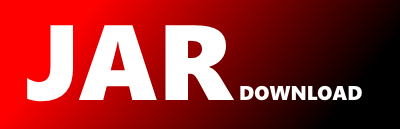
org.gradle.api.plugins.ExtensionAware Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.plugins;
import org.gradle.api.tasks.Internal;
/**
* Objects that can be extended at runtime with other objects.
*
*
* // Extensions are just plain objects, there is no interface/type
* class MyExtension {
* String foo
*
* MyExtension(String foo) {
* this.foo = foo
* }
* }
*
* // Add new extensions via the extension container
* project.extensions.create('custom', MyExtension, "bar")
* // («name», «type», «constructor args», …)
*
* // extensions appear as properties on the target object by the given name
* assert project.custom instanceof MyExtension
* assert project.custom.foo == "bar"
*
* // also via a namespace method
* project.custom {
* assert foo == "bar"
* foo = "other"
* }
* assert project.custom.foo == "other"
*
* // Extensions added with the extension container's create method are themselves extensible
* assert project.custom instanceof ExtensionAware
* project.custom.extensions.create("nested", MyExtension, "baz")
* assert project.custom.nested.foo == "baz"
*
* // All extension aware objects have a special “ext” extension of type ExtraPropertiesExtension
* assert project.hasProperty("myProperty") == false
* project.ext.myProperty = "myValue"
*
* // Properties added to the “ext” extension are promoted to the owning object
* assert project.myProperty == "myValue"
*
*
* Many Gradle objects are extension aware. This includes; projects, tasks, configurations, dependencies etc.
*
* For more on adding & creating extensions, see {@link ExtensionContainer}.
*
* For more on extra properties, see {@link ExtraPropertiesExtension}.
*
* An ExtensionAware
object has several 'scopes' that Gradle searches for properties. These scopes are:
*
*
* - The object itself. This scope includes any property getters and setters declared by the
* implementation class. The properties of this scope are readable or writable depending on the presence
* of the corresponding getter or setter method.
*
* - Groovy Meta-programming methods implemented by the object's class, like
propertyMissing()
. Care must be taken by plugin authors to
* ensure propertyMissing()
is implemented such that if a property is not found a MissingPropertyException(String, Class) exception is thrown.
* If propertyMissing()
always returns a value for any property, Gradle will not search the rest of the scopes below.
*
* - The extra properties of the object. Each object maintains a map of extra properties, which
* can contain any arbitrary name -> value pair. Once defined, the properties of this scope are readable and writable.
*
* - The extensions added to the object by plugins. Each extension is available as a read-only property with the same name as the extension.
*
*/
public interface ExtensionAware {
/**
* The container of extensions.
*/
@Internal
ExtensionContainer getExtensions();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy