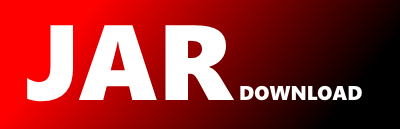
org.gradle.compile.daemon.ParallelCompilerDaemonIntegrationTest.shared.JavaClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
import java.io.File;
import java.io.Serializable;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
/**
* An immutable classpath.
*/
public class JavaClass implements Serializable {
private final List files;
public JavaClass(Iterable files) {
this.files = new ArrayList();
for (File file : files) {
this.files.add(file);
}
}
public JavaClass(File... files) {
this(Arrays.asList(files));
}
@Override
public String toString() {
return files.toString();
}
public boolean isEmpty() {
return files.isEmpty();
}
public Collection getAsURIs() {
List urls = new ArrayList();
for (File file : files) {
urls.add(file.toURI());
}
return urls;
}
public Collection getAsFiles() {
return files;
}
public URL[] getAsURLArray() {
Collection result = getAsURLs();
return result.toArray(new URL[0]);
}
public Collection getAsURLs() {
List urls = new ArrayList();
for (File file : files) {
try {
urls.add(file.toURI().toURL());
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
return urls;
}
public JavaClass plus(JavaClass other) {
if (files.isEmpty()) {
return other;
}
if (other.isEmpty()) {
return this;
}
return new JavaClass(concat(files, other.getAsFiles()));
}
public JavaClass plus(Collection other) {
if (other.isEmpty()) {
return this;
}
return new JavaClass(concat(files, other));
}
private Iterable concat(List files1, Collection files2) {
List result = new ArrayList();
result.addAll(files1);
result.addAll(files2);
return result;
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj == null || obj.getClass() != getClass()) {
return false;
}
JavaClass other = (JavaClass) obj;
return files.equals(other.files);
}
@Override
public int hashCode() {
return files.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy