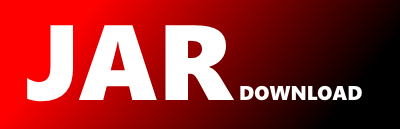
dev.harrel.jsonschema.Dialects Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema Show documentation
Show all versions of json-schema Show documentation
Library for JSON schema validation
package dev.harrel.jsonschema;
import java.net.URI;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import static dev.harrel.jsonschema.Vocabulary.*;
import static java.util.Collections.singleton;
import static java.util.Collections.unmodifiableMap;
/**
* Static container class for all officially supported dialects.
*/
public final class Dialects {
private Dialects() {}
static final Map OFFICIAL_DIALECTS;
static {
Map map = new HashMap<>();
map.put(URI.create(SpecificationVersion.DRAFT2020_12.getId()), new Draft2020Dialect());
map.put(URI.create(SpecificationVersion.DRAFT2019_09.getId()), new Draft2019Dialect());
map.put(UriUtil.removeEmptyFragment(SpecificationVersion.DRAFT7.getId()), new Draft7Dialect());
OFFICIAL_DIALECTS = Collections.unmodifiableMap(map);
}
/**
* Dialect corresponding to draft2020-12 specification.
*/
public static class Draft2020Dialect implements Dialect {
private final EvaluatorFactory evaluatorFactory;
private final Set requiredVocabularies;
private final Map defaultVocabularyObject;
public Draft2020Dialect() {
this.evaluatorFactory = new Draft2020EvaluatorFactory();
this.requiredVocabularies = singleton(Draft2020.CORE);
Map vocabs = new HashMap<>();
vocabs.put(Draft2020.CORE, true);
vocabs.put(Draft2020.APPLICATOR, true);
vocabs.put(Draft2020.UNEVALUATED, true);
vocabs.put(Draft2020.VALIDATION, true);
vocabs.put(Draft2020.META_DATA, true);
vocabs.put(Draft2020.FORMAT_ANNOTATION, true);
vocabs.put(Draft2020.CONTENT, true);
this.defaultVocabularyObject = unmodifiableMap(vocabs);
}
@Override
public SpecificationVersion getSpecificationVersion() {
return SpecificationVersion.DRAFT2020_12;
}
@Override
public String getMetaSchema() {
return SpecificationVersion.DRAFT2020_12.getId();
}
@Override
public EvaluatorFactory getEvaluatorFactory() {
return evaluatorFactory;
}
@Override
public Set getSupportedVocabularies() {
return defaultVocabularyObject.keySet();
}
@Override
public Set getRequiredVocabularies() {
return requiredVocabularies;
}
@Override
public Map getDefaultVocabularyObject() {
return defaultVocabularyObject;
}
}
/**
* Dialect corresponding to draft2019-09 specification.
*/
public static class Draft2019Dialect implements Dialect {
private final EvaluatorFactory evaluatorFactory;
private final Set requiredVocabularies;
private final Map defaultVocabularyObject;
public Draft2019Dialect() {
this.evaluatorFactory = new Draft2019EvaluatorFactory();
this.requiredVocabularies = singleton(Draft2019.CORE);
Map vocabs = new HashMap<>();
vocabs.put(Draft2019.CORE, true);
vocabs.put(Draft2019.APPLICATOR, true);
vocabs.put(Draft2019.VALIDATION, true);
vocabs.put(Draft2019.META_DATA, true);
vocabs.put(Draft2019.FORMAT, false);
vocabs.put(Draft2019.CONTENT, true);
this.defaultVocabularyObject = unmodifiableMap(vocabs);
}
@Override
public SpecificationVersion getSpecificationVersion() {
return SpecificationVersion.DRAFT2019_09;
}
@Override
public String getMetaSchema() {
return SpecificationVersion.DRAFT2019_09.getId();
}
@Override
public EvaluatorFactory getEvaluatorFactory() {
return evaluatorFactory;
}
@Override
public Set getSupportedVocabularies() {
return defaultVocabularyObject.keySet();
}
@Override
public Set getRequiredVocabularies() {
return requiredVocabularies;
}
@Override
public Map getDefaultVocabularyObject() {
return defaultVocabularyObject;
}
}
/**
* Dialect corresponding to draft7 specification.
*/
public static class Draft7Dialect implements Dialect {
private final EvaluatorFactory evaluatorFactory;
public Draft7Dialect() {
this.evaluatorFactory = new Draft7EvaluatorFactory();
}
@Override
public SpecificationVersion getSpecificationVersion() {
return SpecificationVersion.DRAFT7;
}
@Override
public String getMetaSchema() {
return SpecificationVersion.DRAFT7.getId();
}
@Override
public EvaluatorFactory getEvaluatorFactory() {
return evaluatorFactory;
}
@Override
public Set getSupportedVocabularies() {
return Collections.emptySet();
}
@Override
public Set getRequiredVocabularies() {
return Collections.emptySet();
}
@Override
public Map getDefaultVocabularyObject() {
return Collections.emptyMap();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy