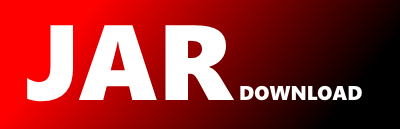
org.gradle.api.internal.OverlappingOutputs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.internal;
import com.google.common.collect.ImmutableSortedMap;
import org.gradle.internal.file.FileType;
import org.gradle.internal.fingerprint.FileCollectionFingerprint;
import org.gradle.internal.fingerprint.FileSystemLocationFingerprint;
import org.gradle.internal.hash.HashCode;
import org.gradle.internal.snapshot.DirectorySnapshot;
import org.gradle.internal.snapshot.FileSystemLocationSnapshot;
import org.gradle.internal.snapshot.FileSystemSnapshot;
import org.gradle.internal.snapshot.FileSystemSnapshotVisitor;
import javax.annotation.Nullable;
import java.util.Map;
import java.util.Optional;
public class OverlappingOutputs {
private final String propertyName;
private final String overlappedFilePath;
public OverlappingOutputs(String propertyName, String overlappedFilePath) {
this.propertyName = propertyName;
this.overlappedFilePath = overlappedFilePath;
}
public static Optional detect(ImmutableSortedMap previous, ImmutableSortedMap current) {
for (Map.Entry entry : current.entrySet()) {
String propertyName = entry.getKey();
FileSystemSnapshot beforeExecution = entry.getValue();
FileCollectionFingerprint afterPreviousExecution = getFingerprintAfterPreviousExecution(previous, propertyName);
OverlappingOutputs overlappingOutputs = OverlappingOutputs.detect(propertyName, afterPreviousExecution, beforeExecution);
if (overlappingOutputs != null) {
return Optional.of(overlappingOutputs);
}
}
return Optional.empty();
}
private static FileCollectionFingerprint getFingerprintAfterPreviousExecution(@Nullable ImmutableSortedMap previous, String propertyName) {
if (previous != null) {
FileCollectionFingerprint afterPreviousExecution = previous.get(propertyName);
if (afterPreviousExecution != null) {
return afterPreviousExecution;
}
}
return FileCollectionFingerprint.EMPTY;
}
@Nullable
private static OverlappingOutputs detect(String propertyName, FileCollectionFingerprint previous, FileSystemSnapshot before) {
Map previousFingerprints = previous.getFingerprints();
OverlappingOutputsDetectingVisitor outputsDetectingVisitor = new OverlappingOutputsDetectingVisitor(previousFingerprints);
before.accept(outputsDetectingVisitor);
String overlappingPath = outputsDetectingVisitor.getOverlappingPath();
return overlappingPath == null ? null : new OverlappingOutputs(propertyName, overlappingPath);
}
private static boolean changedSincePreviousExecution(HashCode contentHash, HashCode previousContentHash) {
// _changed_ since last execution, possibly by another task
return !contentHash.equals(previousContentHash);
}
private static boolean createdSincePreviousExecution(@Nullable HashCode previousContentHash) {
// created since last execution, possibly by another task
return previousContentHash == null;
}
public String getPropertyName() {
return propertyName;
}
public String getOverlappedFilePath() {
return overlappedFilePath;
}
public String toString() {
return String.format("output property '%s' with path '%s'", propertyName, overlappedFilePath);
}
private static class OverlappingOutputsDetectingVisitor implements FileSystemSnapshotVisitor {
private final Map previousFingerprints;
private int treeDepth = 0;
private String overlappingPath;
public OverlappingOutputsDetectingVisitor(Map previousFingerprints) {
this.previousFingerprints = previousFingerprints;
}
@Override
public boolean preVisitDirectory(DirectorySnapshot directorySnapshot) {
treeDepth++;
if (overlappingPath == null) {
overlappingPath = detectOverlappingPath(directorySnapshot);
}
return overlappingPath == null;
}
@Override
public void visitFile(FileSystemLocationSnapshot fileSnapshot) {
if (overlappingPath == null) {
overlappingPath = detectOverlappingPath(fileSnapshot);
}
}
@Override
public void postVisitDirectory(DirectorySnapshot directorySnapshot) {
treeDepth--;
}
@Nullable
private String detectOverlappingPath(FileSystemLocationSnapshot beforeSnapshot) {
String path = beforeSnapshot.getAbsolutePath();
HashCode contentHash = beforeSnapshot.getHash();
FileSystemLocationFingerprint previousFingerprint = previousFingerprints.get(path);
HashCode previousContentHash = previousFingerprint == null ? null : previousFingerprint.getNormalizedContentHash();
// Missing files can be ignored
if (!isRoot() || beforeSnapshot.getType() != FileType.Missing) {
if (createdSincePreviousExecution(previousContentHash)
|| (beforeSnapshot.getType() != previousFingerprint.getType())
// The fingerprint hashes for non-regular files are slightly different to the snapshot hashes, we only need to compare them for regular files
|| (beforeSnapshot.getType() == FileType.RegularFile && changedSincePreviousExecution(contentHash, previousContentHash))) {
return path;
}
}
return null;
}
private boolean isRoot() {
return treeDepth == 0;
}
@Nullable
public String getOverlappingPath() {
return overlappingPath;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy