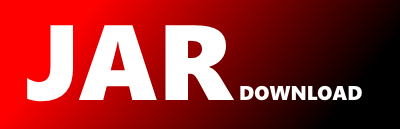
org.gradle.integtests.fixtures.configurationcache.ConfigurationCacheProblemsFixture.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.integtests.fixtures.configurationcache
import groovy.json.JsonSlurper
import groovy.transform.PackageScope
import junit.framework.AssertionFailedError
import org.gradle.api.Action
import org.gradle.api.internal.DocumentationRegistry
import org.gradle.integtests.fixtures.executer.ExecutionFailure
import org.gradle.integtests.fixtures.executer.ExecutionResult
import org.gradle.integtests.fixtures.executer.GradleExecuter
import org.gradle.integtests.fixtures.executer.LogContent
import org.gradle.internal.logging.ConsoleRenderer
import org.gradle.test.fixtures.file.TestFile
import org.gradle.util.internal.ConfigureUtil
import org.hamcrest.BaseMatcher
import org.hamcrest.Description
import org.hamcrest.Matcher
import javax.annotation.Nullable
import java.nio.file.Paths
import java.util.regex.Pattern
import static org.hamcrest.CoreMatchers.containsString
import static org.hamcrest.CoreMatchers.equalTo
import static org.hamcrest.CoreMatchers.not
import static org.hamcrest.CoreMatchers.notNullValue
import static org.hamcrest.CoreMatchers.nullValue
import static org.hamcrest.CoreMatchers.startsWith
import static org.hamcrest.MatcherAssert.assertThat
import static org.junit.Assert.assertTrue
final class ConfigurationCacheProblemsFixture {
protected static final String PROBLEMS_REPORT_HTML_FILE_NAME = "configuration-cache-report.html"
private final GradleExecuter executer
private final File rootDir
ConfigurationCacheProblemsFixture(GradleExecuter executer, File rootDir) {
this.executer = executer
this.rootDir = rootDir
}
void assertFailureHasError(
ExecutionFailure failure,
String error,
@DelegatesTo(value = HasConfigurationCacheErrorSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
assertFailureHasError(failure, error, ConfigureUtil.configureUsing(specClosure))
}
void assertFailureHasError(
ExecutionFailure failure,
String error,
Action specAction = {}
) {
assertFailureHasError(failure, newErrorSpec(error, specAction))
}
void assertFailureHasError(
ExecutionFailure failure,
HasConfigurationCacheErrorSpec spec
) {
spec.validateSpec()
assertFailureDescription(failure, failureDescriptionMatcherForError(spec))
if (spec.hasProblems()) {
assertHasConsoleSummary(failure.output, spec)
assertProblemsHtmlReport(failure.output, rootDir, spec)
} else {
assertNoProblemsSummary(failure.output)
}
}
HasConfigurationCacheErrorSpec newErrorSpec(
String error,
@DelegatesTo(value = HasConfigurationCacheErrorSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
return newErrorSpec(error, ConfigureUtil.configureUsing(specClosure))
}
HasConfigurationCacheErrorSpec newErrorSpec(
String error,
Action specAction = {}
) {
def spec = new HasConfigurationCacheErrorSpec(error)
specAction.execute(spec)
return spec
}
void assertFailureHasProblems(
ExecutionFailure failure,
@DelegatesTo(value = HasConfigurationCacheProblemsSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
assertFailureHasProblems(failure, ConfigureUtil.configureUsing(specClosure))
}
void assertFailureHasProblems(
ExecutionFailure failure,
Action specAction = {}
) {
assertFailureHasProblems(failure, newProblemsSpec(specAction))
}
void assertFailureHasProblems(
ExecutionFailure failure,
HasConfigurationCacheProblemsSpec spec
) {
assertNoProblemsSummary(failure.output)
assertFailureDescription(failure, failureDescriptionMatcherForProblems(spec))
assertProblemsHtmlReport(failure.error, rootDir, spec)
}
void assertFailureHasTooManyProblems(
ExecutionFailure failure,
@DelegatesTo(value = HasConfigurationCacheProblemsSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
assertFailureHasTooManyProblems(failure, ConfigureUtil.configureUsing(specClosure))
}
void assertFailureHasTooManyProblems(
ExecutionFailure failure,
Action specAction = {}
) {
assertFailureHasTooManyProblems(failure, newProblemsSpec(specAction))
}
void assertFailureHasTooManyProblems(
ExecutionFailure failure,
HasConfigurationCacheProblemsSpec spec
) {
assertNoProblemsSummary(failure.output)
assertFailureDescription(failure, failureDescriptionMatcherForTooManyProblems(spec))
assertProblemsHtmlReport(failure.error, rootDir, spec)
}
void assertResultHasProblems(
ExecutionResult result,
@DelegatesTo(value = HasConfigurationCacheProblemsSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
assertResultHasProblems(result, ConfigureUtil.configureUsing(specClosure))
}
void assertResultHasProblems(
ExecutionResult result,
Action specAction = {}
) {
assertResultHasProblems(result, newProblemsSpec(specAction))
}
void assertResultHasProblems(
ExecutionResult result,
HasConfigurationCacheProblemsSpec spec
) {
// assert !(result instanceof ExecutionFailure)
if (spec.hasProblems()) {
assertHasConsoleSummary(result.output, spec)
assertProblemsHtmlReport(result.output, rootDir, spec)
} else {
assertNoProblemsSummary(result.output)
}
// TODO:bamboo avoid reading jsModel twice when asserting on problems AND inputs
assertInputs(result.output, rootDir, spec.inputs)
}
HasConfigurationCacheProblemsSpec newProblemsSpec(
@DelegatesTo(value = HasConfigurationCacheProblemsSpec, strategy = Closure.DELEGATE_FIRST) Closure> specClosure
) {
return newProblemsSpec(ConfigureUtil.configureUsing(specClosure))
}
HasConfigurationCacheProblemsSpec newProblemsSpec(
Action specAction = {}
) {
def spec = new HasConfigurationCacheProblemsSpec()
specAction.execute(spec)
return spec
}
private static Matcher failureDescriptionMatcherForError(HasConfigurationCacheErrorSpec spec) {
return equalTo("Configuration cache state could not be cached: ${spec.error}".toString())
}
private static Matcher failureDescriptionMatcherForProblems(HasConfigurationCacheProblemsSpec spec) {
return buildMatcherForProblemsFailureDescription(
"Configuration cache problems found in this build.",
spec
)
}
private static Matcher failureDescriptionMatcherForTooManyProblems(HasConfigurationCacheProblemsSpec spec) {
return buildMatcherForProblemsFailureDescription(
"Maximum number of configuration cache problems has been reached.\n" +
"This behavior can be adjusted. " +
new DocumentationRegistry().getDocumentationRecommendationFor("on this", "configuration_cache", "config_cache:usage:max_problems"),
spec
)
}
private static Matcher buildMatcherForProblemsFailureDescription(
String message,
HasConfigurationCacheProblemsSpec spec
) {
return new BaseMatcher() {
@Override
boolean matches(Object item) {
if (!item.toString().contains(message)) {
return false
}
assertHasConsoleSummary(item.toString(), spec)
return true
}
@Override
void describeTo(Description description) {
description.appendText("contains expected problems")
}
}
}
private static void assertNoProblemsSummary(String text) {
assertThat(text, not(containsString("configuration cache problem")))
}
private static void assertFailureDescription(
ExecutionFailure failure,
Matcher failureMatcher
) {
failure.assertThatDescription(failureMatcher)
}
private static void assertHasConsoleSummary(String text, HasConfigurationCacheProblemsSpec spec) {
def uniqueCount = spec.uniqueProblems.size()
def totalCount = spec.totalProblemsCount ?: uniqueCount
def summary = extractSummary(text)
assert summary.totalProblems == totalCount
assert summary.uniqueProblems == uniqueCount
assert summary.messages.size() == spec.uniqueProblems.size()
for (int i in spec.uniqueProblems.indices) {
assert spec.uniqueProblems[i].matches(summary.messages[i])
}
}
private static void assertProblemsHtmlReport(
String output,
File rootDir,
HasConfigurationCacheProblemsSpec spec
) {
def totalProblemCount = spec.totalProblemsCount ?: spec.uniqueProblems.size()
assertProblemsHtmlReport(
rootDir,
output,
totalProblemCount,
spec.uniqueProblems.size(),
spec.problemsWithStackTraceCount == null ? totalProblemCount : spec.problemsWithStackTraceCount
)
}
private static void assertInputs(
String output,
File rootDir,
InputsSpec spec
) {
if (spec == InputsSpec.IGNORING) {
return
}
List> expectedInputs = spec instanceof InputsSpec.ExpectingSome
? spec.inputs.collect()
: []
def reportDir = resolveConfigurationCacheReportDirectory(rootDir, output)
if (reportDir == null) {
assertThat(
"Expecting inputs but no report was found",
expectedInputs,
equalTo([])
)
return
}
Map jsModel = readJsModelFromReportDir(reportDir)
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy