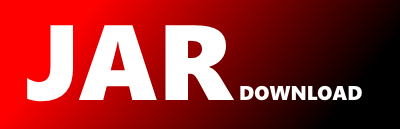
org.gradle.plugin.use.PluginDependenciesSpec Maven / Gradle / Ivy
Show all versions of gradle-api Show documentation
/*
* Copyright 2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.plugin.use;
import org.gradle.api.provider.Provider;
import org.gradle.api.provider.ProviderConvertible;
/**
* The DSL for declaring plugins to use in a script.
*
* In a build script, the plugins {}
script block API is of this type.
* That is, you can use this API in the body of the plugins script block to declare plugins to be used for the script.
*
* Relationship with the apply() method
*
* The plugins {}
block serves a similar purpose to the {@link org.gradle.api.plugins.PluginAware#apply(java.util.Map)} method
* that can be used to apply a plugin directly to a {@code Project} object or similar.
* A key difference is that plugins applied via the plugins {}
block are conceptually applied to the script, and by extension the script target.
* At this time there is no observable practical difference between the two approaches with regard to the end result.
*
* Strict Syntax
*
* When used in a build script, the plugins {}
block only allows a strict subset of the full build script programming language.
* Only the API of this type can be used, and values must be literal (e.g. constant strings, not variables).
* Interpolated strings are permitted for {@link PluginDependencySpec#version(String)}, however replacement values must be sourced from Gradle properties.
* Moreover, the plugins {}
block must be the first code of a build script.
* There is one exception to this, in that the {@code buildscript {}} block (used for declaring script dependencies) must precede it.
*
*
* This implies the following constraints:
*
*
* - Only {@link #id(String)}, {@link #alias(Provider)}, and {@link #alias(ProviderConvertible)} method calls may be top level statements
* - {@link #id(String)} calls may only be followed by a {@link PluginDependencySpec#version(String)} and/or {@link PluginDependencySpec#apply(boolean)} method call on the returned object
* - {@link #id(String)}, {@link PluginDependencySpec#version(String)} and {@link PluginDependencySpec#apply(boolean)} methods must be called with a literal argument (i.e. not a variable)
* - The
plugins {}
script block must follow any buildscript {}
script block, but must precede all other logic in the script
*
* Available Plugins
* Core Plugins
*
* Core Gradle plugins are able to be applied using the plugins {}
block.
* Core plugins must be specified without a version number, and can have a qualified or unqualified id.
* That is, the {@code java} plugin can be used via:
*
*
* plugins {
* id 'java'
* }
*
*
* Or via:
*
*
* plugins {
* id 'org.gradle.java'
* }
*
*
* Core Gradle plugins use the {@code org.gradle} namespace.
*
*
* For the list of available core plugins for a particular Gradle version, please consult the user manual.
*
* Community Plugins
*
* Non-core plugins are available from the Gradle Plugin Portal.
* These plugins are contributed by users of Gradle to extend Gradle's functionality.
* Visit plugins.gradle.org to browse the available plugins and versions.
*
*
* To use a community plugin, the fully qualified id must be specified along with a version.
*
* Settings Script Usage
*
* When used in a settings script, this API sets the default version of a plugin, allowing build scripts to
* reference a plugin id without an associated version.
*
*
* Within a settings script, the "Strict Syntax" rules outlined above do not apply. The `plugins` block may contain
* arbitrary code, and version Strings may contain property replacements. It is an error to call the `apply` method
* with a value other than `false` (the default).
*
*/
public interface PluginDependenciesSpec {
/**
* Add a dependency on the plugin with the given id.
*
*
* plugins {
* id "org.company.myplugin"
* }
*
* Further constraints (e.g. version number) can be specified by the methods of the return value.
*
* plugins {
* id "org.company.myplugin" version "1.3"
* }
*
*
* Plugins are automatically applied to the current script by default. This can be disabled using the {@code apply false} option:
*
*
* plugins {
* id "org.company.myplugin" version "1.3" apply false
* }
*
*
* This is useful to reuse task classes from a plugin or to apply it to some other target than the current script.
*
* @param id the id of the plugin to depend on
* @return a mutable plugin dependency specification that can be used to further refine the dependency
*/
PluginDependencySpec id(String id);
/**
* Adds a plugin dependency using a notation coming from a version catalog.
* The resulting dependency spec can be refined with a version overriding
* what the version catalog provides.
* @param notation the plugin reference
* @return a mutable plugin dependency specification that can be used to further refine the dependency
*
* @since 7.2
*/
default PluginDependencySpec alias(Provider notation) {
PluginDependency pluginDependency = notation.get();
if (pluginDependency.getVersion().getRequiredVersion().isEmpty()) {
return id(pluginDependency.getPluginId());
} else {
return id(pluginDependency.getPluginId()).version(pluginDependency.getVersion().getRequiredVersion());
}
}
/**
* Adds a plugin dependency using a notation coming from a version catalog.
* The resulting dependency spec can be refined with a version overriding
* what the version catalog provides.
* @param notation the plugin reference
* @return a mutable plugin dependency specification that can be used to further refine the dependency
*
* @since 7.3
*/
default PluginDependencySpec alias(ProviderConvertible notation) {
return alias(notation.asProvider());
}
}