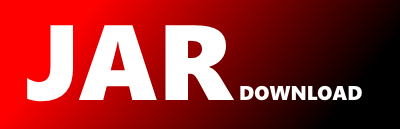
org.gradle.internal.classpath.Instrumented Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.internal.classpath;
import com.google.common.collect.AbstractIterator;
import org.codehaus.groovy.runtime.callsite.AbstractCallSite;
import org.codehaus.groovy.runtime.callsite.CallSite;
import org.codehaus.groovy.runtime.callsite.CallSiteArray;
import org.codehaus.groovy.runtime.wrappers.Wrapper;
import javax.annotation.Nullable;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Function;
public class Instrumented {
private static final Listener NO_OP = (key, value, consumer) -> {
};
private static final AtomicReference LISTENER = new AtomicReference<>(NO_OP);
public static void setListener(Listener listener) {
LISTENER.set(listener);
}
public static void discardListener() {
LISTENER.set(NO_OP);
}
// Called by generated code
@SuppressWarnings("unused")
public static void groovyCallSites(CallSiteArray array) {
for (CallSite callSite : array.array) {
switch (callSite.getName()) {
case "getProperty":
array.array[callSite.getIndex()] = new SystemPropertyCallSite(callSite);
break;
case "properties":
array.array[callSite.getIndex()] = new SystemPropertiesCallSite(callSite);
break;
case "getInteger":
array.array[callSite.getIndex()] = new IntegerSystemPropertyCallSite(callSite);
break;
case "getLong":
array.array[callSite.getIndex()] = new LongSystemPropertyCallSite(callSite);
break;
case "getBoolean":
array.array[callSite.getIndex()] = new BooleanSystemPropertyCallSite(callSite);
break;
}
}
}
// Called by generated code.
public static String systemProperty(String key, String consumer) {
return systemProperty(key, null, consumer);
}
// Called by generated code.
public static String systemProperty(String key, @Nullable String defaultValue, String consumer) {
String value = System.getProperty(key);
LISTENER.get().systemPropertyQueried(key, value, consumer);
if (value == null) {
return defaultValue;
}
return value;
}
// Called by generated code.
public static Properties systemProperties(String consumer) {
return new DecoratingProperties(System.getProperties(), consumer);
}
// Called by generated code.
public static Integer getInteger(String key, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Integer.getInteger(key);
}
// Called by generated code.
public static Integer getInteger(String key, int defaultValue, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Integer.getInteger(key, defaultValue);
}
// Called by generated code.
public static Integer getInteger(String key, Integer defaultValue, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Integer.getInteger(key, defaultValue);
}
// Called by generated code.
public static Long getLong(String key, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Long.getLong(key);
}
// Called by generated code.
public static Long getLong(String key, long defaultValue, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Long.getLong(key, defaultValue);
}
// Called by generated code.
public static Long getLong(String key, Long defaultValue, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Long.getLong(key, defaultValue);
}
// Called by generated code.
public static boolean getBoolean(String key, String consumer) {
LISTENER.get().systemPropertyQueried(key, System.getProperty(key), consumer);
return Boolean.getBoolean(key);
}
private static Object unwrap(Object obj) {
if (obj instanceof Wrapper) {
return ((Wrapper) obj).unwrap();
}
return obj;
}
private static String convertToString(Object arg) {
if (arg instanceof CharSequence) {
return ((CharSequence) arg).toString();
}
return (String) arg;
}
public interface Listener {
/**
* @param consumer The name of the class that is reading the property value
*/
void systemPropertyQueried(String key, @Nullable Object value, String consumer);
}
private static class DecoratingProperties extends Properties {
private final String consumer;
private final Properties delegate;
public DecoratingProperties(Properties delegate, String consumer) {
this.consumer = consumer;
this.delegate = delegate;
}
@Override
public Enumeration> propertyNames() {
return delegate.propertyNames();
}
@Override
public Set stringPropertyNames() {
return delegate.stringPropertyNames();
}
@Override
public int size() {
return delegate.size();
}
@Override
public boolean isEmpty() {
return delegate.isEmpty();
}
@Override
public Enumeration
© 2015 - 2025 Weber Informatics LLC | Privacy Policy