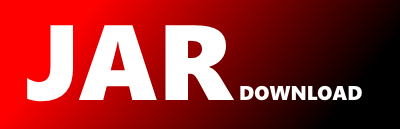
org.gradle.process.internal.worker.GradleWorkerMain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.process.internal.worker;
import org.gradle.internal.classloader.FilteringClassLoader;
import org.gradle.process.internal.streams.EncodedStream;
import java.io.DataInputStream;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
/**
* The main entry point for a worker process that is using the system ClassLoader strategy. Reads worker configuration and a serialized worker action from stdin,
* sets up the worker ClassLoader, and then delegates to {@link org.gradle.process.internal.worker.child.SystemApplicationClassLoaderWorker} to deserialize and execute the action.
*/
public class GradleWorkerMain {
public void run() throws Exception {
DataInputStream instr = new DataInputStream(new EncodedStream.EncodedInput(System.in));
// Read shared packages
int sharedPackagesCount = instr.readInt();
List sharedPackages = new ArrayList(sharedPackagesCount);
for (int i = 0; i < sharedPackagesCount; i++) {
sharedPackages.add(instr.readUTF());
}
// Read worker implementation classpath
int classPathLength = instr.readInt();
URL[] implementationClassPath = new URL[classPathLength];
for (int i = 0; i < classPathLength; i++) {
String url = instr.readUTF();
implementationClassPath[i] = new URL(url);
}
ClassLoader implementationClassLoader;
if (classPathLength > 0) {
// Set up worker ClassLoader
FilteringClassLoader.Spec filteringClassLoaderSpec = new FilteringClassLoader.Spec();
for (String sharedPackage : sharedPackages) {
filteringClassLoaderSpec.allowPackage(sharedPackage);
}
FilteringClassLoader filteringClassLoader = new FilteringClassLoader(getClass().getClassLoader(), filteringClassLoaderSpec);
implementationClassLoader = new URLClassLoader(implementationClassPath, filteringClassLoader);
} else {
// If no implementation classpath has been provided, just use the application classloader
implementationClassLoader = getClass().getClassLoader();
}
Class extends Callable> workerClass = implementationClassLoader.loadClass("org.gradle.process.internal.worker.child.SystemApplicationClassLoaderWorker").asSubclass(Callable.class);
Callable main = workerClass.getConstructor(DataInputStream.class).newInstance(instr);
main.call();
}
public static void main(String[] args) {
try {
new GradleWorkerMain().run();
System.exit(0);
} catch (Throwable throwable) {
throwable.printStackTrace(System.err);
System.exit(1);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy