extends TestR18Connection implements InternalCancellableConnection {
@Override
BuildResult> getModel(ModelIdentifier modelIdentifier, InternalCancellationToken cancellationToken, BuildParameters operationParameters)
throws BuildExceptionVersion1, InternalUnsupportedModelException, InternalUnsupportedBuildArgumentException, IllegalStateException {
throw new UnsupportedOperationException();
}
@Override
def BuildResult run(InternalBuildAction action, InternalCancellationToken cancellationToken, BuildParameters operationParameters)
throws BuildExceptionVersion1, InternalUnsupportedBuildArgumentException, InternalBuildActionFailureException, IllegalStateException {
throw new UnsupportedOperationException();
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('2.1')
}
}
class TestR18Connection extends TestR16Connection implements InternalBuildActionExecutor {
def BuildResult run(InternalBuildAction action, BuildParameters operationParameters) throws BuildExceptionVersion1, InternalUnsupportedBuildArgumentException, IllegalStateException {
throw new UnsupportedOperationException()
}
ConnectionMetaDataVersion1 getMetaData() {
return new TestMetaData('1.8')
}
}
class TestR16Connection extends TestR12Connection implements ModelBuilder {
BuildResult
© 2015 - 2025 Weber Informatics LLC | Privacy Policy