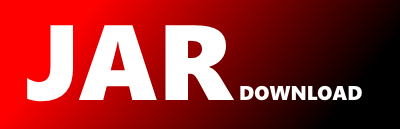
org.gradle.api.execution.TaskExecutionGraph Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2007-2008 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.execution;
import groovy.lang.Closure;
import org.gradle.api.Action;
import org.gradle.api.Task;
import java.util.List;
import java.util.Set;
/**
* A TaskExecutionGraph
is responsible for managing the execution of the {@link Task} instances which
* are part of the build. The TaskExecutionGraph
maintains an execution plan of tasks to be executed (or
* which have been executed), and you can query this plan from your build file.
*
* You can access the {@code TaskExecutionGraph} by calling {@link org.gradle.api.invocation.Gradle#getTaskGraph()}.
* In your build file you can use {@code gradle.taskGraph} to access it.
*
* The TaskExecutionGraph
is populated only after all the projects in the build have been evaluated. It
* is empty before then. You can receive a notification when the graph is populated, using {@link
* #whenReady(groovy.lang.Closure)} or {@link #addTaskExecutionGraphListener(TaskExecutionGraphListener)}.
*/
public interface TaskExecutionGraph {
/**
* Adds a listener to this graph, to be notified when this graph is ready.
*
* @param listener The listener to add. Does nothing if this listener has already been added.
*/
void addTaskExecutionGraphListener(TaskExecutionGraphListener listener);
/**
* Remove a listener from this graph.
*
* @param listener The listener to remove. Does nothing if this listener was never added to this graph.
*/
void removeTaskExecutionGraphListener(TaskExecutionGraphListener listener);
/**
* Adds a listener to this graph, to be notified as tasks are executed.
*
* @param listener The listener to add. Does nothing if this listener has already been added.
* @deprecated This method is not supported when configuration caching is enabled.
*/
@Deprecated
void addTaskExecutionListener(TaskExecutionListener listener);
/**
* Remove a listener from this graph.
*
* @param listener The listener to remove. Does nothing if this listener was never added to this graph.
* @deprecated This method is not supported when configuration caching is enabled.
*/
@Deprecated
void removeTaskExecutionListener(TaskExecutionListener listener);
/**
* Adds a closure to be called when this graph has been populated. This graph is passed to the closure as a
* parameter.
*
* @param closure The closure to execute when this graph has been populated.
*/
void whenReady(Closure closure);
/**
* Adds an action to be called when this graph has been populated. This graph is passed to the action as a
* parameter.
*
* @param action The action to execute when this graph has been populated.
*
* @since 3.1
*/
void whenReady(Action action);
/**
* Adds a closure to be called immediately before a task is executed. The task is passed to the closure as a
* parameter.
*
* @param closure The closure to execute when a task is about to be executed.
* @deprecated This method is not supported when configuration caching is enabled.
*/
@Deprecated
void beforeTask(Closure closure);
/**
* Adds an action to be called immediately before a task is executed. The task is passed to the action as a
* parameter.
*
* @param action The action to execute when a task is about to be executed.
* @deprecated This method is not supported when configuration caching is enabled.
*
* @since 3.1
*/
@Deprecated
void beforeTask(Action action);
/**
* Adds a closure to be called immediately after a task has executed. The task is passed to the closure as the
* first parameter. A {@link org.gradle.api.tasks.TaskState} is passed as the second parameter. Both parameters are
* optional.
*
* @param closure The closure to execute when a task has been executed
* @deprecated This method is not supported when configuration caching is enabled.
*/
@Deprecated
void afterTask(Closure closure);
/**
* Adds an action to be called immediately after a task has executed. The task is passed to the action as the
* first parameter.
*
* @param action The action to execute when a task has been executed
* @deprecated This method is not supported when configuration caching is enabled.
*
* @since 3.1
*/
@Deprecated
void afterTask(Action action);
/**
* Determines whether the given task is included in the execution plan.
*
* @param path the absolute path of the task.
* @return true if a task with the given path is included in the execution plan.
* @throws IllegalStateException When this graph has not been populated.
*/
boolean hasTask(String path);
/**
* Determines whether the given task is included in the execution plan.
*
* @param task the task
* @return true if the given task is included in the execution plan.
* @throws IllegalStateException When this graph has not been populated.
*/
boolean hasTask(Task task);
/**
* Returns the tasks which are included in the execution plan.
* The order of the tasks in the result is compatible with the constraints (dependsOn/mustRunAfter/etc) set in the build configuration.
* However, Gradle may execute tasks in a slightly different order to speed up the overall execution while still respecting the constraints.
*
*
* @return The tasks. Returns an empty list if no tasks are to be executed.
*/
List getAllTasks();
/**
* Returns the dependencies of a task which are part of the execution graph.
*
* @return The tasks. Returns an empty set if there are no dependent tasks.
* @throws IllegalStateException When this graph has not been populated or the task is not part of it.
*
* @since 4.6
*/
Set getDependencies(Task task);
}