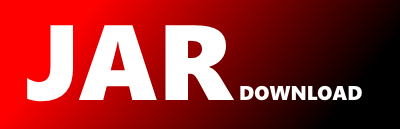
dev.harrel.jsonschema.EvaluationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema Show documentation
Show all versions of json-schema Show documentation
Library for JSON schema validation
package dev.harrel.jsonschema;
import java.net.URI;
import java.util.*;
import static java.util.Collections.unmodifiableList;
/**
* {@code EvaluationContext} class represents state of current evaluation (instance validation against schema).
* {@link Evaluator} can use this class for its processing logic.
*
* @see Evaluator
*/
public final class EvaluationContext {
private final JsonNodeFactory jsonNodeFactory;
private final JsonParser jsonParser;
private final SchemaRegistry schemaRegistry;
private final SchemaResolver schemaResolver;
private final Deque dynamicScope = new ArrayDeque<>();
private final Deque refStack = new ArrayDeque<>();
private final LinkedList evaluationStack = new LinkedList<>();
private final AnnotationTree annotationTree = new AnnotationTree();
private final List errors = new ArrayList<>();
EvaluationContext(JsonNodeFactory jsonNodeFactory,
JsonParser jsonParser,
SchemaRegistry schemaRegistry,
SchemaResolver schemaResolver) {
this.jsonNodeFactory = Objects.requireNonNull(jsonNodeFactory);
this.jsonParser = Objects.requireNonNull(jsonParser);
this.schemaRegistry = Objects.requireNonNull(schemaRegistry);
this.schemaResolver = Objects.requireNonNull(schemaResolver);
this.evaluationStack.push("");
}
/**
* Resolves schema using provided reference string, and then validates instance node against it.
* This method can invoke {@link SchemaResolver}.
*
* @param schemaRef reference to the schema
* @param node instance node to be validated
* @return if validation was successful
* @throws SchemaNotFoundException when schema cannot be resolved
*/
public boolean resolveRefAndValidate(String schemaRef, JsonNode node) {
return resolveRefAndValidate(CompoundUri.fromString(schemaRef), node);
}
boolean resolveRefAndValidate(CompoundUri compoundUri, JsonNode node) {
Schema schema = resolveSchema(compoundUri);
if (schema == null) {
throw new SchemaNotFoundException(compoundUri);
}
return validateAgainstRefSchema(schema, node);
}
/**
* Dynamically resolves schema using provided reference string, and then validates instance node against it.
* This method is specifically created for $dynamicRef keyword.
*
* @param schemaRef reference to the schema
* @param node instance node to be validated
* @return if validation was successful
* @throws SchemaNotFoundException when schema cannot be resolved
*/
public boolean resolveDynamicRefAndValidate(String schemaRef, JsonNode node) {
return resolveDynamicRefAndValidate(CompoundUri.fromString(schemaRef), node);
}
boolean resolveDynamicRefAndValidate(CompoundUri compoundUri, JsonNode node) {
Schema schema = resolveDynamicSchema(compoundUri);
if (schema == null) {
throw new SchemaNotFoundException(compoundUri);
}
return validateAgainstRefSchema(schema, node);
}
/**
* Recursively resolves schema using provided reference string (current implementation behaves the same for any reference string),
* and then validates instance node against it.
* This method is specifically created for $recursiveRef keyword.
*
* @param schemaRef reference to the schema (specification-wise this should always have a value of '#')
* @param node instance node to be validated
* @return if validation was successful
* @throws SchemaNotFoundException when schema cannot be resolved
*/
public boolean resolveRecursiveRefAndValidate(String schemaRef, JsonNode node) {
Schema schema = resolveRecursiveSchema();
if (schema == null) {
throw new SchemaNotFoundException(CompoundUri.fromString(schemaRef));
}
return validateAgainstRefSchema(schema, node);
}
/**
* Resolves internal schema using provided reference string and then validates instance node against it.
* This method should only be used for internal schema resolutions, that means schema/evaluator calling this
* method should only refer to schema instances which are descendants of calling node.
* Note that this method is semantically different from {@link EvaluationContext#resolveRefAndValidate} and it
* cannot invoke {@link SchemaResolver}.
*
* @param schemaRef reference to the schema
* @param node instance node to be validated
* @return if validation was successful
* @throws SchemaNotFoundException when schema cannot be resolved
*/
public boolean resolveInternalRefAndValidate(String schemaRef, JsonNode node) {
return resolveInternalRefAndValidate(CompoundUri.fromString(schemaRef), node);
}
boolean resolveInternalRefAndValidate(CompoundUri compoundUri, JsonNode node) {
Schema schema = schemaRegistry.get(compoundUri);
if (schema == null) {
throw new SchemaNotFoundException(compoundUri);
}
return validateAgainstSchema(schema, node);
}
List getErrors() {
return unmodifiableList(errors);
}
Optional getSiblingAnnotation(String sibling, String instanceLocation, Class annotationType) {
return getSiblingAnnotation(sibling, instanceLocation)
.filter(annotationType::isInstance)
.map(annotationType::cast);
}
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy