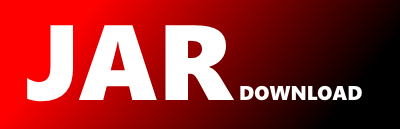
org.gradle.configurationcache.serialization.codecs.CollectionCodecs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.configurationcache.serialization.codecs
import org.gradle.configurationcache.serialization.Codec
import org.gradle.configurationcache.serialization.ReadContext
import org.gradle.configurationcache.serialization.WriteContext
import org.gradle.configurationcache.serialization.codec
import org.gradle.configurationcache.serialization.logPropertyProblem
import org.gradle.configurationcache.serialization.readCollectionInto
import org.gradle.configurationcache.serialization.readMapInto
import org.gradle.configurationcache.serialization.writeCollection
import org.gradle.configurationcache.serialization.writeMap
import java.util.LinkedList
import java.util.TreeMap
import java.util.TreeSet
import java.util.concurrent.ConcurrentHashMap
internal
val arrayListCodec: Codec> = collectionCodec { ArrayList(it) }
internal
val linkedListCodec: Codec> = collectionCodec { LinkedList() }
/**
* Decodes HashSet instances as LinkedHashSet to preserve original iteration order.
*/
internal
object HashSetCodec : Codec> {
override suspend fun WriteContext.encode(value: HashSet) {
writeCollectionCheckingForCircularElements(value)
}
override suspend fun ReadContext.decode(): HashSet =
readCollectionCheckingForCircularElementsInto(::LinkedHashSet)
}
private
suspend fun WriteContext.writeCollectionCheckingForCircularElements(collection: Collection) {
var writeCircularMarker = true
writeCollection(collection) { element ->
if (element != null && element in circularReferences && element.javaClass.overridesHashCode()) {
logPropertyProblem("serialize") {
text("Circular references can lead to undefined behavior upon deserialization.")
}
if (writeCircularMarker) {
write(CircularReferenceMarker.INSTANCE)
writeCircularMarker = false
}
}
write(element)
}
}
private
suspend fun > ReadContext.readCollectionCheckingForCircularElementsInto(
factory: (Int) -> T
): T {
val size = readSmallInt()
val container = factory(size)
for (i in 0 until size) {
val element = read()
if (element === CircularReferenceMarker.INSTANCE) {
// upon reading a circular reference, wait until all objects have been initialized
// before inserting the elements in the resulting set.
val remainingSize = size - i
val remaining = ArrayList(remainingSize).apply {
for (j in 0 until remainingSize) {
add(read())
}
}
onFinish {
container.addAll(remaining)
}
return container
}
container.add(element)
}
return container
}
private
object CircularReferenceMarker {
val INSTANCE: Any = Marker.INSTANCE
private
enum class Marker {
INSTANCE
}
}
private
fun Class<*>.overridesHashCode(): Boolean =
getMethod("hashCode").declaringClass !== java.lang.Object::class.java
internal
val treeSetCodec: Codec> = codec(
{
write(it.comparator())
writeCollection(it)
},
{
@Suppress("unchecked_cast")
val comparator = read() as Comparator?
readCollectionInto { TreeSet(comparator) }
}
)
internal
fun > collectionCodec(factory: (Int) -> T) = codec(
{ writeCollection(it) },
{ readCollectionInto(factory) }
)
/**
* Decodes HashMap instances as LinkedHashMap to preserve original iteration order.
*/
internal
val hashMapCodec: Codec> = mapCodec { LinkedHashMap(it) }
internal
val linkedHashMapCodec: Codec> = mapCodec { LinkedHashMap(it) }
internal
val concurrentHashMapCodec: Codec> = mapCodec { ConcurrentHashMap(it) }
internal
val treeMapCodec: Codec> = codec(
{
write(it.comparator())
writeMap(it)
},
{
@Suppress("unchecked_cast")
val comparator = read() as Comparator?
readMapInto { TreeMap(comparator) }
}
)
internal
fun > mapCodec(factory: (Int) -> T): Codec = codec(
{ writeMap(it) },
{ readMapInto(factory) }
)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy