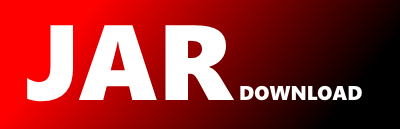
io.activej.common.StringFormatUtils Maven / Gradle / Ivy
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.common;
import java.time.Duration;
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.Period;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeFormatterBuilder;
import java.time.format.DateTimeParseException;
import java.util.HashSet;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static java.lang.Math.round;
import static java.time.format.DateTimeFormatter.ISO_LOCAL_DATE;
import static java.time.format.DateTimeFormatter.ISO_LOCAL_TIME;
public final class StringFormatUtils {
public static String formatMemSize(MemSize memSize) {
long bytes = memSize.toLong();
if (bytes == 0) {
return "0";
}
for (long unit = MemSize.TB; ; unit /= 1024L) {
long divideResult = bytes / unit;
long remainder = bytes % unit;
if (divideResult == 0) {
continue;
}
if (remainder == 0) {
return divideResult + getUnit(unit);
}
}
}
private static String getUnit(long unit) {
if (unit == MemSize.TB) {
return "Tb";
} else {
switch ((int) unit) {
case (int) MemSize.GB:
return "Gb";
case (int) MemSize.MB:
return "Mb";
case (int) MemSize.KB:
return "Kb";
case 1:
return "";
default:
throw new IllegalArgumentException("Wrong unit");
}
}
}
private static final Pattern MEM_SIZE_PATTERN = Pattern.compile("(?\\d+)([.](?\\d+))?\\s*(?(|g|m|k|t)b?)?(\\s+|$)", Pattern.CASE_INSENSITIVE);
public static MemSize parseMemSize(String string) {
Set units = new HashSet<>();
Matcher matcher = MEM_SIZE_PATTERN.matcher(string.trim().toLowerCase());
long result = 0;
int lastEnd = 0;
while (!matcher.hitEnd()) {
if (!matcher.find() || matcher.start() != lastEnd) {
throw new IllegalArgumentException("Invalid MemSize: " + string);
}
lastEnd = matcher.end();
String unit = matcher.group("unit");
if (unit == null) {
unit = "";
}
if (!unit.endsWith("b")) {
unit += "b";
}
if (!units.add(unit)) {
throw new IllegalArgumentException("Memory unit " + unit + " occurs more than once in: " + string);
}
long memsize = Long.parseLong(matcher.group("size"));
long numerator = 0;
long denominator = 1;
String floatingPoint = matcher.group("floating");
if (floatingPoint != null) {
if (unit.equals("b")) {
throw new IllegalArgumentException("MemSize unit bytes cannot be fractional");
}
numerator = Long.parseLong(floatingPoint);
for (int i = 0; i < floatingPoint.length(); i++) {
denominator *= 10;
}
}
double fractional = (double) numerator / denominator;
switch (unit) {
case "tb":
result += memsize * MemSize.TB;
result += round(MemSize.TB * fractional);
break;
case "gb":
result += memsize * MemSize.GB;
result += round(MemSize.GB * fractional);
break;
case "mb":
result += memsize * MemSize.MB;
result += round(MemSize.MB * fractional);
break;
case "kb":
result += memsize * MemSize.KB;
result += round(MemSize.KB * fractional);
break;
case "b":
case "":
result += memsize;
break;
}
}
return MemSize.of(result);
}
public static String formatDuration(Duration value) {
if (value.isZero()) {
return "0 seconds";
}
String result = "";
long days, hours, minutes, seconds, nano, milli;
days = value.toDays();
if (days != 0) {
result += days + " days ";
}
hours = value.toHours() - days * 24;
if (hours != 0) {
result += hours + " hours ";
}
minutes = value.toMinutes() - days * 1440 - hours * 60;
if (minutes != 0) {
result += minutes + " minutes ";
}
seconds = value.getSeconds() - days * 86400 - hours * 3600 - minutes * 60;
if (seconds != 0) {
result += seconds + " seconds ";
}
nano = value.getNano();
milli = (nano - nano % 1000000) / 1000000;
if (milli != 0) {
result += milli + " millis ";
}
nano = nano % 1000000;
if (nano != 0) {
result += nano + " nanos ";
}
return result.trim();
}
private static final Pattern DURATION_PATTERN = Pattern.compile("(?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy