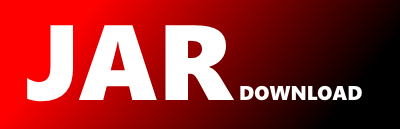
dev.gradleplugins.runnerkit.ClassUtils Maven / Gradle / Ivy
package dev.gradleplugins.runnerkit;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
final class ClassUtils {
@SuppressWarnings("unchecked")
public static T staticInvoke(String className, String methodName, Class>[] parameterTypes, Object... args) {
try {
Class> clazz = Class.forName(className);
Method method = clazz.getMethod(methodName, parameterTypes);
return (T) method.invoke(null, args);
} catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException("Please verify your dependencies on runnerKit.", e);
}
}
@SuppressWarnings("unchecked")
public static T newInstance(String className) {
try {
Class> clazz = Class.forName(className);
Constructor constructor = (Constructor) clazz.getDeclaredConstructor();
return (T) constructor.newInstance();
} catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException | InvocationTargetException | InstantiationException e) {
throw new RuntimeException("Please verify your dependencies on runnerKit.", e);
}
}
@SuppressWarnings("unchecked")
public static T newInstance(String className, Class>[] parameterTypes, Object... args) {
try {
Class> clazz = Class.forName(className);
Constructor constructor = (Constructor) clazz.getDeclaredConstructor(parameterTypes);
return (T) constructor.newInstance(args);
} catch (ClassNotFoundException | NoSuchMethodException | IllegalAccessException | InvocationTargetException | InstantiationException e) {
throw new RuntimeException("Please verify your dependencies on runnerKit.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy