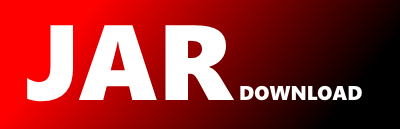
org.gradle.api.Task Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api;
import groovy.lang.Closure;
import groovy.lang.DelegatesTo;
import groovy.lang.MissingPropertyException;
import org.gradle.api.logging.Logger;
import org.gradle.api.logging.LoggingManager;
import org.gradle.api.plugins.ExtensionAware;
import org.gradle.api.provider.Property;
import org.gradle.api.provider.Provider;
import org.gradle.api.services.BuildService;
import org.gradle.api.services.BuildServiceRegistration;
import org.gradle.api.specs.Spec;
import org.gradle.api.tasks.Internal;
import org.gradle.api.tasks.TaskDependency;
import org.gradle.api.tasks.TaskDestroyables;
import org.gradle.api.tasks.TaskInputs;
import org.gradle.api.tasks.TaskLocalState;
import org.gradle.api.tasks.TaskOutputs;
import org.gradle.api.tasks.TaskState;
import org.gradle.internal.deprecation.DeprecationLogger;
import javax.annotation.Nullable;
import java.io.File;
import java.time.Duration;
import java.util.List;
import java.util.Set;
/**
* A Task
represents a single atomic piece of work for a build, such as compiling classes or generating
* javadoc.
*
* Each task belongs to a {@link Project}. You can use the various methods on {@link
* org.gradle.api.tasks.TaskContainer} to create and lookup task instances. For example, {@link
* org.gradle.api.tasks.TaskContainer#create(String)} creates an empty task with the given name. You can also use the
* {@code task} keyword in your build file:
*
* task myTask
* task myTask { configure closure }
* task myTask(type: SomeType)
* task myTask(type: SomeType) { configure closure }
*
*
* Each task has a name, which can be used to refer to the task within its owning project, and a fully qualified
* path, which is unique across all tasks in all projects. The path is the concatenation of the owning project's path
* and the task's name. Path elements are separated using the {@value org.gradle.api.Project#PATH_SEPARATOR}
* character.
*
* Task Actions
*
* A Task
is made up of a sequence of {@link Action} objects. When the task is executed, each of the
* actions is executed in turn, by calling {@link Action#execute}. You can add actions to a task by calling {@link
* #doFirst(Action)} or {@link #doLast(Action)}.
*
* Groovy closures can also be used to provide a task action. When the action is executed, the closure is called with
* the task as parameter. You can add action closures to a task by calling {@link #doFirst(groovy.lang.Closure)} or
* {@link #doLast(groovy.lang.Closure)}.
*
* There are 2 special exceptions which a task action can throw to abort execution and continue without failing the
* build. A task action can abort execution of the action and continue to the next action of the task by throwing a
* {@link org.gradle.api.tasks.StopActionException}. A task action can abort execution of the task and continue to the
* next task by throwing a {@link org.gradle.api.tasks.StopExecutionException}. Using these exceptions allows you to
* have precondition actions which skip execution of the task, or part of the task, if not true.
*
* Task Dependencies and Task Ordering
*
* A task may have dependencies on other tasks or might be scheduled to always run after another task.
* Gradle ensures that all task dependencies and ordering rules are honored when executing tasks, so that the task is executed after
* all of its dependencies and any "must run after" tasks have been executed.
*
* Dependencies to a task are controlled using {@link #dependsOn(Object...)} or {@link #setDependsOn(Iterable)},
* and {@link #mustRunAfter(Object...)}, {@link #setMustRunAfter(Iterable)}, {@link #shouldRunAfter(Object...)} and
* {@link #setShouldRunAfter(Iterable)} are used to specify ordering between tasks. You can use objects of any of
* the following types to specify dependencies and ordering:
*
*
*
* - A {@code String}, {@code CharSequence} or {@code groovy.lang.GString} task path or name. A relative path is interpreted relative to the task's {@link Project}. This
* allows you to refer to tasks in other projects. These task references will not cause task creation.
*
* - A {@link Task}.
*
* - A {@link TaskDependency} object.
*
* - A {@link org.gradle.api.tasks.TaskReference} object.
*
* - A {@link Buildable} object.
*
* - A {@link org.gradle.api.file.RegularFileProperty} or {@link org.gradle.api.file.DirectoryProperty}.
*
* - A {@link Provider} object. May contain any of the types listed here.
*
* - A {@code Iterable}, {@code Collection}, {@code Map} or array. May contain any of the types listed here. The elements of the
* iterable/collection/map/array are recursively converted to tasks.
*
* - A {@code Callable}. The {@code call()} method may return any of the types listed here. Its return value is
* recursively converted to tasks. A {@code null} return value is treated as an empty collection.
*
* - A Groovy {@code Closure} or Kotlin function. The closure may take a {@code Task} as parameter.
* The closure or function may return any of the types listed here. Its return value is
* recursively converted to tasks. A {@code null} return value is treated as an empty collection.
*
* - Anything else is treated as an error.
*
*
*
* Using a Task in a Build File
*
* Dynamic Properties
*
* A {@code Task} has 4 'scopes' for properties. You can access these properties by name from the build file or by
* calling the {@link #property(String)} method. You can change the value of these properties by calling the {@link #setProperty(String, Object)} method.
*
*
*
* - The {@code Task} object itself. This includes any property getters and setters declared by the {@code Task}
* implementation class. The properties of this scope are readable or writable based on the presence of the
* corresponding getter and setter methods.
*
* - The extensions added to the task by plugins. Each extension is available as a read-only property with the same
* name as the extension.
*
* - The convention properties added to the task by plugins. A plugin can add properties and methods to a task through
* the task's {@link org.gradle.api.plugins.Convention} object. The properties of this scope may be readable or writable, depending on the convention objects.
*
* - The extra properties of the task. Each task object maintains a map of additional properties. These
* are arbitrary name -> value pairs which you can use to dynamically add properties to a task object. Once defined, the properties
* of this scope are readable and writable.
*
*
*
* Dynamic Methods
*
* A {@link Plugin} may add methods to a {@code Task} using its {@link org.gradle.api.plugins.Convention} object.
*
* Parallel Execution
*
* By default, tasks are not executed in parallel unless a task is waiting on asynchronous work and another task (which
* is not dependent) is ready to execute.
*
* Parallel execution can be enabled by the --parallel
flag when the build is initiated.
* In parallel mode, the tasks of different projects (i.e. in a multi project build) are able to be executed in parallel.
*/
public interface Task extends Comparable, ExtensionAware, Named {
String TASK_NAME = "name";
String TASK_DESCRIPTION = "description";
String TASK_GROUP = "group";
String TASK_TYPE = "type";
String TASK_DEPENDS_ON = "dependsOn";
String TASK_OVERWRITE = "overwrite";
String TASK_ACTION = "action";
/**
* Constructor arguments for the Task
*
* @since 4.7
*/
String TASK_CONSTRUCTOR_ARGS = "constructorArgs";
/**
* Returns the name of this task. The name uniquely identifies the task within its {@link Project}.
*
* @return The name of the task. Never returns null.
*/
@Internal
@Override
String getName();
/**
* An implementation of the namer interface for tasks that returns {@link #getName()}.
*
* @deprecated Use {@link Named.Namer#INSTANCE} instead (since {@link Task} now extends {@link Named}).
*/
@Deprecated
class Namer implements org.gradle.api.Namer {
public Namer() {
DeprecationLogger.deprecateType(Namer.class)
.replaceWith("Named.Namer.INSTANCE")
.withContext("Task implements Named, so you can use Named.Namer.INSTANCE instead of Task.Namer")
.willBeRemovedInGradle9()
.withUpgradeGuideSection(8, "deprecated_namers")
.nagUser();
}
@Override
public String determineName(Task task) {
return Named.Namer.INSTANCE.determineName(task);
}
}
/**
* Returns the {@link Project} which this task belongs to.
*
* Calling this method from a task action is not supported when configuration caching is enabled.
*
* @return The project this task belongs to. Never returns null.
*/
@Internal
Project getProject();
/**
* Returns the sequence of {@link Action} objects which will be executed by this task, in the order of
* execution.
*
* @return The task actions in the order they are executed. Returns an empty list if this task has no actions.
*/
@Internal
List> getActions();
/**
* Sets the sequence of {@link Action} objects which will be executed by this task.
*
* @param actions The actions.
*/
void setActions(List> actions);
/**
* Returns a {@link TaskDependency} which contains all the tasks that this task depends on.
*
* Calling this method from a task action is not supported when configuration caching is enabled.
*
* @return The dependencies of this task. Never returns null.
*/
@Internal
TaskDependency getTaskDependencies();
/**
* Returns the dependencies of this task.
*
* @return The dependencies of this task. Returns an empty set if this task has no dependencies.
*/
@Internal
Set