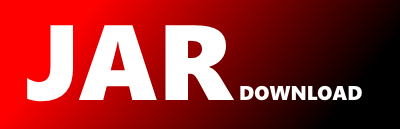
org.gradle.api.file.ConfigurableFilePermissions Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.api.file;
import org.gradle.api.Action;
/**
* Provides the means of specifying file and directory access permissions for all classes of system users.
*
* For details on classes of users and file/directory permissions see {@link FilePermissions}.
*
* An example usage of this functionality would be configuring a copy task and explicitly specifying the destination file permissions:
*
* from(...)
* into(...)
* filePermissions {
* user {
* read = true
* execute = true
* }
* other.execute = false
* }
*
* @since 8.3
*/
public interface ConfigurableFilePermissions extends FilePermissions {
/**
* Returns the permissions the owner of the file has for the file/directory.
*
* The returned object is live, modifying it will change the user's permissions.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*/
@Override
ConfigurableUserClassFilePermissions getUser();
/**
* Modifies the permissions the owner of the file has for the file/directory.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*
* Note that the provided configuration action only applies incremental modifications on top of whatever permission
* the user has at the moment and that the default values permissions start out are different for files and directories
* (see {@link UserClassFilePermissions}).
*/
void user(Action super ConfigurableUserClassFilePermissions> configureAction);
/**
* Returns the permissions a user, who is a member of the group that the file/directory belongs to, has for the file/directory.
*
* The returned object is live, modifying it will change the user's permissions.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*/
@Override
ConfigurableUserClassFilePermissions getGroup();
/**
* Modifies the permissions a user, who is a member of the group that the file/directory belongs to, has for the file/directory.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*
* Note that the provided configuration action only applies incremental modifications on top of whatever permission
* the user has at the moment and that the default values permissions start out are different for files and directories
* (see {@link UserClassFilePermissions}).
*/
void group(Action super ConfigurableUserClassFilePermissions> configureAction);
/**
* Returns the permissions all other users (non-owner, non-group) have for the file/directory.
*
* The returned object is live, modifying it will change the user's permissions.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*/
@Override
ConfigurableUserClassFilePermissions getOther();
/**
* Modifies the permissions all other users (non-owner, non-group) have
* for the file/directory.
*
* For further details on permissions see {@link ConfigurableUserClassFilePermissions}.
*
* Note that the provided configuration action only applies incremental modifications on top of whatever permission
* the user has at the moment and that the default values permissions start out are different for files and directories
* (see {@link UserClassFilePermissions}).
*/
void other(Action super ConfigurableUserClassFilePermissions> configureAction);
/**
* Sets Unix style permissions. Accept values in two styles of notation:
*
* - NUMERIC notation: uses 3 octal (base-8) digits representing permissions for the 3 categories of users; for example "755"
* - SYMBOLIC notation: uses 3 sets of 3 characters, each set representing the permissions for one of the user categories; for example "rwxr-xr-x"
*
*
* The NUMERIC notation consist of 3 digits having values from 0 to 7.
* 1st digit represents the OWNER, 2nd represents the GROUP while the 3rd represents OTHER users.
*
* Each of the digits is the sum of its component bits in the binary numeral system.
* Each of the 3 bits represents a permission.
* 1st bit is the READ bit, adds 4 to the digit (binary 100).
* 2nd bit is the WRITE bit, adds 2 to the digit (binary 010).
* 3rd bit is the EXECUTE bit, adds 1 to the digit (binary 001).
*
* See the examples below.
*
* NOTE: providing the 3 numeric digits can also be done in the octal literal form, so "0740" will
* be handled identically to "740".
*
* The SYMBOLIC notation consists of 3 sets of 3 characters. The 1st set represents the OWNER,
* the 2nd set represents the GROUP, the 3rd set represents OTHER users.
*
* Each of the tree characters represents the read, write and execute permissions:
*
* r
if READING is permitted, -
if it is not; must be 1st in the set
* w
if WRITING is permitted, -
if it is not; must be 2nd in the set
* x
if EXECUTING is permitted, -
if it is not; must be 3rd in the set
*
*
*
* Examples of Unix style permissions
*
* Numeric
* Symbolic
* Meaning
*
*
* 000
* ---------
* no permissions
*
*
* 700
* rwx------
* read, write & execute only for owner
*
*
* 770
* rwxrwx---
* read, write & execute for owner and group
*
*
* 111
* --x--x--x
* execute
*
*
* 222
* -w--w--w-
* write
*
*
* 333
* -wx-wx-wx
* write & execute
*
*
* 444
* r--r--r--
* read
*
*
* 555
* r-xr-xr-x
* read & execute
*
*
* 666
* rw-rw-rw-
* read & write
*
*
* 740
* rwxr-----
* owner can read, write & execute; group can only read; others have no permissions
*
*
*
* An example usage of this method would be configuring a copy task and explicitly specifying the destination file permissions:
*
* from(...)
* into(...)
* filePermissions { unix("r--r--r--") }
*
*/
void unix(String unixNumericOrSymbolic);
/**
* Sets Unix style numeric permissions. See {@link #unix(String)} for details.
*
* As described there, the value is expected to come from the range of 3 digit octal numbers,
* i.e. from 0 (included) to 512 (excluded).
*
* When represented in octal form, the numbers have the same meaning as their string
* counterparts (see {@link #unix(String)}), so the following statements are all equivalent:
*
*
* unix("0740") // octal number as string
* unix("740") // octal number as string (no prefix)
* unix(0740) // octal int literal
* unix(482) // decimal int literal
* unix("rwxr-----") // non-numeric string
*
*/
void unix(int unixNumeric);
}