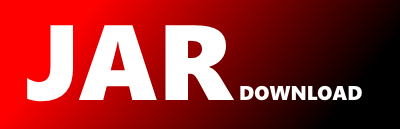
org.gradle.performance.fixture.AbstractCrossBuildPerformanceTestRunner.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-api Show documentation
Show all versions of gradle-api Show documentation
Gradle 6.9.1 API redistribution.
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gradle.performance.fixture
import groovy.transform.CompileStatic
import org.gradle.integtests.fixtures.executer.GradleDistribution
import org.gradle.integtests.fixtures.executer.IntegrationTestBuildContext
import org.gradle.integtests.fixtures.executer.UnderDevelopmentGradleDistribution
import org.gradle.internal.time.Clock
import org.gradle.internal.time.Time
import org.gradle.performance.results.CrossBuildPerformanceResults
import org.gradle.performance.results.DataReporter
import org.gradle.performance.results.ResultsStoreHelper
import org.gradle.profiler.BuildMutator
import org.gradle.profiler.InvocationSettings
import org.junit.Assume
import java.util.function.Function
@CompileStatic
abstract class AbstractCrossBuildPerformanceTestRunner {
private final List> buildMutators = []
private final List measuredBuildOperations = []
final IntegrationTestBuildContext buildContext
final GradleDistribution gradleDistribution
final BuildExperimentRunner experimentRunner
final Clock clock = Time.clock()
String testClassName
String testId
String testGroup
String testProject
List specs = []
boolean measureGarbageCollection = true
final DataReporter reporter
AbstractCrossBuildPerformanceTestRunner(BuildExperimentRunner experimentRunner, DataReporter dataReporter, IntegrationTestBuildContext buildContext) {
this.reporter = dataReporter
this.experimentRunner = experimentRunner
this.buildContext = buildContext
this.gradleDistribution = new UnderDevelopmentGradleDistribution(buildContext)
this.testProject = TestScenarioSelector.loadConfiguredTestProject()
}
void baseline(@DelegatesTo(GradleBuildExperimentSpec.GradleBuilder) Closure> configureAction) {
buildSpec(configureAction)
}
void buildSpec(@DelegatesTo(GradleBuildExperimentSpec.GradleBuilder) Closure> configureAction) {
def builder = GradleBuildExperimentSpec.builder()
builder.projectName = testProject
configureGradleSpec(builder)
configureAndAddSpec(builder, configureAction)
}
void addBuildMutator(Function buildMutator) {
buildMutators.add(buildMutator)
}
void measureBuildOperation(String operation) {
measuredBuildOperations << operation
}
protected void configureGradleSpec(GradleBuildExperimentSpec.GradleBuilder builder) {
builder.measuredBuildOperations.addAll(measuredBuildOperations)
builder.measureGarbageCollection(measureGarbageCollection)
builder.invocation.distribution(gradleDistribution)
}
protected void configureAndAddSpec(BuildExperimentSpec.Builder builder, Closure> configureAction) {
defaultSpec(builder)
builder.with(configureAction as Closure
© 2015 - 2025 Weber Informatics LLC | Privacy Policy