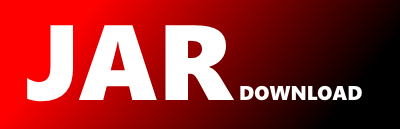
org.gradle.internal.operations.BuildOperationQueue Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.internal.operations;
/**
* An individual active, single use, queue of build operations.
*
* The queue is active in that operations are potentially executed as soon as they are added.
* The queue is single use in that no further work can be added once {@link #waitForCompletion()} has completed.
*
* A queue instance is threadsafe. Build operations can submit further operations to the queue but must not block waiting for them to complete.
*
* @param type of build operations to hold
*/
public interface BuildOperationQueue {
/**
* Adds an operation to be executed, potentially executing it instantly.
*
* @param operation operation to execute
*/
void add(T operation);
/**
* Cancels all queued operations in this queue. Any operations that have started will be allowed to complete.
*/
void cancel();
/**
* Waits for all previously added operations to complete.
*
* On failure, some effort is made to cancel any operations that have not started.
*
* @throws MultipleBuildOperationFailures if any operation failed
*/
void waitForCompletion() throws MultipleBuildOperationFailures;
/**
* Sets the location of a log file where build operation output can be found. For use in exceptions.
*/
void setLogLocation(String logLocation);
interface QueueWorker {
void execute(O buildOperation);
String getDisplayName();
}
}