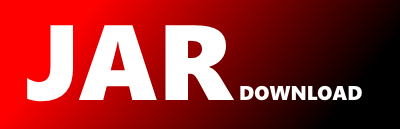
org.gradle.api.reporting.dependencies.internal.JsonProjectDependencyRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.reporting.dependencies.internal;
import groovy.json.JsonBuilder;
import org.gradle.api.artifacts.ModuleIdentifier;
import org.gradle.api.artifacts.component.ComponentIdentifier;
import org.gradle.api.artifacts.component.ModuleComponentIdentifier;
import org.gradle.api.artifacts.result.DependencyResult;
import org.gradle.api.artifacts.result.ResolvedComponentResult;
import org.gradle.api.internal.artifacts.ivyservice.ivyresolve.strategy.VersionComparator;
import org.gradle.api.internal.artifacts.ivyservice.ivyresolve.strategy.VersionParser;
import org.gradle.api.internal.artifacts.ivyservice.ivyresolve.strategy.VersionSelectorScheme;
import org.gradle.api.internal.artifacts.result.DefaultResolvedComponentResult;
import org.gradle.api.specs.Spec;
import org.gradle.api.tasks.diagnostics.internal.ConfigurationDetails;
import org.gradle.api.tasks.diagnostics.internal.ProjectDetails.ProjectNameAndPath;
import org.gradle.api.tasks.diagnostics.internal.graph.nodes.RenderableDependency;
import org.gradle.api.tasks.diagnostics.internal.graph.nodes.RenderableModuleResult;
import org.gradle.api.tasks.diagnostics.internal.insight.DependencyInsightReporter;
import org.gradle.internal.Actions;
import org.gradle.util.GradleVersion;
import org.gradle.util.internal.CollectionUtils;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Renderer that emits a JSON tree containing the HTML dependency report structure for a given project. The structure is the following:
*
*
* {
* "gradleVersion" : "...",
* "generationDate" : "...",
* "project" : {
* "name" : "...",
* "description : "...", (optional)
* "configurations" : [
* "name" : "...",
* "description" : "...", (optional)
* "dependencies" : [
* {
* "module" : "group:name"
* "name" : "...",
* "resolvable" : "FAILED" | "RESOLVED" | "RESOLVED_CONSTRAINT" | "UNRESOLVED",
* "alreadyRendered" : true|false
* "hasConflict" : true|false
* "children" : [
* same array as configurations.dependencies.children
* ]
* },
* ...
* ],
* "moduleInsights : [
* {
* "module" : "group:name"
* "insight" : [
* {
* "name" : "...",
* "description" : "...",
* "resolvable" : "FAILED" | "RESOLVED" | "RESOLVED_CONSTRAINT" | "UNRESOLVED",
* "hasConflict" : true|false,
* "children": [
* {
* "name" : "...",
* "resolvable" : "...",
* "hasConflict" : true|false,
* "alreadyRendered" : true|false
* "isLeaf" : true|false
* "children" : [
* same array as configurations.moduleInsights.insight.children
* ]
* },
* ...
* ]
* },
* ...
* ]
* }
* ,
* ...
* ]
* ]
* }
* }
*
*/
class JsonProjectDependencyRenderer {
public JsonProjectDependencyRenderer(VersionSelectorScheme versionSelectorScheme, VersionComparator versionComparator, VersionParser versionParser) {
this.versionSelectorScheme = versionSelectorScheme;
this.versionComparator = versionComparator;
this.versionParser = versionParser;
}
/**
* Generates the project dependency report structure
*
* @param project the project for which the report must be generated
* @return the generated JSON, as a String
*/
public String render(ProjectNameAndPath project, Iterable configurations) {
JsonBuilder json = new JsonBuilder();
renderProject(project, configurations, json);
return json.toString();
}
// Historic note: this class still uses the Groovy JsonBuilder, as it was originally developed as a Groovy class.
private void renderProject(ProjectNameAndPath project, Iterable configurations, JsonBuilder json) {
Map overall = new LinkedHashMap<>();
overall.put("gradleVersion", GradleVersion.current().toString());
overall.put("generationDate", new Date().toString());
Map projectOut = new LinkedHashMap<>();
projectOut.put("name", project.getName());
projectOut.put("description", project.getDescription());
projectOut.put("configurations", createConfigurations(configurations));
overall.put("project", projectOut);
json.call(overall);
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy