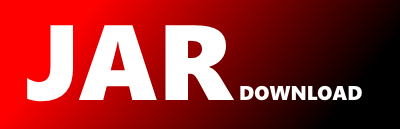
org.gradle.api.plugins.quality.PmdExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.plugins.quality;
import org.gradle.api.Incubating;
import org.gradle.api.Project;
import org.gradle.api.file.ConfigurableFileCollection;
import org.gradle.api.file.FileCollection;
import org.gradle.api.provider.Property;
import org.gradle.api.resources.TextResource;
import javax.annotation.Nullable;
import java.util.Arrays;
import java.util.List;
/**
* Configuration options for the PMD plugin.
*
* @see PmdPlugin
*/
public class PmdExtension extends CodeQualityExtension {
private final Project project;
private List ruleSets;
private TargetJdk targetJdk;
private TextResource ruleSetConfig;
private ConfigurableFileCollection ruleSetFiles;
private boolean consoleOutput;
private final Property rulesMinimumPriority;
private final Property maxFailures;
private final Property incrementalAnalysis;
private final Property threads;
public PmdExtension(Project project) {
this.project = project;
this.rulesMinimumPriority = project.getObjects().property(Integer.class).convention(5);
this.incrementalAnalysis = project.getObjects().property(Boolean.class).convention(true);
this.maxFailures = project.getObjects().property(Integer.class).convention(0);
this.threads = project.getObjects().property(Integer.class).convention(1);
}
/**
* The built-in rule sets to be used. See the official list of built-in rule sets.
*
*
* ruleSets = ["category/java/errorprone.xml", "category/java/bestpractices.xml"]
*
*/
public List getRuleSets() {
return ruleSets;
}
/**
* The built-in rule sets to be used. See the official list of built-in rule sets.
*
*
* ruleSets = ["category/java/errorprone.xml", "category/java/bestpractices.xml"]
*
*/
public void setRuleSets(List ruleSets) {
this.ruleSets = ruleSets;
}
/**
* Convenience method for adding rule sets.
*
*
* ruleSets "category/java/errorprone.xml", "category/java/bestpractices.xml"
*
*
* @param ruleSets the rule sets to be added
*/
public void ruleSets(String... ruleSets) {
this.ruleSets.addAll(Arrays.asList(ruleSets));
}
/**
* The target jdk to use with pmd, 1.3, 1.4, 1.5, 1.6, 1.7 or jsp
*/
public TargetJdk getTargetJdk() {
return targetJdk;
}
/**
* Sets the target jdk used with pmd.
*
* @param targetJdk The target jdk
* @since 4.0
*/
public void setTargetJdk(TargetJdk targetJdk) {
this.targetJdk = targetJdk;
}
/**
* The maximum number of failures to allow before stopping the build.
*
* If ignoreFailures
is set, this is ignored and no limit is enforced.
*
* @since 6.4
*/
public Property getMaxFailures() {
return maxFailures;
}
/**
* Sets the target jdk used with pmd.
*
* @param value The value for the target jdk as defined by {@link TargetJdk#toVersion(Object)}
*/
public void setTargetJdk(Object value) {
targetJdk = TargetJdk.toVersion(value);
}
/**
* The rule priority threshold; violations for rules with a lower priority will not be reported. Default value is 5, which means that all violations will be reported.
*
* This is equivalent to PMD's Ant task minimumPriority property.
*
* See the official documentation for the list of priorities.
*
*
* rulesMinimumPriority = 3
*
*
* @since 6.8
*/
public Property getRulesMinimumPriority() {
return rulesMinimumPriority;
}
/**
* The custom rule set to be used (if any). Replaces {@code ruleSetFiles}, except that it does not currently support multiple rule sets.
*
* See the official documentation for how to author a rule set.
*
*
* ruleSetConfig = resources.text.fromFile("config/pmd/myRuleSet.xml")
*
*
* @since 2.2
*/
@Nullable
public TextResource getRuleSetConfig() {
return ruleSetConfig;
}
/**
* The custom rule set to be used (if any). Replaces {@code ruleSetFiles}, except that it does not currently support multiple rule sets.
*
* See the official documentation for how to author a rule set.
*
*
* ruleSetConfig = resources.text.fromFile("config/pmd/myRuleSet.xml")
*
*
* @since 2.2
*/
public void setRuleSetConfig(@Nullable TextResource ruleSetConfig) {
this.ruleSetConfig = ruleSetConfig;
}
/**
* The custom rule set files to be used. See the official documentation for how to author a rule set file.
* If you want to only use custom rule sets, you must clear {@code ruleSets}.
*
*
* ruleSetFiles = files("config/pmd/myRuleSet.xml")
*
*/
public FileCollection getRuleSetFiles() {
return ruleSetFiles;
}
/**
* The custom rule set files to be used. See the official documentation for how to author a rule set file.
* This adds to the default rule sets defined by {@link #getRuleSets()}.
*
*
* ruleSetFiles = files("config/pmd/myRuleSets.xml")
*
*/
public void setRuleSetFiles(FileCollection ruleSetFiles) {
this.ruleSetFiles = project.getObjects().fileCollection().from(ruleSetFiles);
}
/**
* Convenience method for adding rule set files.
*
*
* ruleSetFiles "config/pmd/myRuleSet.xml"
*
*
* @param ruleSetFiles the rule set files to be added
*/
public void ruleSetFiles(Object... ruleSetFiles) {
this.ruleSetFiles.from(ruleSetFiles);
}
/**
* Whether or not to write PMD results to {@code System.out}.
*/
public boolean isConsoleOutput() {
return consoleOutput;
}
/**
* Whether or not to write PMD results to {@code System.out}.
*/
public void setConsoleOutput(boolean consoleOutput) {
this.consoleOutput = consoleOutput;
}
/**
* Controls whether to use incremental analysis or not.
*
* This is only supported for PMD 6.0.0 or better. See for more details.
*
* @since 5.6
*/
public Property getIncrementalAnalysis() {
return incrementalAnalysis;
}
/**
* The number of threads used by PMD.
*
* @since 7.5
*/
@Incubating
public Property getThreads() {
return threads;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy