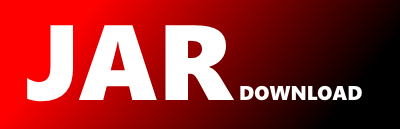
org.gradle.api.internal.tasks.properties.DefaultTaskProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.internal.tasks.properties;
import com.google.common.collect.ImmutableSortedSet;
import org.gradle.api.NonNullApi;
import org.gradle.api.file.FileCollection;
import org.gradle.api.internal.TaskInternal;
import org.gradle.api.internal.file.FileCollectionFactory;
import org.gradle.api.internal.tasks.TaskDependencyContainer;
import org.gradle.api.internal.tasks.TaskPropertyUtils;
import org.gradle.api.services.BuildService;
import org.gradle.api.tasks.TaskExecutionException;
import org.gradle.internal.fingerprint.DirectorySensitivity;
import org.gradle.internal.fingerprint.FileNormalizer;
import org.gradle.internal.fingerprint.LineEndingSensitivity;
import org.gradle.internal.properties.InputBehavior;
import org.gradle.internal.properties.InputFilePropertyType;
import org.gradle.internal.properties.PropertyValue;
import org.gradle.internal.properties.PropertyVisitor;
import org.gradle.internal.properties.bean.PropertyWalker;
import org.gradle.internal.reflect.validation.ReplayingTypeValidationContext;
import org.gradle.internal.reflect.validation.TypeValidationContext;
import javax.annotation.Nullable;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
@NonNullApi
public class DefaultTaskProperties implements TaskProperties {
private final ImmutableSortedSet inputProperties;
private final ImmutableSortedSet inputFileProperties;
private final ImmutableSortedSet outputFileProperties;
private final ImmutableSortedSet serviceReferences;
private final boolean hasDeclaredOutputs;
private final ReplayingTypeValidationContext validationProblems;
private final FileCollection localStateFiles;
private final FileCollection destroyableFiles;
private final List validatingProperties;
public static TaskProperties resolve(PropertyWalker propertyWalker, FileCollectionFactory fileCollectionFactory, TaskInternal task) {
String beanName = task.toString();
GetInputPropertiesVisitor inputPropertiesVisitor = new GetInputPropertiesVisitor();
GetInputFilesVisitor inputFilesVisitor = new GetInputFilesVisitor(beanName, fileCollectionFactory);
GetServiceReferencesVisitor serviceReferencesVisitor = new GetServiceReferencesVisitor();
ValidationVisitor validationVisitor = new ValidationVisitor();
OutputFilesCollector outputFilesCollector = new OutputFilesCollector();
OutputUnpacker outputUnpacker = new OutputUnpacker(
beanName,
fileCollectionFactory,
true,
true,
OutputUnpacker.UnpackedOutputConsumer.composite(outputFilesCollector, validationVisitor)
);
GetLocalStateVisitor localStateVisitor = new GetLocalStateVisitor(beanName, fileCollectionFactory);
GetDestroyablesVisitor destroyablesVisitor = new GetDestroyablesVisitor(beanName, fileCollectionFactory);
ReplayingTypeValidationContext validationContext = new ReplayingTypeValidationContext();
try {
TaskPropertyUtils.visitProperties(propertyWalker, task, validationContext, new CompositePropertyVisitor(
inputPropertiesVisitor,
inputFilesVisitor,
outputUnpacker,
validationVisitor,
destroyablesVisitor,
localStateVisitor,
serviceReferencesVisitor
));
} catch (Exception e) {
throw new TaskExecutionException(task, e);
}
return new DefaultTaskProperties(
inputPropertiesVisitor.getProperties(),
inputFilesVisitor.getFileProperties(),
outputFilesCollector.getFileProperties(),
serviceReferencesVisitor.getServiceReferences(),
outputUnpacker.hasDeclaredOutputs(),
localStateVisitor.getFiles(),
destroyablesVisitor.getFiles(),
validationVisitor.getTaskPropertySpecs(),
validationContext);
}
private DefaultTaskProperties(
ImmutableSortedSet inputProperties,
ImmutableSortedSet inputFileProperties,
ImmutableSortedSet outputFileProperties,
ImmutableSortedSet serviceReferences,
boolean hasDeclaredOutputs,
FileCollection localStateFiles,
FileCollection destroyableFiles,
List validatingProperties,
ReplayingTypeValidationContext validationProblems
) {
this.validatingProperties = validatingProperties;
this.validationProblems = validationProblems;
this.inputProperties = inputProperties;
this.inputFileProperties = inputFileProperties;
this.outputFileProperties = outputFileProperties;
this.serviceReferences = serviceReferences;
this.hasDeclaredOutputs = hasDeclaredOutputs;
this.localStateFiles = localStateFiles;
this.destroyableFiles = destroyableFiles;
}
@Override
public Iterable extends LifecycleAwareValue> getLifecycleAwareValues() {
return validatingProperties;
}
@Override
public ImmutableSortedSet getOutputFileProperties() {
return outputFileProperties;
}
@Override
public ImmutableSortedSet getInputFileProperties() {
return inputFileProperties;
}
@Override
public ImmutableSortedSet getServiceReferences() {
return serviceReferences;
}
@Override
public void validateType(TypeValidationContext validationContext) {
validationProblems.replay(null, validationContext);
}
@Override
public void validate(PropertyValidationContext validationContext) {
for (ValidatingProperty validatingProperty : validatingProperties) {
validatingProperty.validate(validationContext);
}
}
@Override
public boolean hasDeclaredOutputs() {
return hasDeclaredOutputs;
}
@Override
public ImmutableSortedSet getInputProperties() {
return inputProperties;
}
@Override
public FileCollection getLocalStateFiles() {
return localStateFiles;
}
@Override
public FileCollection getDestroyableFiles() {
return destroyableFiles;
}
private static class GetLocalStateVisitor implements PropertyVisitor {
private final String beanName;
private final FileCollectionFactory fileCollectionFactory;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy