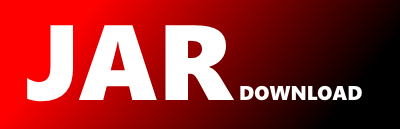
org.hl7.fhir.r4.model.Contract Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.hl7.fhir.r4 Show documentation
Show all versions of org.hl7.fhir.r4 Show documentation
Builds the hapi fhir r4. Requires hapi-fhir-base and hapi-fhir-utilities be built first and be
excluded from any other poms requiring it.
The newest version!
package org.hl7.fhir.r4.model;
import java.math.BigDecimal;
/*
Copyright (c) 2011+, HL7, Inc.
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
* Neither the name of HL7 nor the names of its contributors may be used to
endorse or promote products derived from this software without specific
prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
POSSIBILITY OF SUCH DAMAGE.
*/
// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.hl7.fhir.exceptions.FHIRException;
import org.hl7.fhir.instance.model.api.IBaseBackboneElement;
import org.hl7.fhir.utilities.Utilities;
import ca.uhn.fhir.model.api.annotation.Block;
import ca.uhn.fhir.model.api.annotation.Child;
import ca.uhn.fhir.model.api.annotation.Description;
import ca.uhn.fhir.model.api.annotation.ResourceDef;
import ca.uhn.fhir.model.api.annotation.SearchParamDefinition;
/**
* Legally enforceable, formally recorded unilateral or bilateral directive
* i.e., a policy or agreement.
*/
@ResourceDef(name = "Contract", profile = "http://hl7.org/fhir/StructureDefinition/Contract")
public class Contract extends DomainResource {
public enum ContractStatus {
/**
* Contract is augmented with additional information to correct errors in a
* predecessor or to updated values in a predecessor. Usage: Contract altered
* within effective time. Precedence Order = 9. Comparable FHIR and v.3 status
* codes: revised; replaced.
*/
AMENDED,
/**
* Contract is augmented with additional information that was missing from a
* predecessor Contract. Usage: Contract altered within effective time.
* Precedence Order = 9. Comparable FHIR and v.3 status codes: updated,
* replaced.
*/
APPENDED,
/**
* Contract is terminated due to failure of the Grantor and/or the Grantee to
* fulfil one or more contract provisions. Usage: Abnormal contract termination.
* Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed;
* aborted.
*/
CANCELLED,
/**
* Contract is pended to rectify failure of the Grantor or the Grantee to fulfil
* contract provision(s). E.g., Grantee complaint about Grantor's failure to
* comply with contract provisions. Usage: Contract pended. Precedence Order =
* 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended.
*/
DISPUTED,
/**
* Contract was created in error. No Precedence Order. Status may be applied to
* a Contract with any status.
*/
ENTEREDINERROR,
/**
* Contract execution pending; may be executed when either the Grantor or the
* Grantee accepts the contract provisions by signing. I.e., where either the
* Grantor or the Grantee has signed, but not both. E.g., when an insurance
* applicant signs the insurers application, which references the policy. Usage:
* Optional first step of contract execution activity. May be skipped and
* contracting activity moves directly to executed state. Precedence Order = 3.
* Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended;
* active.
*/
EXECUTABLE,
/**
* Contract is activated for period stipulated when both the Grantor and Grantee
* have signed it. Usage: Required state for normal completion of contracting
* activity. Precedence Order = 6. Comparable FHIR and v.3 status codes:
* accepted; completed.
*/
EXECUTED,
/**
* Contract execution is suspended while either or both the Grantor and Grantee
* propose and consider new or revised contract provisions. I.e., where the
* party which has not signed proposes changes to the terms. E .g., a life
* insurer declines to agree to the signed application because the life insurer
* has evidence that the applicant, who asserted to being younger or a
* non-smoker to get a lower premium rate - but offers instead to agree to a
* higher premium based on the applicants actual age or smoking status. Usage:
* Optional contract activity between executable and executed state. Precedence
* Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held.
*/
NEGOTIABLE,
/**
* Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract
* hard copy or electronic 'template', 'form' or 'application'. E.g., health
* insurance application; consent directive form. Usage: Beginning of contract
* negotiation, which may have been completed as a precondition because used for
* 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes:
* requested; new.
*/
OFFERED,
/**
* Contract template is available as the basis for an application or offer by
* the Grantor or Grantee. E.g., health insurance policy; consent directive
* policy. Usage: Required initial contract activity, which may have been
* completed as a precondition because used for 0..* contracts. Precedence Order
* = 1. Comparable FHIR and v.3 status codes: proposed; intended.
*/
POLICY,
/**
* Execution of the Contract is not completed because either or both the Grantor
* and Grantee decline to accept some or all of the contract provisions. Usage:
* Optional contract activity between executable and abnormal termination.
* Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped;
* cancelled.
*/
REJECTED,
/**
* Beginning of a successor Contract at the termination of predecessor Contract
* lifecycle. Usage: Follows termination of a preceding Contract that has
* reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3
* status codes: superseded.
*/
RENEWED,
/**
* A Contract that is rescinded. May be required prior to replacing with an
* updated Contract. Comparable FHIR and v.3 status codes: nullified.
*/
REVOKED,
/**
* Contract is reactivated after being pended because of faulty execution.
* *E.g., competency of the signer(s), or where the policy is substantially
* different from and did not accompany the application/form so that the
* applicant could not compare them. Aka - ''reactivated''. Usage: Optional
* stage where a pended contract is reactivated. Precedence Order = 8.
* Comparable FHIR and v.3 status codes: reactivated.
*/
RESOLVED,
/**
* Contract reaches its expiry date. It might or might not be renewed or
* renegotiated. Usage: Normal end of contract period. Precedence Order = 12.
* Comparable FHIR and v.3 status codes: Obsoleted.
*/
TERMINATED,
/**
* added to help the parsers with the generic types
*/
NULL;
public static ContractStatus fromCode(String codeString) throws FHIRException {
if (codeString == null || "".equals(codeString))
return null;
if ("amended".equals(codeString))
return AMENDED;
if ("appended".equals(codeString))
return APPENDED;
if ("cancelled".equals(codeString))
return CANCELLED;
if ("disputed".equals(codeString))
return DISPUTED;
if ("entered-in-error".equals(codeString))
return ENTEREDINERROR;
if ("executable".equals(codeString))
return EXECUTABLE;
if ("executed".equals(codeString))
return EXECUTED;
if ("negotiable".equals(codeString))
return NEGOTIABLE;
if ("offered".equals(codeString))
return OFFERED;
if ("policy".equals(codeString))
return POLICY;
if ("rejected".equals(codeString))
return REJECTED;
if ("renewed".equals(codeString))
return RENEWED;
if ("revoked".equals(codeString))
return REVOKED;
if ("resolved".equals(codeString))
return RESOLVED;
if ("terminated".equals(codeString))
return TERMINATED;
if (Configuration.isAcceptInvalidEnums())
return null;
else
throw new FHIRException("Unknown ContractStatus code '" + codeString + "'");
}
public String toCode() {
switch (this) {
case AMENDED:
return "amended";
case APPENDED:
return "appended";
case CANCELLED:
return "cancelled";
case DISPUTED:
return "disputed";
case ENTEREDINERROR:
return "entered-in-error";
case EXECUTABLE:
return "executable";
case EXECUTED:
return "executed";
case NEGOTIABLE:
return "negotiable";
case OFFERED:
return "offered";
case POLICY:
return "policy";
case REJECTED:
return "rejected";
case RENEWED:
return "renewed";
case REVOKED:
return "revoked";
case RESOLVED:
return "resolved";
case TERMINATED:
return "terminated";
case NULL:
return null;
default:
return "?";
}
}
public String getSystem() {
switch (this) {
case AMENDED:
return "http://hl7.org/fhir/contract-status";
case APPENDED:
return "http://hl7.org/fhir/contract-status";
case CANCELLED:
return "http://hl7.org/fhir/contract-status";
case DISPUTED:
return "http://hl7.org/fhir/contract-status";
case ENTEREDINERROR:
return "http://hl7.org/fhir/contract-status";
case EXECUTABLE:
return "http://hl7.org/fhir/contract-status";
case EXECUTED:
return "http://hl7.org/fhir/contract-status";
case NEGOTIABLE:
return "http://hl7.org/fhir/contract-status";
case OFFERED:
return "http://hl7.org/fhir/contract-status";
case POLICY:
return "http://hl7.org/fhir/contract-status";
case REJECTED:
return "http://hl7.org/fhir/contract-status";
case RENEWED:
return "http://hl7.org/fhir/contract-status";
case REVOKED:
return "http://hl7.org/fhir/contract-status";
case RESOLVED:
return "http://hl7.org/fhir/contract-status";
case TERMINATED:
return "http://hl7.org/fhir/contract-status";
case NULL:
return null;
default:
return "?";
}
}
public String getDefinition() {
switch (this) {
case AMENDED:
return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced.";
case APPENDED:
return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced.";
case CANCELLED:
return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted.";
case DISPUTED:
return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended.";
case ENTEREDINERROR:
return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status.";
case EXECUTABLE:
return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active.";
case EXECUTED:
return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed.";
case NEGOTIABLE:
return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held.";
case OFFERED:
return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new.";
case POLICY:
return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended.";
case REJECTED:
return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled.";
case RENEWED:
return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded.";
case REVOKED:
return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified.";
case RESOLVED:
return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated.";
case TERMINATED:
return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted.";
case NULL:
return null;
default:
return "?";
}
}
public String getDisplay() {
switch (this) {
case AMENDED:
return "Amended";
case APPENDED:
return "Appended";
case CANCELLED:
return "Cancelled";
case DISPUTED:
return "Disputed";
case ENTEREDINERROR:
return "Entered in Error";
case EXECUTABLE:
return "Executable";
case EXECUTED:
return "Executed";
case NEGOTIABLE:
return "Negotiable";
case OFFERED:
return "Offered";
case POLICY:
return "Policy";
case REJECTED:
return "Rejected";
case RENEWED:
return "Renewed";
case REVOKED:
return "Revoked";
case RESOLVED:
return "Resolved";
case TERMINATED:
return "Terminated";
case NULL:
return null;
default:
return "?";
}
}
}
public static class ContractStatusEnumFactory implements EnumFactory {
public ContractStatus fromCode(String codeString) throws IllegalArgumentException {
if (codeString == null || "".equals(codeString))
if (codeString == null || "".equals(codeString))
return null;
if ("amended".equals(codeString))
return ContractStatus.AMENDED;
if ("appended".equals(codeString))
return ContractStatus.APPENDED;
if ("cancelled".equals(codeString))
return ContractStatus.CANCELLED;
if ("disputed".equals(codeString))
return ContractStatus.DISPUTED;
if ("entered-in-error".equals(codeString))
return ContractStatus.ENTEREDINERROR;
if ("executable".equals(codeString))
return ContractStatus.EXECUTABLE;
if ("executed".equals(codeString))
return ContractStatus.EXECUTED;
if ("negotiable".equals(codeString))
return ContractStatus.NEGOTIABLE;
if ("offered".equals(codeString))
return ContractStatus.OFFERED;
if ("policy".equals(codeString))
return ContractStatus.POLICY;
if ("rejected".equals(codeString))
return ContractStatus.REJECTED;
if ("renewed".equals(codeString))
return ContractStatus.RENEWED;
if ("revoked".equals(codeString))
return ContractStatus.REVOKED;
if ("resolved".equals(codeString))
return ContractStatus.RESOLVED;
if ("terminated".equals(codeString))
return ContractStatus.TERMINATED;
throw new IllegalArgumentException("Unknown ContractStatus code '" + codeString + "'");
}
public Enumeration fromType(PrimitiveType> code) throws FHIRException {
if (code == null)
return null;
if (code.isEmpty())
return new Enumeration(this, ContractStatus.NULL, code);
String codeString = code.asStringValue();
if (codeString == null || "".equals(codeString))
return new Enumeration(this, ContractStatus.NULL, code);
if ("amended".equals(codeString))
return new Enumeration(this, ContractStatus.AMENDED, code);
if ("appended".equals(codeString))
return new Enumeration(this, ContractStatus.APPENDED, code);
if ("cancelled".equals(codeString))
return new Enumeration(this, ContractStatus.CANCELLED, code);
if ("disputed".equals(codeString))
return new Enumeration(this, ContractStatus.DISPUTED, code);
if ("entered-in-error".equals(codeString))
return new Enumeration(this, ContractStatus.ENTEREDINERROR, code);
if ("executable".equals(codeString))
return new Enumeration(this, ContractStatus.EXECUTABLE, code);
if ("executed".equals(codeString))
return new Enumeration(this, ContractStatus.EXECUTED, code);
if ("negotiable".equals(codeString))
return new Enumeration(this, ContractStatus.NEGOTIABLE, code);
if ("offered".equals(codeString))
return new Enumeration(this, ContractStatus.OFFERED, code);
if ("policy".equals(codeString))
return new Enumeration(this, ContractStatus.POLICY, code);
if ("rejected".equals(codeString))
return new Enumeration(this, ContractStatus.REJECTED, code);
if ("renewed".equals(codeString))
return new Enumeration(this, ContractStatus.RENEWED, code);
if ("revoked".equals(codeString))
return new Enumeration(this, ContractStatus.REVOKED, code);
if ("resolved".equals(codeString))
return new Enumeration(this, ContractStatus.RESOLVED, code);
if ("terminated".equals(codeString))
return new Enumeration(this, ContractStatus.TERMINATED, code);
throw new FHIRException("Unknown ContractStatus code '" + codeString + "'");
}
public String toCode(ContractStatus code) {
if (code == ContractStatus.AMENDED)
return "amended";
if (code == ContractStatus.APPENDED)
return "appended";
if (code == ContractStatus.CANCELLED)
return "cancelled";
if (code == ContractStatus.DISPUTED)
return "disputed";
if (code == ContractStatus.ENTEREDINERROR)
return "entered-in-error";
if (code == ContractStatus.EXECUTABLE)
return "executable";
if (code == ContractStatus.EXECUTED)
return "executed";
if (code == ContractStatus.NEGOTIABLE)
return "negotiable";
if (code == ContractStatus.OFFERED)
return "offered";
if (code == ContractStatus.POLICY)
return "policy";
if (code == ContractStatus.REJECTED)
return "rejected";
if (code == ContractStatus.RENEWED)
return "renewed";
if (code == ContractStatus.REVOKED)
return "revoked";
if (code == ContractStatus.RESOLVED)
return "resolved";
if (code == ContractStatus.TERMINATED)
return "terminated";
return "?";
}
public String toSystem(ContractStatus code) {
return code.getSystem();
}
}
public enum ContractPublicationStatus {
/**
* Contract is augmented with additional information to correct errors in a
* predecessor or to updated values in a predecessor. Usage: Contract altered
* within effective time. Precedence Order = 9. Comparable FHIR and v.3 status
* codes: revised; replaced.
*/
AMENDED,
/**
* Contract is augmented with additional information that was missing from a
* predecessor Contract. Usage: Contract altered within effective time.
* Precedence Order = 9. Comparable FHIR and v.3 status codes: updated,
* replaced.
*/
APPENDED,
/**
* Contract is terminated due to failure of the Grantor and/or the Grantee to
* fulfil one or more contract provisions. Usage: Abnormal contract termination.
* Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed;
* aborted.
*/
CANCELLED,
/**
* Contract is pended to rectify failure of the Grantor or the Grantee to fulfil
* contract provision(s). E.g., Grantee complaint about Grantor's failure to
* comply with contract provisions. Usage: Contract pended. Precedence Order =
* 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended.
*/
DISPUTED,
/**
* Contract was created in error. No Precedence Order. Status may be applied to
* a Contract with any status.
*/
ENTEREDINERROR,
/**
* Contract execution pending; may be executed when either the Grantor or the
* Grantee accepts the contract provisions by signing. I.e., where either the
* Grantor or the Grantee has signed, but not both. E.g., when an insurance
* applicant signs the insurers application, which references the policy. Usage:
* Optional first step of contract execution activity. May be skipped and
* contracting activity moves directly to executed state. Precedence Order = 3.
* Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended;
* active.
*/
EXECUTABLE,
/**
* Contract is activated for period stipulated when both the Grantor and Grantee
* have signed it. Usage: Required state for normal completion of contracting
* activity. Precedence Order = 6. Comparable FHIR and v.3 status codes:
* accepted; completed.
*/
EXECUTED,
/**
* Contract execution is suspended while either or both the Grantor and Grantee
* propose and consider new or revised contract provisions. I.e., where the
* party which has not signed proposes changes to the terms. E .g., a life
* insurer declines to agree to the signed application because the life insurer
* has evidence that the applicant, who asserted to being younger or a
* non-smoker to get a lower premium rate - but offers instead to agree to a
* higher premium based on the applicants actual age or smoking status. Usage:
* Optional contract activity between executable and executed state. Precedence
* Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held.
*/
NEGOTIABLE,
/**
* Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract
* hard copy or electronic 'template', 'form' or 'application'. E.g., health
* insurance application; consent directive form. Usage: Beginning of contract
* negotiation, which may have been completed as a precondition because used for
* 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes:
* requested; new.
*/
OFFERED,
/**
* Contract template is available as the basis for an application or offer by
* the Grantor or Grantee. E.g., health insurance policy; consent directive
* policy. Usage: Required initial contract activity, which may have been
* completed as a precondition because used for 0..* contracts. Precedence Order
* = 1. Comparable FHIR and v.3 status codes: proposed; intended.
*/
POLICY,
/**
* Execution of the Contract is not completed because either or both the Grantor
* and Grantee decline to accept some or all of the contract provisions. Usage:
* Optional contract activity between executable and abnormal termination.
* Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped;
* cancelled.
*/
REJECTED,
/**
* Beginning of a successor Contract at the termination of predecessor Contract
* lifecycle. Usage: Follows termination of a preceding Contract that has
* reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3
* status codes: superseded.
*/
RENEWED,
/**
* A Contract that is rescinded. May be required prior to replacing with an
* updated Contract. Comparable FHIR and v.3 status codes: nullified.
*/
REVOKED,
/**
* Contract is reactivated after being pended because of faulty execution.
* *E.g., competency of the signer(s), or where the policy is substantially
* different from and did not accompany the application/form so that the
* applicant could not compare them. Aka - ''reactivated''. Usage: Optional
* stage where a pended contract is reactivated. Precedence Order = 8.
* Comparable FHIR and v.3 status codes: reactivated.
*/
RESOLVED,
/**
* Contract reaches its expiry date. It might or might not be renewed or
* renegotiated. Usage: Normal end of contract period. Precedence Order = 12.
* Comparable FHIR and v.3 status codes: Obsoleted.
*/
TERMINATED,
/**
* added to help the parsers with the generic types
*/
NULL;
public static ContractPublicationStatus fromCode(String codeString) throws FHIRException {
if (codeString == null || "".equals(codeString))
return null;
if ("amended".equals(codeString))
return AMENDED;
if ("appended".equals(codeString))
return APPENDED;
if ("cancelled".equals(codeString))
return CANCELLED;
if ("disputed".equals(codeString))
return DISPUTED;
if ("entered-in-error".equals(codeString))
return ENTEREDINERROR;
if ("executable".equals(codeString))
return EXECUTABLE;
if ("executed".equals(codeString))
return EXECUTED;
if ("negotiable".equals(codeString))
return NEGOTIABLE;
if ("offered".equals(codeString))
return OFFERED;
if ("policy".equals(codeString))
return POLICY;
if ("rejected".equals(codeString))
return REJECTED;
if ("renewed".equals(codeString))
return RENEWED;
if ("revoked".equals(codeString))
return REVOKED;
if ("resolved".equals(codeString))
return RESOLVED;
if ("terminated".equals(codeString))
return TERMINATED;
if (Configuration.isAcceptInvalidEnums())
return null;
else
throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'");
}
public String toCode() {
switch (this) {
case AMENDED:
return "amended";
case APPENDED:
return "appended";
case CANCELLED:
return "cancelled";
case DISPUTED:
return "disputed";
case ENTEREDINERROR:
return "entered-in-error";
case EXECUTABLE:
return "executable";
case EXECUTED:
return "executed";
case NEGOTIABLE:
return "negotiable";
case OFFERED:
return "offered";
case POLICY:
return "policy";
case REJECTED:
return "rejected";
case RENEWED:
return "renewed";
case REVOKED:
return "revoked";
case RESOLVED:
return "resolved";
case TERMINATED:
return "terminated";
case NULL:
return null;
default:
return "?";
}
}
public String getSystem() {
switch (this) {
case AMENDED:
return "http://hl7.org/fhir/contract-publicationstatus";
case APPENDED:
return "http://hl7.org/fhir/contract-publicationstatus";
case CANCELLED:
return "http://hl7.org/fhir/contract-publicationstatus";
case DISPUTED:
return "http://hl7.org/fhir/contract-publicationstatus";
case ENTEREDINERROR:
return "http://hl7.org/fhir/contract-publicationstatus";
case EXECUTABLE:
return "http://hl7.org/fhir/contract-publicationstatus";
case EXECUTED:
return "http://hl7.org/fhir/contract-publicationstatus";
case NEGOTIABLE:
return "http://hl7.org/fhir/contract-publicationstatus";
case OFFERED:
return "http://hl7.org/fhir/contract-publicationstatus";
case POLICY:
return "http://hl7.org/fhir/contract-publicationstatus";
case REJECTED:
return "http://hl7.org/fhir/contract-publicationstatus";
case RENEWED:
return "http://hl7.org/fhir/contract-publicationstatus";
case REVOKED:
return "http://hl7.org/fhir/contract-publicationstatus";
case RESOLVED:
return "http://hl7.org/fhir/contract-publicationstatus";
case TERMINATED:
return "http://hl7.org/fhir/contract-publicationstatus";
case NULL:
return null;
default:
return "?";
}
}
public String getDefinition() {
switch (this) {
case AMENDED:
return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced.";
case APPENDED:
return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced.";
case CANCELLED:
return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted.";
case DISPUTED:
return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended.";
case ENTEREDINERROR:
return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status.";
case EXECUTABLE:
return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active.";
case EXECUTED:
return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed.";
case NEGOTIABLE:
return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held.";
case OFFERED:
return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new.";
case POLICY:
return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended.";
case REJECTED:
return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled.";
case RENEWED:
return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded.";
case REVOKED:
return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified.";
case RESOLVED:
return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated.";
case TERMINATED:
return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted.";
case NULL:
return null;
default:
return "?";
}
}
public String getDisplay() {
switch (this) {
case AMENDED:
return "Amended";
case APPENDED:
return "Appended";
case CANCELLED:
return "Cancelled";
case DISPUTED:
return "Disputed";
case ENTEREDINERROR:
return "Entered in Error";
case EXECUTABLE:
return "Executable";
case EXECUTED:
return "Executed";
case NEGOTIABLE:
return "Negotiable";
case OFFERED:
return "Offered";
case POLICY:
return "Policy";
case REJECTED:
return "Rejected";
case RENEWED:
return "Renewed";
case REVOKED:
return "Revoked";
case RESOLVED:
return "Resolved";
case TERMINATED:
return "Terminated";
case NULL:
return null;
default:
return "?";
}
}
}
public static class ContractPublicationStatusEnumFactory implements EnumFactory {
public ContractPublicationStatus fromCode(String codeString) throws IllegalArgumentException {
if (codeString == null || "".equals(codeString))
if (codeString == null || "".equals(codeString))
return null;
if ("amended".equals(codeString))
return ContractPublicationStatus.AMENDED;
if ("appended".equals(codeString))
return ContractPublicationStatus.APPENDED;
if ("cancelled".equals(codeString))
return ContractPublicationStatus.CANCELLED;
if ("disputed".equals(codeString))
return ContractPublicationStatus.DISPUTED;
if ("entered-in-error".equals(codeString))
return ContractPublicationStatus.ENTEREDINERROR;
if ("executable".equals(codeString))
return ContractPublicationStatus.EXECUTABLE;
if ("executed".equals(codeString))
return ContractPublicationStatus.EXECUTED;
if ("negotiable".equals(codeString))
return ContractPublicationStatus.NEGOTIABLE;
if ("offered".equals(codeString))
return ContractPublicationStatus.OFFERED;
if ("policy".equals(codeString))
return ContractPublicationStatus.POLICY;
if ("rejected".equals(codeString))
return ContractPublicationStatus.REJECTED;
if ("renewed".equals(codeString))
return ContractPublicationStatus.RENEWED;
if ("revoked".equals(codeString))
return ContractPublicationStatus.REVOKED;
if ("resolved".equals(codeString))
return ContractPublicationStatus.RESOLVED;
if ("terminated".equals(codeString))
return ContractPublicationStatus.TERMINATED;
throw new IllegalArgumentException("Unknown ContractPublicationStatus code '" + codeString + "'");
}
public Enumeration fromType(PrimitiveType> code) throws FHIRException {
if (code == null)
return null;
if (code.isEmpty())
return new Enumeration(this, ContractPublicationStatus.NULL, code);
String codeString = code.asStringValue();
if (codeString == null || "".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.NULL, code);
if ("amended".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.AMENDED, code);
if ("appended".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.APPENDED, code);
if ("cancelled".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.CANCELLED, code);
if ("disputed".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.DISPUTED, code);
if ("entered-in-error".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.ENTEREDINERROR, code);
if ("executable".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.EXECUTABLE, code);
if ("executed".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.EXECUTED, code);
if ("negotiable".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.NEGOTIABLE, code);
if ("offered".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.OFFERED, code);
if ("policy".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.POLICY, code);
if ("rejected".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.REJECTED, code);
if ("renewed".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.RENEWED, code);
if ("revoked".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.REVOKED, code);
if ("resolved".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.RESOLVED, code);
if ("terminated".equals(codeString))
return new Enumeration(this, ContractPublicationStatus.TERMINATED, code);
throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'");
}
public String toCode(ContractPublicationStatus code) {
if (code == ContractPublicationStatus.AMENDED)
return "amended";
if (code == ContractPublicationStatus.APPENDED)
return "appended";
if (code == ContractPublicationStatus.CANCELLED)
return "cancelled";
if (code == ContractPublicationStatus.DISPUTED)
return "disputed";
if (code == ContractPublicationStatus.ENTEREDINERROR)
return "entered-in-error";
if (code == ContractPublicationStatus.EXECUTABLE)
return "executable";
if (code == ContractPublicationStatus.EXECUTED)
return "executed";
if (code == ContractPublicationStatus.NEGOTIABLE)
return "negotiable";
if (code == ContractPublicationStatus.OFFERED)
return "offered";
if (code == ContractPublicationStatus.POLICY)
return "policy";
if (code == ContractPublicationStatus.REJECTED)
return "rejected";
if (code == ContractPublicationStatus.RENEWED)
return "renewed";
if (code == ContractPublicationStatus.REVOKED)
return "revoked";
if (code == ContractPublicationStatus.RESOLVED)
return "resolved";
if (code == ContractPublicationStatus.TERMINATED)
return "terminated";
return "?";
}
public String toSystem(ContractPublicationStatus code) {
return code.getSystem();
}
}
@Block()
public static class ContentDefinitionComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Precusory content structure and use, i.e., a boilerplate, template,
* application for a contract such as an insurance policy or benefits under a
* program, e.g., workers compensation.
*/
@Child(name = "type", type = {
CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Content structure and use", formalDefinition = "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-type")
protected CodeableConcept type;
/**
* Detailed Precusory content type.
*/
@Child(name = "subType", type = {
CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Detailed Content Type Definition", formalDefinition = "Detailed Precusory content type.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-subtype")
protected CodeableConcept subType;
/**
* The individual or organization that published the Contract precursor content.
*/
@Child(name = "publisher", type = { Practitioner.class, PractitionerRole.class,
Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Publisher Entity", formalDefinition = "The individual or organization that published the Contract precursor content.")
protected Reference publisher;
/**
* The actual object that is the target of the reference (The individual or
* organization that published the Contract precursor content.)
*/
protected Resource publisherTarget;
/**
* The date (and optionally time) when the contract was published. The date must
* change when the business version changes and it must change if the status
* code changes. In addition, it should change when the substantive content of
* the contract changes.
*/
@Child(name = "publicationDate", type = {
DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "When published", formalDefinition = "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.")
protected DateTimeType publicationDate;
/**
* amended | appended | cancelled | disputed | entered-in-error | executable |
* executed | negotiable | offered | policy | rejected | renewed | revoked |
* resolved | terminated.
*/
@Child(name = "publicationStatus", type = {
CodeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-publicationstatus")
protected Enumeration publicationStatus;
/**
* A copyright statement relating to Contract precursor content. Copyright
* statements are generally legal restrictions on the use and publishing of the
* Contract precursor content.
*/
@Child(name = "copyright", type = {
MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Publication Ownership", formalDefinition = "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.")
protected MarkdownType copyright;
private static final long serialVersionUID = -699592864L;
/**
* Constructor
*/
public ContentDefinitionComponent() {
super();
}
/**
* Constructor
*/
public ContentDefinitionComponent(CodeableConcept type, Enumeration publicationStatus) {
super();
this.type = type;
this.publicationStatus = publicationStatus;
}
/**
* @return {@link #type} (Precusory content structure and use, i.e., a
* boilerplate, template, application for a contract such as an
* insurance policy or benefits under a program, e.g., workers
* compensation.)
*/
public CodeableConcept getType() {
if (this.type == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.type");
else if (Configuration.doAutoCreate())
this.type = new CodeableConcept(); // cc
return this.type;
}
public boolean hasType() {
return this.type != null && !this.type.isEmpty();
}
/**
* @param value {@link #type} (Precusory content structure and use, i.e., a
* boilerplate, template, application for a contract such as an
* insurance policy or benefits under a program, e.g., workers
* compensation.)
*/
public ContentDefinitionComponent setType(CodeableConcept value) {
this.type = value;
return this;
}
/**
* @return {@link #subType} (Detailed Precusory content type.)
*/
public CodeableConcept getSubType() {
if (this.subType == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.subType");
else if (Configuration.doAutoCreate())
this.subType = new CodeableConcept(); // cc
return this.subType;
}
public boolean hasSubType() {
return this.subType != null && !this.subType.isEmpty();
}
/**
* @param value {@link #subType} (Detailed Precusory content type.)
*/
public ContentDefinitionComponent setSubType(CodeableConcept value) {
this.subType = value;
return this;
}
/**
* @return {@link #publisher} (The individual or organization that published the
* Contract precursor content.)
*/
public Reference getPublisher() {
if (this.publisher == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.publisher");
else if (Configuration.doAutoCreate())
this.publisher = new Reference(); // cc
return this.publisher;
}
public boolean hasPublisher() {
return this.publisher != null && !this.publisher.isEmpty();
}
/**
* @param value {@link #publisher} (The individual or organization that
* published the Contract precursor content.)
*/
public ContentDefinitionComponent setPublisher(Reference value) {
this.publisher = value;
return this;
}
/**
* @return {@link #publisher} The actual object that is the target of the
* reference. The reference library doesn't populate this, but you can
* use it to hold the resource if you resolve it. (The individual or
* organization that published the Contract precursor content.)
*/
public Resource getPublisherTarget() {
return this.publisherTarget;
}
/**
* @param value {@link #publisher} The actual object that is the target of the
* reference. The reference library doesn't use these, but you can
* use it to hold the resource if you resolve it. (The individual
* or organization that published the Contract precursor content.)
*/
public ContentDefinitionComponent setPublisherTarget(Resource value) {
this.publisherTarget = value;
return this;
}
/**
* @return {@link #publicationDate} (The date (and optionally time) when the
* contract was published. The date must change when the business
* version changes and it must change if the status code changes. In
* addition, it should change when the substantive content of the
* contract changes.). This is the underlying object with id, value and
* extensions. The accessor "getPublicationDate" gives direct access to
* the value
*/
public DateTimeType getPublicationDateElement() {
if (this.publicationDate == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationDate");
else if (Configuration.doAutoCreate())
this.publicationDate = new DateTimeType(); // bb
return this.publicationDate;
}
public boolean hasPublicationDateElement() {
return this.publicationDate != null && !this.publicationDate.isEmpty();
}
public boolean hasPublicationDate() {
return this.publicationDate != null && !this.publicationDate.isEmpty();
}
/**
* @param value {@link #publicationDate} (The date (and optionally time) when
* the contract was published. The date must change when the
* business version changes and it must change if the status code
* changes. In addition, it should change when the substantive
* content of the contract changes.). This is the underlying object
* with id, value and extensions. The accessor "getPublicationDate"
* gives direct access to the value
*/
public ContentDefinitionComponent setPublicationDateElement(DateTimeType value) {
this.publicationDate = value;
return this;
}
/**
* @return The date (and optionally time) when the contract was published. The
* date must change when the business version changes and it must change
* if the status code changes. In addition, it should change when the
* substantive content of the contract changes.
*/
public Date getPublicationDate() {
return this.publicationDate == null ? null : this.publicationDate.getValue();
}
/**
* @param value The date (and optionally time) when the contract was published.
* The date must change when the business version changes and it
* must change if the status code changes. In addition, it should
* change when the substantive content of the contract changes.
*/
public ContentDefinitionComponent setPublicationDate(Date value) {
if (value == null)
this.publicationDate = null;
else {
if (this.publicationDate == null)
this.publicationDate = new DateTimeType();
this.publicationDate.setValue(value);
}
return this;
}
/**
* @return {@link #publicationStatus} (amended | appended | cancelled | disputed
* | entered-in-error | executable | executed | negotiable | offered |
* policy | rejected | renewed | revoked | resolved | terminated.). This
* is the underlying object with id, value and extensions. The accessor
* "getPublicationStatus" gives direct access to the value
*/
public Enumeration getPublicationStatusElement() {
if (this.publicationStatus == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationStatus");
else if (Configuration.doAutoCreate())
this.publicationStatus = new Enumeration(
new ContractPublicationStatusEnumFactory()); // bb
return this.publicationStatus;
}
public boolean hasPublicationStatusElement() {
return this.publicationStatus != null && !this.publicationStatus.isEmpty();
}
public boolean hasPublicationStatus() {
return this.publicationStatus != null && !this.publicationStatus.isEmpty();
}
/**
* @param value {@link #publicationStatus} (amended | appended | cancelled |
* disputed | entered-in-error | executable | executed | negotiable
* | offered | policy | rejected | renewed | revoked | resolved |
* terminated.). This is the underlying object with id, value and
* extensions. The accessor "getPublicationStatus" gives direct
* access to the value
*/
public ContentDefinitionComponent setPublicationStatusElement(Enumeration value) {
this.publicationStatus = value;
return this;
}
/**
* @return amended | appended | cancelled | disputed | entered-in-error |
* executable | executed | negotiable | offered | policy | rejected |
* renewed | revoked | resolved | terminated.
*/
public ContractPublicationStatus getPublicationStatus() {
return this.publicationStatus == null ? null : this.publicationStatus.getValue();
}
/**
* @param value amended | appended | cancelled | disputed | entered-in-error |
* executable | executed | negotiable | offered | policy | rejected
* | renewed | revoked | resolved | terminated.
*/
public ContentDefinitionComponent setPublicationStatus(ContractPublicationStatus value) {
if (this.publicationStatus == null)
this.publicationStatus = new Enumeration(new ContractPublicationStatusEnumFactory());
this.publicationStatus.setValue(value);
return this;
}
/**
* @return {@link #copyright} (A copyright statement relating to Contract
* precursor content. Copyright statements are generally legal
* restrictions on the use and publishing of the Contract precursor
* content.). This is the underlying object with id, value and
* extensions. The accessor "getCopyright" gives direct access to the
* value
*/
public MarkdownType getCopyrightElement() {
if (this.copyright == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContentDefinitionComponent.copyright");
else if (Configuration.doAutoCreate())
this.copyright = new MarkdownType(); // bb
return this.copyright;
}
public boolean hasCopyrightElement() {
return this.copyright != null && !this.copyright.isEmpty();
}
public boolean hasCopyright() {
return this.copyright != null && !this.copyright.isEmpty();
}
/**
* @param value {@link #copyright} (A copyright statement relating to Contract
* precursor content. Copyright statements are generally legal
* restrictions on the use and publishing of the Contract precursor
* content.). This is the underlying object with id, value and
* extensions. The accessor "getCopyright" gives direct access to
* the value
*/
public ContentDefinitionComponent setCopyrightElement(MarkdownType value) {
this.copyright = value;
return this;
}
/**
* @return A copyright statement relating to Contract precursor content.
* Copyright statements are generally legal restrictions on the use and
* publishing of the Contract precursor content.
*/
public String getCopyright() {
return this.copyright == null ? null : this.copyright.getValue();
}
/**
* @param value A copyright statement relating to Contract precursor content.
* Copyright statements are generally legal restrictions on the use
* and publishing of the Contract precursor content.
*/
public ContentDefinitionComponent setCopyright(String value) {
if (value == null)
this.copyright = null;
else {
if (this.copyright == null)
this.copyright = new MarkdownType();
this.copyright.setValue(value);
}
return this;
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("type", "CodeableConcept",
"Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.",
0, 1, type));
children.add(new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, subType));
children.add(new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)",
"The individual or organization that published the Contract precursor content.", 0, 1, publisher));
children.add(new Property("publicationDate", "dateTime",
"The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.",
0, 1, publicationDate));
children.add(new Property("publicationStatus", "code",
"amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.",
0, 1, publicationStatus));
children.add(new Property("copyright", "markdown",
"A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.",
0, 1, copyright));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case 3575610:
/* type */ return new Property("type", "CodeableConcept",
"Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.",
0, 1, type);
case -1868521062:
/* subType */ return new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1,
subType);
case 1447404028:
/* publisher */ return new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)",
"The individual or organization that published the Contract precursor content.", 0, 1, publisher);
case 1470566394:
/* publicationDate */ return new Property("publicationDate", "dateTime",
"The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.",
0, 1, publicationDate);
case 616500542:
/* publicationStatus */ return new Property("publicationStatus", "code",
"amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.",
0, 1, publicationStatus);
case 1522889671:
/* copyright */ return new Property("copyright", "markdown",
"A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.",
0, 1, copyright);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case 3575610:
/* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept
case -1868521062:
/* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept
case 1447404028:
/* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // Reference
case 1470566394:
/* publicationDate */ return this.publicationDate == null ? new Base[0] : new Base[] { this.publicationDate }; // DateTimeType
case 616500542:
/* publicationStatus */ return this.publicationStatus == null ? new Base[0]
: new Base[] { this.publicationStatus }; // Enumeration
case 1522889671:
/* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case 3575610: // type
this.type = castToCodeableConcept(value); // CodeableConcept
return value;
case -1868521062: // subType
this.subType = castToCodeableConcept(value); // CodeableConcept
return value;
case 1447404028: // publisher
this.publisher = castToReference(value); // Reference
return value;
case 1470566394: // publicationDate
this.publicationDate = castToDateTime(value); // DateTimeType
return value;
case 616500542: // publicationStatus
value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value));
this.publicationStatus = (Enumeration) value; // Enumeration
return value;
case 1522889671: // copyright
this.copyright = castToMarkdown(value); // MarkdownType
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("type")) {
this.type = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("subType")) {
this.subType = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("publisher")) {
this.publisher = castToReference(value); // Reference
} else if (name.equals("publicationDate")) {
this.publicationDate = castToDateTime(value); // DateTimeType
} else if (name.equals("publicationStatus")) {
value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value));
this.publicationStatus = (Enumeration) value; // Enumeration
} else if (name.equals("copyright")) {
this.copyright = castToMarkdown(value); // MarkdownType
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("type")) {
this.type = null;
} else if (name.equals("subType")) {
this.subType = null;
} else if (name.equals("publisher")) {
this.publisher = null;
} else if (name.equals("publicationDate")) {
this.publicationDate = null;
} else if (name.equals("publicationStatus")) {
this.publicationStatus = null;
} else if (name.equals("copyright")) {
this.copyright = null;
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case 3575610:
return getType();
case -1868521062:
return getSubType();
case 1447404028:
return getPublisher();
case 1470566394:
return getPublicationDateElement();
case 616500542:
return getPublicationStatusElement();
case 1522889671:
return getCopyrightElement();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case 3575610:
/* type */ return new String[] { "CodeableConcept" };
case -1868521062:
/* subType */ return new String[] { "CodeableConcept" };
case 1447404028:
/* publisher */ return new String[] { "Reference" };
case 1470566394:
/* publicationDate */ return new String[] { "dateTime" };
case 616500542:
/* publicationStatus */ return new String[] { "code" };
case 1522889671:
/* copyright */ return new String[] { "markdown" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("type")) {
this.type = new CodeableConcept();
return this.type;
} else if (name.equals("subType")) {
this.subType = new CodeableConcept();
return this.subType;
} else if (name.equals("publisher")) {
this.publisher = new Reference();
return this.publisher;
} else if (name.equals("publicationDate")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationDate");
} else if (name.equals("publicationStatus")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationStatus");
} else if (name.equals("copyright")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.copyright");
} else
return super.addChild(name);
}
public ContentDefinitionComponent copy() {
ContentDefinitionComponent dst = new ContentDefinitionComponent();
copyValues(dst);
return dst;
}
public void copyValues(ContentDefinitionComponent dst) {
super.copyValues(dst);
dst.type = type == null ? null : type.copy();
dst.subType = subType == null ? null : subType.copy();
dst.publisher = publisher == null ? null : publisher.copy();
dst.publicationDate = publicationDate == null ? null : publicationDate.copy();
dst.publicationStatus = publicationStatus == null ? null : publicationStatus.copy();
dst.copyright = copyright == null ? null : copyright.copy();
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof ContentDefinitionComponent))
return false;
ContentDefinitionComponent o = (ContentDefinitionComponent) other_;
return compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true)
&& compareDeep(publisher, o.publisher, true) && compareDeep(publicationDate, o.publicationDate, true)
&& compareDeep(publicationStatus, o.publicationStatus, true) && compareDeep(copyright, o.copyright, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof ContentDefinitionComponent))
return false;
ContentDefinitionComponent o = (ContentDefinitionComponent) other_;
return compareValues(publicationDate, o.publicationDate, true)
&& compareValues(publicationStatus, o.publicationStatus, true) && compareValues(copyright, o.copyright, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subType, publisher, publicationDate,
publicationStatus, copyright);
}
public String fhirType() {
return "Contract.contentDefinition";
}
}
@Block()
public static class TermComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Unique identifier for this particular Contract Provision.
*/
@Child(name = "identifier", type = {
Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true)
@Description(shortDefinition = "Contract Term Number", formalDefinition = "Unique identifier for this particular Contract Provision.")
protected Identifier identifier;
/**
* When this Contract Provision was issued.
*/
@Child(name = "issued", type = {
DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true)
@Description(shortDefinition = "Contract Term Issue Date Time", formalDefinition = "When this Contract Provision was issued.")
protected DateTimeType issued;
/**
* Relevant time or time-period when this Contract Provision is applicable.
*/
@Child(name = "applies", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true)
@Description(shortDefinition = "Contract Term Effective Time", formalDefinition = "Relevant time or time-period when this Contract Provision is applicable.")
protected Period applies;
/**
* The entity that the term applies to.
*/
@Child(name = "topic", type = { CodeableConcept.class,
Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Term Concern", formalDefinition = "The entity that the term applies to.")
protected Type topic;
/**
* A legal clause or condition contained within a contract that requires one or
* both parties to perform a particular requirement by some specified time or
* prevents one or both parties from performing a particular requirement by some
* specified time.
*/
@Child(name = "type", type = {
CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Term Type or Form", formalDefinition = "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type")
protected CodeableConcept type;
/**
* A specialized legal clause or condition based on overarching contract type.
*/
@Child(name = "subType", type = {
CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Term Type specific classification", formalDefinition = "A specialized legal clause or condition based on overarching contract type.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-subtype")
protected CodeableConcept subType;
/**
* Statement of a provision in a policy or a contract.
*/
@Child(name = "text", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true)
@Description(shortDefinition = "Term Statement", formalDefinition = "Statement of a provision in a policy or a contract.")
protected StringType text;
/**
* Security labels that protect the handling of information about the term and
* its elements, which may be specifically identified..
*/
@Child(name = "securityLabel", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Protection for the Term", formalDefinition = "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..")
protected List securityLabel;
/**
* The matter of concern in the context of this provision of the agrement.
*/
@Child(name = "offer", type = {}, order = 9, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Context of the Contract term", formalDefinition = "The matter of concern in the context of this provision of the agrement.")
protected ContractOfferComponent offer;
/**
* Contract Term Asset List.
*/
@Child(name = "asset", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Contract Term Asset List", formalDefinition = "Contract Term Asset List.")
protected List asset;
/**
* An actor taking a role in an activity for which it can be assigned some
* degree of responsibility for the activity taking place.
*/
@Child(name = "action", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Entity being ascribed responsibility", formalDefinition = "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.")
protected List action;
/**
* Nested group of Contract Provisions.
*/
@Child(name = "group", type = {
TermComponent.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Nested Contract Term Group", formalDefinition = "Nested group of Contract Provisions.")
protected List group;
private static final long serialVersionUID = -460907186L;
/**
* Constructor
*/
public TermComponent() {
super();
}
/**
* Constructor
*/
public TermComponent(ContractOfferComponent offer) {
super();
this.offer = offer;
}
/**
* @return {@link #identifier} (Unique identifier for this particular Contract
* Provision.)
*/
public Identifier getIdentifier() {
if (this.identifier == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.identifier");
else if (Configuration.doAutoCreate())
this.identifier = new Identifier(); // cc
return this.identifier;
}
public boolean hasIdentifier() {
return this.identifier != null && !this.identifier.isEmpty();
}
/**
* @param value {@link #identifier} (Unique identifier for this particular
* Contract Provision.)
*/
public TermComponent setIdentifier(Identifier value) {
this.identifier = value;
return this;
}
/**
* @return {@link #issued} (When this Contract Provision was issued.). This is
* the underlying object with id, value and extensions. The accessor
* "getIssued" gives direct access to the value
*/
public DateTimeType getIssuedElement() {
if (this.issued == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.issued");
else if (Configuration.doAutoCreate())
this.issued = new DateTimeType(); // bb
return this.issued;
}
public boolean hasIssuedElement() {
return this.issued != null && !this.issued.isEmpty();
}
public boolean hasIssued() {
return this.issued != null && !this.issued.isEmpty();
}
/**
* @param value {@link #issued} (When this Contract Provision was issued.). This
* is the underlying object with id, value and extensions. The
* accessor "getIssued" gives direct access to the value
*/
public TermComponent setIssuedElement(DateTimeType value) {
this.issued = value;
return this;
}
/**
* @return When this Contract Provision was issued.
*/
public Date getIssued() {
return this.issued == null ? null : this.issued.getValue();
}
/**
* @param value When this Contract Provision was issued.
*/
public TermComponent setIssued(Date value) {
if (value == null)
this.issued = null;
else {
if (this.issued == null)
this.issued = new DateTimeType();
this.issued.setValue(value);
}
return this;
}
/**
* @return {@link #applies} (Relevant time or time-period when this Contract
* Provision is applicable.)
*/
public Period getApplies() {
if (this.applies == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.applies");
else if (Configuration.doAutoCreate())
this.applies = new Period(); // cc
return this.applies;
}
public boolean hasApplies() {
return this.applies != null && !this.applies.isEmpty();
}
/**
* @param value {@link #applies} (Relevant time or time-period when this
* Contract Provision is applicable.)
*/
public TermComponent setApplies(Period value) {
this.applies = value;
return this;
}
/**
* @return {@link #topic} (The entity that the term applies to.)
*/
public Type getTopic() {
return this.topic;
}
/**
* @return {@link #topic} (The entity that the term applies to.)
*/
public CodeableConcept getTopicCodeableConcept() throws FHIRException {
if (this.topic == null)
this.topic = new CodeableConcept();
if (!(this.topic instanceof CodeableConcept))
throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "
+ this.topic.getClass().getName() + " was encountered");
return (CodeableConcept) this.topic;
}
public boolean hasTopicCodeableConcept() {
return this != null && this.topic instanceof CodeableConcept;
}
/**
* @return {@link #topic} (The entity that the term applies to.)
*/
public Reference getTopicReference() throws FHIRException {
if (this.topic == null)
this.topic = new Reference();
if (!(this.topic instanceof Reference))
throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.topic.getClass().getName()
+ " was encountered");
return (Reference) this.topic;
}
public boolean hasTopicReference() {
return this != null && this.topic instanceof Reference;
}
public boolean hasTopic() {
return this.topic != null && !this.topic.isEmpty();
}
/**
* @param value {@link #topic} (The entity that the term applies to.)
*/
public TermComponent setTopic(Type value) {
if (value != null && !(value instanceof CodeableConcept || value instanceof Reference))
throw new Error("Not the right type for Contract.term.topic[x]: " + value.fhirType());
this.topic = value;
return this;
}
/**
* @return {@link #type} (A legal clause or condition contained within a
* contract that requires one or both parties to perform a particular
* requirement by some specified time or prevents one or both parties
* from performing a particular requirement by some specified time.)
*/
public CodeableConcept getType() {
if (this.type == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.type");
else if (Configuration.doAutoCreate())
this.type = new CodeableConcept(); // cc
return this.type;
}
public boolean hasType() {
return this.type != null && !this.type.isEmpty();
}
/**
* @param value {@link #type} (A legal clause or condition contained within a
* contract that requires one or both parties to perform a
* particular requirement by some specified time or prevents one or
* both parties from performing a particular requirement by some
* specified time.)
*/
public TermComponent setType(CodeableConcept value) {
this.type = value;
return this;
}
/**
* @return {@link #subType} (A specialized legal clause or condition based on
* overarching contract type.)
*/
public CodeableConcept getSubType() {
if (this.subType == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.subType");
else if (Configuration.doAutoCreate())
this.subType = new CodeableConcept(); // cc
return this.subType;
}
public boolean hasSubType() {
return this.subType != null && !this.subType.isEmpty();
}
/**
* @param value {@link #subType} (A specialized legal clause or condition based
* on overarching contract type.)
*/
public TermComponent setSubType(CodeableConcept value) {
this.subType = value;
return this;
}
/**
* @return {@link #text} (Statement of a provision in a policy or a contract.).
* This is the underlying object with id, value and extensions. The
* accessor "getText" gives direct access to the value
*/
public StringType getTextElement() {
if (this.text == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.text");
else if (Configuration.doAutoCreate())
this.text = new StringType(); // bb
return this.text;
}
public boolean hasTextElement() {
return this.text != null && !this.text.isEmpty();
}
public boolean hasText() {
return this.text != null && !this.text.isEmpty();
}
/**
* @param value {@link #text} (Statement of a provision in a policy or a
* contract.). This is the underlying object with id, value and
* extensions. The accessor "getText" gives direct access to the
* value
*/
public TermComponent setTextElement(StringType value) {
this.text = value;
return this;
}
/**
* @return Statement of a provision in a policy or a contract.
*/
public String getText() {
return this.text == null ? null : this.text.getValue();
}
/**
* @param value Statement of a provision in a policy or a contract.
*/
public TermComponent setText(String value) {
if (Utilities.noString(value))
this.text = null;
else {
if (this.text == null)
this.text = new StringType();
this.text.setValue(value);
}
return this;
}
/**
* @return {@link #securityLabel} (Security labels that protect the handling of
* information about the term and its elements, which may be
* specifically identified..)
*/
public List getSecurityLabel() {
if (this.securityLabel == null)
this.securityLabel = new ArrayList();
return this.securityLabel;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public TermComponent setSecurityLabel(List theSecurityLabel) {
this.securityLabel = theSecurityLabel;
return this;
}
public boolean hasSecurityLabel() {
if (this.securityLabel == null)
return false;
for (SecurityLabelComponent item : this.securityLabel)
if (!item.isEmpty())
return true;
return false;
}
public SecurityLabelComponent addSecurityLabel() { // 3
SecurityLabelComponent t = new SecurityLabelComponent();
if (this.securityLabel == null)
this.securityLabel = new ArrayList();
this.securityLabel.add(t);
return t;
}
public TermComponent addSecurityLabel(SecurityLabelComponent t) { // 3
if (t == null)
return this;
if (this.securityLabel == null)
this.securityLabel = new ArrayList();
this.securityLabel.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #securityLabel},
* creating it if it does not already exist
*/
public SecurityLabelComponent getSecurityLabelFirstRep() {
if (getSecurityLabel().isEmpty()) {
addSecurityLabel();
}
return getSecurityLabel().get(0);
}
/**
* @return {@link #offer} (The matter of concern in the context of this
* provision of the agrement.)
*/
public ContractOfferComponent getOffer() {
if (this.offer == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create TermComponent.offer");
else if (Configuration.doAutoCreate())
this.offer = new ContractOfferComponent(); // cc
return this.offer;
}
public boolean hasOffer() {
return this.offer != null && !this.offer.isEmpty();
}
/**
* @param value {@link #offer} (The matter of concern in the context of this
* provision of the agrement.)
*/
public TermComponent setOffer(ContractOfferComponent value) {
this.offer = value;
return this;
}
/**
* @return {@link #asset} (Contract Term Asset List.)
*/
public List getAsset() {
if (this.asset == null)
this.asset = new ArrayList();
return this.asset;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public TermComponent setAsset(List theAsset) {
this.asset = theAsset;
return this;
}
public boolean hasAsset() {
if (this.asset == null)
return false;
for (ContractAssetComponent item : this.asset)
if (!item.isEmpty())
return true;
return false;
}
public ContractAssetComponent addAsset() { // 3
ContractAssetComponent t = new ContractAssetComponent();
if (this.asset == null)
this.asset = new ArrayList();
this.asset.add(t);
return t;
}
public TermComponent addAsset(ContractAssetComponent t) { // 3
if (t == null)
return this;
if (this.asset == null)
this.asset = new ArrayList();
this.asset.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #asset}, creating it
* if it does not already exist
*/
public ContractAssetComponent getAssetFirstRep() {
if (getAsset().isEmpty()) {
addAsset();
}
return getAsset().get(0);
}
/**
* @return {@link #action} (An actor taking a role in an activity for which it
* can be assigned some degree of responsibility for the activity taking
* place.)
*/
public List getAction() {
if (this.action == null)
this.action = new ArrayList();
return this.action;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public TermComponent setAction(List theAction) {
this.action = theAction;
return this;
}
public boolean hasAction() {
if (this.action == null)
return false;
for (ActionComponent item : this.action)
if (!item.isEmpty())
return true;
return false;
}
public ActionComponent addAction() { // 3
ActionComponent t = new ActionComponent();
if (this.action == null)
this.action = new ArrayList();
this.action.add(t);
return t;
}
public TermComponent addAction(ActionComponent t) { // 3
if (t == null)
return this;
if (this.action == null)
this.action = new ArrayList();
this.action.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #action}, creating it
* if it does not already exist
*/
public ActionComponent getActionFirstRep() {
if (getAction().isEmpty()) {
addAction();
}
return getAction().get(0);
}
/**
* @return {@link #group} (Nested group of Contract Provisions.)
*/
public List getGroup() {
if (this.group == null)
this.group = new ArrayList();
return this.group;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public TermComponent setGroup(List theGroup) {
this.group = theGroup;
return this;
}
public boolean hasGroup() {
if (this.group == null)
return false;
for (TermComponent item : this.group)
if (!item.isEmpty())
return true;
return false;
}
public TermComponent addGroup() { // 3
TermComponent t = new TermComponent();
if (this.group == null)
this.group = new ArrayList();
this.group.add(t);
return t;
}
public TermComponent addGroup(TermComponent t) { // 3
if (t == null)
return this;
if (this.group == null)
this.group = new ArrayList();
this.group.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #group}, creating it
* if it does not already exist
*/
public TermComponent getGroupFirstRep() {
if (getGroup().isEmpty()) {
addGroup();
}
return getGroup().get(0);
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.",
0, 1, identifier));
children.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued));
children.add(new Property("applies", "Period",
"Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies));
children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0,
1, topic));
children.add(new Property("type", "CodeableConcept",
"A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.",
0, 1, type));
children.add(new Property("subType", "CodeableConcept",
"A specialized legal clause or condition based on overarching contract type.", 0, 1, subType));
children.add(new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, text));
children.add(new Property("securityLabel", "",
"Security labels that protect the handling of information about the term and its elements, which may be specifically identified..",
0, java.lang.Integer.MAX_VALUE, securityLabel));
children.add(new Property("offer", "", "The matter of concern in the context of this provision of the agrement.",
0, 1, offer));
children.add(new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, asset));
children.add(new Property("action", "",
"An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.",
0, java.lang.Integer.MAX_VALUE, action));
children.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0,
java.lang.Integer.MAX_VALUE, group));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -1618432855:
/* identifier */ return new Property("identifier", "Identifier",
"Unique identifier for this particular Contract Provision.", 0, 1, identifier);
case -1179159893:
/* issued */ return new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1,
issued);
case -793235316:
/* applies */ return new Property("applies", "Period",
"Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies);
case -957295375:
/* topic[x] */ return new Property("topic[x]", "CodeableConcept|Reference(Any)",
"The entity that the term applies to.", 0, 1, topic);
case 110546223:
/* topic */ return new Property("topic[x]", "CodeableConcept|Reference(Any)",
"The entity that the term applies to.", 0, 1, topic);
case 777778802:
/* topicCodeableConcept */ return new Property("topic[x]", "CodeableConcept|Reference(Any)",
"The entity that the term applies to.", 0, 1, topic);
case -343345444:
/* topicReference */ return new Property("topic[x]", "CodeableConcept|Reference(Any)",
"The entity that the term applies to.", 0, 1, topic);
case 3575610:
/* type */ return new Property("type", "CodeableConcept",
"A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.",
0, 1, type);
case -1868521062:
/* subType */ return new Property("subType", "CodeableConcept",
"A specialized legal clause or condition based on overarching contract type.", 0, 1, subType);
case 3556653:
/* text */ return new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1,
text);
case -722296940:
/* securityLabel */ return new Property("securityLabel", "",
"Security labels that protect the handling of information about the term and its elements, which may be specifically identified..",
0, java.lang.Integer.MAX_VALUE, securityLabel);
case 105650780:
/* offer */ return new Property("offer", "",
"The matter of concern in the context of this provision of the agrement.", 0, 1, offer);
case 93121264:
/* asset */ return new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE,
asset);
case -1422950858:
/* action */ return new Property("action", "",
"An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.",
0, java.lang.Integer.MAX_VALUE, action);
case 98629247:
/* group */ return new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0,
java.lang.Integer.MAX_VALUE, group);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case -1618432855:
/* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier
case -1179159893:
/* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType
case -793235316:
/* applies */ return this.applies == null ? new Base[0] : new Base[] { this.applies }; // Period
case 110546223:
/* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Type
case 3575610:
/* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept
case -1868521062:
/* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept
case 3556653:
/* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType
case -722296940:
/* securityLabel */ return this.securityLabel == null ? new Base[0]
: this.securityLabel.toArray(new Base[this.securityLabel.size()]); // SecurityLabelComponent
case 105650780:
/* offer */ return this.offer == null ? new Base[0] : new Base[] { this.offer }; // ContractOfferComponent
case 93121264:
/* asset */ return this.asset == null ? new Base[0] : this.asset.toArray(new Base[this.asset.size()]); // ContractAssetComponent
case -1422950858:
/* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // ActionComponent
case 98629247:
/* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // TermComponent
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case -1618432855: // identifier
this.identifier = castToIdentifier(value); // Identifier
return value;
case -1179159893: // issued
this.issued = castToDateTime(value); // DateTimeType
return value;
case -793235316: // applies
this.applies = castToPeriod(value); // Period
return value;
case 110546223: // topic
this.topic = castToType(value); // Type
return value;
case 3575610: // type
this.type = castToCodeableConcept(value); // CodeableConcept
return value;
case -1868521062: // subType
this.subType = castToCodeableConcept(value); // CodeableConcept
return value;
case 3556653: // text
this.text = castToString(value); // StringType
return value;
case -722296940: // securityLabel
this.getSecurityLabel().add((SecurityLabelComponent) value); // SecurityLabelComponent
return value;
case 105650780: // offer
this.offer = (ContractOfferComponent) value; // ContractOfferComponent
return value;
case 93121264: // asset
this.getAsset().add((ContractAssetComponent) value); // ContractAssetComponent
return value;
case -1422950858: // action
this.getAction().add((ActionComponent) value); // ActionComponent
return value;
case 98629247: // group
this.getGroup().add((TermComponent) value); // TermComponent
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("identifier")) {
this.identifier = castToIdentifier(value); // Identifier
} else if (name.equals("issued")) {
this.issued = castToDateTime(value); // DateTimeType
} else if (name.equals("applies")) {
this.applies = castToPeriod(value); // Period
} else if (name.equals("topic[x]")) {
this.topic = castToType(value); // Type
} else if (name.equals("type")) {
this.type = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("subType")) {
this.subType = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("text")) {
this.text = castToString(value); // StringType
} else if (name.equals("securityLabel")) {
this.getSecurityLabel().add((SecurityLabelComponent) value);
} else if (name.equals("offer")) {
this.offer = (ContractOfferComponent) value; // ContractOfferComponent
} else if (name.equals("asset")) {
this.getAsset().add((ContractAssetComponent) value);
} else if (name.equals("action")) {
this.getAction().add((ActionComponent) value);
} else if (name.equals("group")) {
this.getGroup().add((TermComponent) value);
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("identifier")) {
this.identifier = null;
} else if (name.equals("issued")) {
this.issued = null;
} else if (name.equals("applies")) {
this.applies = null;
} else if (name.equals("topic[x]")) {
this.topic = null;
} else if (name.equals("type")) {
this.type = null;
} else if (name.equals("subType")) {
this.subType = null;
} else if (name.equals("text")) {
this.text = null;
} else if (name.equals("securityLabel")) {
this.getSecurityLabel().remove((SecurityLabelComponent) value);
} else if (name.equals("offer")) {
this.offer = (ContractOfferComponent) value; // ContractOfferComponent
} else if (name.equals("asset")) {
this.getAsset().remove((ContractAssetComponent) value);
} else if (name.equals("action")) {
this.getAction().remove((ActionComponent) value);
} else if (name.equals("group")) {
this.getGroup().remove((TermComponent) value);
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1618432855:
return getIdentifier();
case -1179159893:
return getIssuedElement();
case -793235316:
return getApplies();
case -957295375:
return getTopic();
case 110546223:
return getTopic();
case 3575610:
return getType();
case -1868521062:
return getSubType();
case 3556653:
return getTextElement();
case -722296940:
return addSecurityLabel();
case 105650780:
return getOffer();
case 93121264:
return addAsset();
case -1422950858:
return addAction();
case 98629247:
return addGroup();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1618432855:
/* identifier */ return new String[] { "Identifier" };
case -1179159893:
/* issued */ return new String[] { "dateTime" };
case -793235316:
/* applies */ return new String[] { "Period" };
case 110546223:
/* topic */ return new String[] { "CodeableConcept", "Reference" };
case 3575610:
/* type */ return new String[] { "CodeableConcept" };
case -1868521062:
/* subType */ return new String[] { "CodeableConcept" };
case 3556653:
/* text */ return new String[] { "string" };
case -722296940:
/* securityLabel */ return new String[] {};
case 105650780:
/* offer */ return new String[] {};
case 93121264:
/* asset */ return new String[] {};
case -1422950858:
/* action */ return new String[] {};
case 98629247:
/* group */ return new String[] { "@Contract.term" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("identifier")) {
this.identifier = new Identifier();
return this.identifier;
} else if (name.equals("issued")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.issued");
} else if (name.equals("applies")) {
this.applies = new Period();
return this.applies;
} else if (name.equals("topicCodeableConcept")) {
this.topic = new CodeableConcept();
return this.topic;
} else if (name.equals("topicReference")) {
this.topic = new Reference();
return this.topic;
} else if (name.equals("type")) {
this.type = new CodeableConcept();
return this.type;
} else if (name.equals("subType")) {
this.subType = new CodeableConcept();
return this.subType;
} else if (name.equals("text")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.text");
} else if (name.equals("securityLabel")) {
return addSecurityLabel();
} else if (name.equals("offer")) {
this.offer = new ContractOfferComponent();
return this.offer;
} else if (name.equals("asset")) {
return addAsset();
} else if (name.equals("action")) {
return addAction();
} else if (name.equals("group")) {
return addGroup();
} else
return super.addChild(name);
}
public TermComponent copy() {
TermComponent dst = new TermComponent();
copyValues(dst);
return dst;
}
public void copyValues(TermComponent dst) {
super.copyValues(dst);
dst.identifier = identifier == null ? null : identifier.copy();
dst.issued = issued == null ? null : issued.copy();
dst.applies = applies == null ? null : applies.copy();
dst.topic = topic == null ? null : topic.copy();
dst.type = type == null ? null : type.copy();
dst.subType = subType == null ? null : subType.copy();
dst.text = text == null ? null : text.copy();
if (securityLabel != null) {
dst.securityLabel = new ArrayList();
for (SecurityLabelComponent i : securityLabel)
dst.securityLabel.add(i.copy());
}
;
dst.offer = offer == null ? null : offer.copy();
if (asset != null) {
dst.asset = new ArrayList();
for (ContractAssetComponent i : asset)
dst.asset.add(i.copy());
}
;
if (action != null) {
dst.action = new ArrayList();
for (ActionComponent i : action)
dst.action.add(i.copy());
}
;
if (group != null) {
dst.group = new ArrayList();
for (TermComponent i : group)
dst.group.add(i.copy());
}
;
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof TermComponent))
return false;
TermComponent o = (TermComponent) other_;
return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true)
&& compareDeep(applies, o.applies, true) && compareDeep(topic, o.topic, true)
&& compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(text, o.text, true)
&& compareDeep(securityLabel, o.securityLabel, true) && compareDeep(offer, o.offer, true)
&& compareDeep(asset, o.asset, true) && compareDeep(action, o.action, true)
&& compareDeep(group, o.group, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof TermComponent))
return false;
TermComponent o = (TermComponent) other_;
return compareValues(issued, o.issued, true) && compareValues(text, o.text, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, issued, applies, topic, type, subType,
text, securityLabel, offer, asset, action, group);
}
public String fhirType() {
return "Contract.term";
}
}
@Block()
public static class SecurityLabelComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Number used to link this term or term element to the applicable Security
* Label.
*/
@Child(name = "number", type = {
UnsignedIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Link to Security Labels", formalDefinition = "Number used to link this term or term element to the applicable Security Label.")
protected List number;
/**
* Security label privacy tag that species the level of confidentiality
* protection required for this term and/or term elements.
*/
@Child(name = "classification", type = {
Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Confidentiality Protection", formalDefinition = "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-classification")
protected Coding classification;
/**
* Security label privacy tag that species the applicable privacy and security
* policies governing this term and/or term elements.
*/
@Child(name = "category", type = {
Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Applicable Policy", formalDefinition = "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-category")
protected List category;
/**
* Security label privacy tag that species the manner in which term and/or term
* elements are to be protected.
*/
@Child(name = "control", type = {
Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Handling Instructions", formalDefinition = "Security label privacy tag that species the manner in which term and/or term elements are to be protected.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-control")
protected List control;
private static final long serialVersionUID = 788281758L;
/**
* Constructor
*/
public SecurityLabelComponent() {
super();
}
/**
* Constructor
*/
public SecurityLabelComponent(Coding classification) {
super();
this.classification = classification;
}
/**
* @return {@link #number} (Number used to link this term or term element to the
* applicable Security Label.)
*/
public List getNumber() {
if (this.number == null)
this.number = new ArrayList();
return this.number;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public SecurityLabelComponent setNumber(List theNumber) {
this.number = theNumber;
return this;
}
public boolean hasNumber() {
if (this.number == null)
return false;
for (UnsignedIntType item : this.number)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #number} (Number used to link this term or term element to the
* applicable Security Label.)
*/
public UnsignedIntType addNumberElement() {// 2
UnsignedIntType t = new UnsignedIntType();
if (this.number == null)
this.number = new ArrayList();
this.number.add(t);
return t;
}
/**
* @param value {@link #number} (Number used to link this term or term element
* to the applicable Security Label.)
*/
public SecurityLabelComponent addNumber(int value) { // 1
UnsignedIntType t = new UnsignedIntType();
t.setValue(value);
if (this.number == null)
this.number = new ArrayList();
this.number.add(t);
return this;
}
/**
* @param value {@link #number} (Number used to link this term or term element
* to the applicable Security Label.)
*/
public boolean hasNumber(int value) {
if (this.number == null)
return false;
for (UnsignedIntType v : this.number)
if (v.getValue().equals(value)) // unsignedInt
return true;
return false;
}
/**
* @return {@link #classification} (Security label privacy tag that species the
* level of confidentiality protection required for this term and/or
* term elements.)
*/
public Coding getClassification() {
if (this.classification == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create SecurityLabelComponent.classification");
else if (Configuration.doAutoCreate())
this.classification = new Coding(); // cc
return this.classification;
}
public boolean hasClassification() {
return this.classification != null && !this.classification.isEmpty();
}
/**
* @param value {@link #classification} (Security label privacy tag that species
* the level of confidentiality protection required for this term
* and/or term elements.)
*/
public SecurityLabelComponent setClassification(Coding value) {
this.classification = value;
return this;
}
/**
* @return {@link #category} (Security label privacy tag that species the
* applicable privacy and security policies governing this term and/or
* term elements.)
*/
public List getCategory() {
if (this.category == null)
this.category = new ArrayList();
return this.category;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public SecurityLabelComponent setCategory(List theCategory) {
this.category = theCategory;
return this;
}
public boolean hasCategory() {
if (this.category == null)
return false;
for (Coding item : this.category)
if (!item.isEmpty())
return true;
return false;
}
public Coding addCategory() { // 3
Coding t = new Coding();
if (this.category == null)
this.category = new ArrayList();
this.category.add(t);
return t;
}
public SecurityLabelComponent addCategory(Coding t) { // 3
if (t == null)
return this;
if (this.category == null)
this.category = new ArrayList();
this.category.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #category}, creating
* it if it does not already exist
*/
public Coding getCategoryFirstRep() {
if (getCategory().isEmpty()) {
addCategory();
}
return getCategory().get(0);
}
/**
* @return {@link #control} (Security label privacy tag that species the manner
* in which term and/or term elements are to be protected.)
*/
public List getControl() {
if (this.control == null)
this.control = new ArrayList();
return this.control;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public SecurityLabelComponent setControl(List theControl) {
this.control = theControl;
return this;
}
public boolean hasControl() {
if (this.control == null)
return false;
for (Coding item : this.control)
if (!item.isEmpty())
return true;
return false;
}
public Coding addControl() { // 3
Coding t = new Coding();
if (this.control == null)
this.control = new ArrayList();
this.control.add(t);
return t;
}
public SecurityLabelComponent addControl(Coding t) { // 3
if (t == null)
return this;
if (this.control == null)
this.control = new ArrayList();
this.control.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #control}, creating it
* if it does not already exist
*/
public Coding getControlFirstRep() {
if (getControl().isEmpty()) {
addControl();
}
return getControl().get(0);
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("number", "unsignedInt",
"Number used to link this term or term element to the applicable Security Label.", 0,
java.lang.Integer.MAX_VALUE, number));
children.add(new Property("classification", "Coding",
"Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.",
0, 1, classification));
children.add(new Property("category", "Coding",
"Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.",
0, java.lang.Integer.MAX_VALUE, category));
children.add(new Property("control", "Coding",
"Security label privacy tag that species the manner in which term and/or term elements are to be protected.",
0, java.lang.Integer.MAX_VALUE, control));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -1034364087:
/* number */ return new Property("number", "unsignedInt",
"Number used to link this term or term element to the applicable Security Label.", 0,
java.lang.Integer.MAX_VALUE, number);
case 382350310:
/* classification */ return new Property("classification", "Coding",
"Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.",
0, 1, classification);
case 50511102:
/* category */ return new Property("category", "Coding",
"Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.",
0, java.lang.Integer.MAX_VALUE, category);
case 951543133:
/* control */ return new Property("control", "Coding",
"Security label privacy tag that species the manner in which term and/or term elements are to be protected.",
0, java.lang.Integer.MAX_VALUE, control);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case -1034364087:
/* number */ return this.number == null ? new Base[0] : this.number.toArray(new Base[this.number.size()]); // UnsignedIntType
case 382350310:
/* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // Coding
case 50511102:
/* category */ return this.category == null ? new Base[0]
: this.category.toArray(new Base[this.category.size()]); // Coding
case 951543133:
/* control */ return this.control == null ? new Base[0] : this.control.toArray(new Base[this.control.size()]); // Coding
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case -1034364087: // number
this.getNumber().add(castToUnsignedInt(value)); // UnsignedIntType
return value;
case 382350310: // classification
this.classification = castToCoding(value); // Coding
return value;
case 50511102: // category
this.getCategory().add(castToCoding(value)); // Coding
return value;
case 951543133: // control
this.getControl().add(castToCoding(value)); // Coding
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("number")) {
this.getNumber().add(castToUnsignedInt(value));
} else if (name.equals("classification")) {
this.classification = castToCoding(value); // Coding
} else if (name.equals("category")) {
this.getCategory().add(castToCoding(value));
} else if (name.equals("control")) {
this.getControl().add(castToCoding(value));
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("number")) {
this.getNumber().remove(castToUnsignedInt(value));
} else if (name.equals("classification")) {
this.classification = null;
} else if (name.equals("category")) {
this.getCategory().remove(castToCoding(value));
} else if (name.equals("control")) {
this.getControl().remove(castToCoding(value));
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1034364087:
return addNumberElement();
case 382350310:
return getClassification();
case 50511102:
return addCategory();
case 951543133:
return addControl();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1034364087:
/* number */ return new String[] { "unsignedInt" };
case 382350310:
/* classification */ return new String[] { "Coding" };
case 50511102:
/* category */ return new String[] { "Coding" };
case 951543133:
/* control */ return new String[] { "Coding" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("number")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.number");
} else if (name.equals("classification")) {
this.classification = new Coding();
return this.classification;
} else if (name.equals("category")) {
return addCategory();
} else if (name.equals("control")) {
return addControl();
} else
return super.addChild(name);
}
public SecurityLabelComponent copy() {
SecurityLabelComponent dst = new SecurityLabelComponent();
copyValues(dst);
return dst;
}
public void copyValues(SecurityLabelComponent dst) {
super.copyValues(dst);
if (number != null) {
dst.number = new ArrayList();
for (UnsignedIntType i : number)
dst.number.add(i.copy());
}
;
dst.classification = classification == null ? null : classification.copy();
if (category != null) {
dst.category = new ArrayList();
for (Coding i : category)
dst.category.add(i.copy());
}
;
if (control != null) {
dst.control = new ArrayList();
for (Coding i : control)
dst.control.add(i.copy());
}
;
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof SecurityLabelComponent))
return false;
SecurityLabelComponent o = (SecurityLabelComponent) other_;
return compareDeep(number, o.number, true) && compareDeep(classification, o.classification, true)
&& compareDeep(category, o.category, true) && compareDeep(control, o.control, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof SecurityLabelComponent))
return false;
SecurityLabelComponent o = (SecurityLabelComponent) other_;
return compareValues(number, o.number, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, classification, category, control);
}
public String fhirType() {
return "Contract.term.securityLabel";
}
}
@Block()
public static class ContractOfferComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Unique identifier for this particular Contract Provision.
*/
@Child(name = "identifier", type = {
Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Offer business ID", formalDefinition = "Unique identifier for this particular Contract Provision.")
protected List identifier;
/**
* Offer Recipient.
*/
@Child(name = "party", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Offer Recipient", formalDefinition = "Offer Recipient.")
protected List party;
/**
* The owner of an asset has the residual control rights over the asset: the
* right to decide all usages of the asset in any way not inconsistent with a
* prior contract, custom, or law (Hart, 1995, p. 30).
*/
@Child(name = "topic", type = { Reference.class }, order = 3, min = 0, max = 1, modifier = false, summary = true)
@Description(shortDefinition = "Negotiable offer asset", formalDefinition = "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).")
protected Reference topic;
/**
* The actual object that is the target of the reference (The owner of an asset
* has the residual control rights over the asset: the right to decide all
* usages of the asset in any way not inconsistent with a prior contract,
* custom, or law (Hart, 1995, p. 30).)
*/
protected Resource topicTarget;
/**
* Type of Contract Provision such as specific requirements, purposes for
* actions, obligations, prohibitions, e.g. life time maximum benefit.
*/
@Child(name = "type", type = {
CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Offer Type or Form", formalDefinition = "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type")
protected CodeableConcept type;
/**
* Type of choice made by accepting party with respect to an offer made by an
* offeror/ grantee.
*/
@Child(name = "decision", type = {
CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Accepting party choice", formalDefinition = "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActConsentDirective")
protected CodeableConcept decision;
/**
* How the decision about a Contract was conveyed.
*/
@Child(name = "decisionMode", type = {
CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "How decision is conveyed", formalDefinition = "How the decision about a Contract was conveyed.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-decision-mode")
protected List decisionMode;
/**
* Response to offer text.
*/
@Child(name = "answer", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Response to offer text", formalDefinition = "Response to offer text.")
protected List answer;
/**
* Human readable form of this Contract Offer.
*/
@Child(name = "text", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Human readable offer text", formalDefinition = "Human readable form of this Contract Offer.")
protected StringType text;
/**
* The id of the clause or question text of the offer in the referenced
* questionnaire/response.
*/
@Child(name = "linkId", type = {
StringType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Pointer to text", formalDefinition = "The id of the clause or question text of the offer in the referenced questionnaire/response.")
protected List linkId;
/**
* Security labels that protects the offer.
*/
@Child(name = "securityLabelNumber", type = {
UnsignedIntType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Offer restriction numbers", formalDefinition = "Security labels that protects the offer.")
protected List securityLabelNumber;
private static final long serialVersionUID = -395674449L;
/**
* Constructor
*/
public ContractOfferComponent() {
super();
}
/**
* @return {@link #identifier} (Unique identifier for this particular Contract
* Provision.)
*/
public List getIdentifier() {
if (this.identifier == null)
this.identifier = new ArrayList();
return this.identifier;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setIdentifier(List theIdentifier) {
this.identifier = theIdentifier;
return this;
}
public boolean hasIdentifier() {
if (this.identifier == null)
return false;
for (Identifier item : this.identifier)
if (!item.isEmpty())
return true;
return false;
}
public Identifier addIdentifier() { // 3
Identifier t = new Identifier();
if (this.identifier == null)
this.identifier = new ArrayList();
this.identifier.add(t);
return t;
}
public ContractOfferComponent addIdentifier(Identifier t) { // 3
if (t == null)
return this;
if (this.identifier == null)
this.identifier = new ArrayList();
this.identifier.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #identifier}, creating
* it if it does not already exist
*/
public Identifier getIdentifierFirstRep() {
if (getIdentifier().isEmpty()) {
addIdentifier();
}
return getIdentifier().get(0);
}
/**
* @return {@link #party} (Offer Recipient.)
*/
public List getParty() {
if (this.party == null)
this.party = new ArrayList();
return this.party;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setParty(List theParty) {
this.party = theParty;
return this;
}
public boolean hasParty() {
if (this.party == null)
return false;
for (ContractPartyComponent item : this.party)
if (!item.isEmpty())
return true;
return false;
}
public ContractPartyComponent addParty() { // 3
ContractPartyComponent t = new ContractPartyComponent();
if (this.party == null)
this.party = new ArrayList();
this.party.add(t);
return t;
}
public ContractOfferComponent addParty(ContractPartyComponent t) { // 3
if (t == null)
return this;
if (this.party == null)
this.party = new ArrayList();
this.party.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #party}, creating it
* if it does not already exist
*/
public ContractPartyComponent getPartyFirstRep() {
if (getParty().isEmpty()) {
addParty();
}
return getParty().get(0);
}
/**
* @return {@link #topic} (The owner of an asset has the residual control rights
* over the asset: the right to decide all usages of the asset in any
* way not inconsistent with a prior contract, custom, or law (Hart,
* 1995, p. 30).)
*/
public Reference getTopic() {
if (this.topic == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractOfferComponent.topic");
else if (Configuration.doAutoCreate())
this.topic = new Reference(); // cc
return this.topic;
}
public boolean hasTopic() {
return this.topic != null && !this.topic.isEmpty();
}
/**
* @param value {@link #topic} (The owner of an asset has the residual control
* rights over the asset: the right to decide all usages of the
* asset in any way not inconsistent with a prior contract, custom,
* or law (Hart, 1995, p. 30).)
*/
public ContractOfferComponent setTopic(Reference value) {
this.topic = value;
return this;
}
/**
* @return {@link #topic} The actual object that is the target of the reference.
* The reference library doesn't populate this, but you can use it to
* hold the resource if you resolve it. (The owner of an asset has the
* residual control rights over the asset: the right to decide all
* usages of the asset in any way not inconsistent with a prior
* contract, custom, or law (Hart, 1995, p. 30).)
*/
public Resource getTopicTarget() {
return this.topicTarget;
}
/**
* @param value {@link #topic} The actual object that is the target of the
* reference. The reference library doesn't use these, but you can
* use it to hold the resource if you resolve it. (The owner of an
* asset has the residual control rights over the asset: the right
* to decide all usages of the asset in any way not inconsistent
* with a prior contract, custom, or law (Hart, 1995, p. 30).)
*/
public ContractOfferComponent setTopicTarget(Resource value) {
this.topicTarget = value;
return this;
}
/**
* @return {@link #type} (Type of Contract Provision such as specific
* requirements, purposes for actions, obligations, prohibitions, e.g.
* life time maximum benefit.)
*/
public CodeableConcept getType() {
if (this.type == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractOfferComponent.type");
else if (Configuration.doAutoCreate())
this.type = new CodeableConcept(); // cc
return this.type;
}
public boolean hasType() {
return this.type != null && !this.type.isEmpty();
}
/**
* @param value {@link #type} (Type of Contract Provision such as specific
* requirements, purposes for actions, obligations, prohibitions,
* e.g. life time maximum benefit.)
*/
public ContractOfferComponent setType(CodeableConcept value) {
this.type = value;
return this;
}
/**
* @return {@link #decision} (Type of choice made by accepting party with
* respect to an offer made by an offeror/ grantee.)
*/
public CodeableConcept getDecision() {
if (this.decision == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractOfferComponent.decision");
else if (Configuration.doAutoCreate())
this.decision = new CodeableConcept(); // cc
return this.decision;
}
public boolean hasDecision() {
return this.decision != null && !this.decision.isEmpty();
}
/**
* @param value {@link #decision} (Type of choice made by accepting party with
* respect to an offer made by an offeror/ grantee.)
*/
public ContractOfferComponent setDecision(CodeableConcept value) {
this.decision = value;
return this;
}
/**
* @return {@link #decisionMode} (How the decision about a Contract was
* conveyed.)
*/
public List getDecisionMode() {
if (this.decisionMode == null)
this.decisionMode = new ArrayList();
return this.decisionMode;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setDecisionMode(List theDecisionMode) {
this.decisionMode = theDecisionMode;
return this;
}
public boolean hasDecisionMode() {
if (this.decisionMode == null)
return false;
for (CodeableConcept item : this.decisionMode)
if (!item.isEmpty())
return true;
return false;
}
public CodeableConcept addDecisionMode() { // 3
CodeableConcept t = new CodeableConcept();
if (this.decisionMode == null)
this.decisionMode = new ArrayList();
this.decisionMode.add(t);
return t;
}
public ContractOfferComponent addDecisionMode(CodeableConcept t) { // 3
if (t == null)
return this;
if (this.decisionMode == null)
this.decisionMode = new ArrayList();
this.decisionMode.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #decisionMode},
* creating it if it does not already exist
*/
public CodeableConcept getDecisionModeFirstRep() {
if (getDecisionMode().isEmpty()) {
addDecisionMode();
}
return getDecisionMode().get(0);
}
/**
* @return {@link #answer} (Response to offer text.)
*/
public List getAnswer() {
if (this.answer == null)
this.answer = new ArrayList();
return this.answer;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setAnswer(List theAnswer) {
this.answer = theAnswer;
return this;
}
public boolean hasAnswer() {
if (this.answer == null)
return false;
for (AnswerComponent item : this.answer)
if (!item.isEmpty())
return true;
return false;
}
public AnswerComponent addAnswer() { // 3
AnswerComponent t = new AnswerComponent();
if (this.answer == null)
this.answer = new ArrayList();
this.answer.add(t);
return t;
}
public ContractOfferComponent addAnswer(AnswerComponent t) { // 3
if (t == null)
return this;
if (this.answer == null)
this.answer = new ArrayList();
this.answer.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #answer}, creating it
* if it does not already exist
*/
public AnswerComponent getAnswerFirstRep() {
if (getAnswer().isEmpty()) {
addAnswer();
}
return getAnswer().get(0);
}
/**
* @return {@link #text} (Human readable form of this Contract Offer.). This is
* the underlying object with id, value and extensions. The accessor
* "getText" gives direct access to the value
*/
public StringType getTextElement() {
if (this.text == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractOfferComponent.text");
else if (Configuration.doAutoCreate())
this.text = new StringType(); // bb
return this.text;
}
public boolean hasTextElement() {
return this.text != null && !this.text.isEmpty();
}
public boolean hasText() {
return this.text != null && !this.text.isEmpty();
}
/**
* @param value {@link #text} (Human readable form of this Contract Offer.).
* This is the underlying object with id, value and extensions. The
* accessor "getText" gives direct access to the value
*/
public ContractOfferComponent setTextElement(StringType value) {
this.text = value;
return this;
}
/**
* @return Human readable form of this Contract Offer.
*/
public String getText() {
return this.text == null ? null : this.text.getValue();
}
/**
* @param value Human readable form of this Contract Offer.
*/
public ContractOfferComponent setText(String value) {
if (Utilities.noString(value))
this.text = null;
else {
if (this.text == null)
this.text = new StringType();
this.text.setValue(value);
}
return this;
}
/**
* @return {@link #linkId} (The id of the clause or question text of the offer
* in the referenced questionnaire/response.)
*/
public List getLinkId() {
if (this.linkId == null)
this.linkId = new ArrayList();
return this.linkId;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setLinkId(List theLinkId) {
this.linkId = theLinkId;
return this;
}
public boolean hasLinkId() {
if (this.linkId == null)
return false;
for (StringType item : this.linkId)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #linkId} (The id of the clause or question text of the offer
* in the referenced questionnaire/response.)
*/
public StringType addLinkIdElement() {// 2
StringType t = new StringType();
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return t;
}
/**
* @param value {@link #linkId} (The id of the clause or question text of the
* offer in the referenced questionnaire/response.)
*/
public ContractOfferComponent addLinkId(String value) { // 1
StringType t = new StringType();
t.setValue(value);
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return this;
}
/**
* @param value {@link #linkId} (The id of the clause or question text of the
* offer in the referenced questionnaire/response.)
*/
public boolean hasLinkId(String value) {
if (this.linkId == null)
return false;
for (StringType v : this.linkId)
if (v.getValue().equals(value)) // string
return true;
return false;
}
/**
* @return {@link #securityLabelNumber} (Security labels that protects the
* offer.)
*/
public List getSecurityLabelNumber() {
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
return this.securityLabelNumber;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractOfferComponent setSecurityLabelNumber(List theSecurityLabelNumber) {
this.securityLabelNumber = theSecurityLabelNumber;
return this;
}
public boolean hasSecurityLabelNumber() {
if (this.securityLabelNumber == null)
return false;
for (UnsignedIntType item : this.securityLabelNumber)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #securityLabelNumber} (Security labels that protects the
* offer.)
*/
public UnsignedIntType addSecurityLabelNumberElement() {// 2
UnsignedIntType t = new UnsignedIntType();
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
this.securityLabelNumber.add(t);
return t;
}
/**
* @param value {@link #securityLabelNumber} (Security labels that protects the
* offer.)
*/
public ContractOfferComponent addSecurityLabelNumber(int value) { // 1
UnsignedIntType t = new UnsignedIntType();
t.setValue(value);
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
this.securityLabelNumber.add(t);
return this;
}
/**
* @param value {@link #securityLabelNumber} (Security labels that protects the
* offer.)
*/
public boolean hasSecurityLabelNumber(int value) {
if (this.securityLabelNumber == null)
return false;
for (UnsignedIntType v : this.securityLabelNumber)
if (v.getValue().equals(value)) // unsignedInt
return true;
return false;
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.",
0, java.lang.Integer.MAX_VALUE, identifier));
children.add(new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party));
children.add(new Property("topic", "Reference(Any)",
"The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).",
0, 1, topic));
children.add(new Property("type", "CodeableConcept",
"Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.",
0, 1, type));
children.add(new Property("decision", "CodeableConcept",
"Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1,
decision));
children.add(new Property("decisionMode", "CodeableConcept", "How the decision about a Contract was conveyed.", 0,
java.lang.Integer.MAX_VALUE, decisionMode));
children.add(new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, answer));
children.add(new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text));
children.add(new Property("linkId", "string",
"The id of the clause or question text of the offer in the referenced questionnaire/response.", 0,
java.lang.Integer.MAX_VALUE, linkId));
children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the offer.", 0,
java.lang.Integer.MAX_VALUE, securityLabelNumber));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -1618432855:
/* identifier */ return new Property("identifier", "Identifier",
"Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier);
case 106437350:
/* party */ return new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party);
case 110546223:
/* topic */ return new Property("topic", "Reference(Any)",
"The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).",
0, 1, topic);
case 3575610:
/* type */ return new Property("type", "CodeableConcept",
"Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.",
0, 1, type);
case 565719004:
/* decision */ return new Property("decision", "CodeableConcept",
"Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1,
decision);
case 675909535:
/* decisionMode */ return new Property("decisionMode", "CodeableConcept",
"How the decision about a Contract was conveyed.", 0, java.lang.Integer.MAX_VALUE, decisionMode);
case -1412808770:
/* answer */ return new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE,
answer);
case 3556653:
/* text */ return new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text);
case -1102667083:
/* linkId */ return new Property("linkId", "string",
"The id of the clause or question text of the offer in the referenced questionnaire/response.", 0,
java.lang.Integer.MAX_VALUE, linkId);
case -149460995:
/* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt",
"Security labels that protects the offer.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case -1618432855:
/* identifier */ return this.identifier == null ? new Base[0]
: this.identifier.toArray(new Base[this.identifier.size()]); // Identifier
case 106437350:
/* party */ return this.party == null ? new Base[0] : this.party.toArray(new Base[this.party.size()]); // ContractPartyComponent
case 110546223:
/* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Reference
case 3575610:
/* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept
case 565719004:
/* decision */ return this.decision == null ? new Base[0] : new Base[] { this.decision }; // CodeableConcept
case 675909535:
/* decisionMode */ return this.decisionMode == null ? new Base[0]
: this.decisionMode.toArray(new Base[this.decisionMode.size()]); // CodeableConcept
case -1412808770:
/* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent
case 3556653:
/* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType
case -1102667083:
/* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType
case -149460995:
/* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0]
: this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case -1618432855: // identifier
this.getIdentifier().add(castToIdentifier(value)); // Identifier
return value;
case 106437350: // party
this.getParty().add((ContractPartyComponent) value); // ContractPartyComponent
return value;
case 110546223: // topic
this.topic = castToReference(value); // Reference
return value;
case 3575610: // type
this.type = castToCodeableConcept(value); // CodeableConcept
return value;
case 565719004: // decision
this.decision = castToCodeableConcept(value); // CodeableConcept
return value;
case 675909535: // decisionMode
this.getDecisionMode().add(castToCodeableConcept(value)); // CodeableConcept
return value;
case -1412808770: // answer
this.getAnswer().add((AnswerComponent) value); // AnswerComponent
return value;
case 3556653: // text
this.text = castToString(value); // StringType
return value;
case -1102667083: // linkId
this.getLinkId().add(castToString(value)); // StringType
return value;
case -149460995: // securityLabelNumber
this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("identifier")) {
this.getIdentifier().add(castToIdentifier(value));
} else if (name.equals("party")) {
this.getParty().add((ContractPartyComponent) value);
} else if (name.equals("topic")) {
this.topic = castToReference(value); // Reference
} else if (name.equals("type")) {
this.type = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("decision")) {
this.decision = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("decisionMode")) {
this.getDecisionMode().add(castToCodeableConcept(value));
} else if (name.equals("answer")) {
this.getAnswer().add((AnswerComponent) value);
} else if (name.equals("text")) {
this.text = castToString(value); // StringType
} else if (name.equals("linkId")) {
this.getLinkId().add(castToString(value));
} else if (name.equals("securityLabelNumber")) {
this.getSecurityLabelNumber().add(castToUnsignedInt(value));
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("identifier")) {
this.getIdentifier().remove(castToIdentifier(value));
} else if (name.equals("party")) {
this.getParty().remove((ContractPartyComponent) value);
} else if (name.equals("topic")) {
this.topic = null;
} else if (name.equals("type")) {
this.type = null;
} else if (name.equals("decision")) {
this.decision = null;
} else if (name.equals("decisionMode")) {
this.getDecisionMode().remove(castToCodeableConcept(value));
} else if (name.equals("answer")) {
this.getAnswer().remove((AnswerComponent) value);
} else if (name.equals("text")) {
this.text = null;
} else if (name.equals("linkId")) {
this.getLinkId().remove(castToString(value));
} else if (name.equals("securityLabelNumber")) {
this.getSecurityLabelNumber().remove(castToUnsignedInt(value));
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1618432855:
return addIdentifier();
case 106437350:
return addParty();
case 110546223:
return getTopic();
case 3575610:
return getType();
case 565719004:
return getDecision();
case 675909535:
return addDecisionMode();
case -1412808770:
return addAnswer();
case 3556653:
return getTextElement();
case -1102667083:
return addLinkIdElement();
case -149460995:
return addSecurityLabelNumberElement();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1618432855:
/* identifier */ return new String[] { "Identifier" };
case 106437350:
/* party */ return new String[] {};
case 110546223:
/* topic */ return new String[] { "Reference" };
case 3575610:
/* type */ return new String[] { "CodeableConcept" };
case 565719004:
/* decision */ return new String[] { "CodeableConcept" };
case 675909535:
/* decisionMode */ return new String[] { "CodeableConcept" };
case -1412808770:
/* answer */ return new String[] {};
case 3556653:
/* text */ return new String[] { "string" };
case -1102667083:
/* linkId */ return new String[] { "string" };
case -149460995:
/* securityLabelNumber */ return new String[] { "unsignedInt" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("identifier")) {
return addIdentifier();
} else if (name.equals("party")) {
return addParty();
} else if (name.equals("topic")) {
this.topic = new Reference();
return this.topic;
} else if (name.equals("type")) {
this.type = new CodeableConcept();
return this.type;
} else if (name.equals("decision")) {
this.decision = new CodeableConcept();
return this.decision;
} else if (name.equals("decisionMode")) {
return addDecisionMode();
} else if (name.equals("answer")) {
return addAnswer();
} else if (name.equals("text")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.text");
} else if (name.equals("linkId")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId");
} else if (name.equals("securityLabelNumber")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber");
} else
return super.addChild(name);
}
public ContractOfferComponent copy() {
ContractOfferComponent dst = new ContractOfferComponent();
copyValues(dst);
return dst;
}
public void copyValues(ContractOfferComponent dst) {
super.copyValues(dst);
if (identifier != null) {
dst.identifier = new ArrayList();
for (Identifier i : identifier)
dst.identifier.add(i.copy());
}
;
if (party != null) {
dst.party = new ArrayList();
for (ContractPartyComponent i : party)
dst.party.add(i.copy());
}
;
dst.topic = topic == null ? null : topic.copy();
dst.type = type == null ? null : type.copy();
dst.decision = decision == null ? null : decision.copy();
if (decisionMode != null) {
dst.decisionMode = new ArrayList();
for (CodeableConcept i : decisionMode)
dst.decisionMode.add(i.copy());
}
;
if (answer != null) {
dst.answer = new ArrayList();
for (AnswerComponent i : answer)
dst.answer.add(i.copy());
}
;
dst.text = text == null ? null : text.copy();
if (linkId != null) {
dst.linkId = new ArrayList();
for (StringType i : linkId)
dst.linkId.add(i.copy());
}
;
if (securityLabelNumber != null) {
dst.securityLabelNumber = new ArrayList();
for (UnsignedIntType i : securityLabelNumber)
dst.securityLabelNumber.add(i.copy());
}
;
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof ContractOfferComponent))
return false;
ContractOfferComponent o = (ContractOfferComponent) other_;
return compareDeep(identifier, o.identifier, true) && compareDeep(party, o.party, true)
&& compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true)
&& compareDeep(decision, o.decision, true) && compareDeep(decisionMode, o.decisionMode, true)
&& compareDeep(answer, o.answer, true) && compareDeep(text, o.text, true)
&& compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof ContractOfferComponent))
return false;
ContractOfferComponent o = (ContractOfferComponent) other_;
return compareValues(text, o.text, true) && compareValues(linkId, o.linkId, true)
&& compareValues(securityLabelNumber, o.securityLabelNumber, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, party, topic, type, decision,
decisionMode, answer, text, linkId, securityLabelNumber);
}
public String fhirType() {
return "Contract.term.offer";
}
}
@Block()
public static class ContractPartyComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Participant in the offer.
*/
@Child(name = "reference", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class,
Device.class, Group.class,
Organization.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Referenced entity", formalDefinition = "Participant in the offer.")
protected List reference;
/**
* The actual objects that are the target of the reference (Participant in the
* offer.)
*/
protected List referenceTarget;
/**
* How the party participates in the offer.
*/
@Child(name = "role", type = {
CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Participant engagement type", formalDefinition = "How the party participates in the offer.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-party-role")
protected CodeableConcept role;
private static final long serialVersionUID = 128949255L;
/**
* Constructor
*/
public ContractPartyComponent() {
super();
}
/**
* Constructor
*/
public ContractPartyComponent(CodeableConcept role) {
super();
this.role = role;
}
/**
* @return {@link #reference} (Participant in the offer.)
*/
public List getReference() {
if (this.reference == null)
this.reference = new ArrayList();
return this.reference;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractPartyComponent setReference(List theReference) {
this.reference = theReference;
return this;
}
public boolean hasReference() {
if (this.reference == null)
return false;
for (Reference item : this.reference)
if (!item.isEmpty())
return true;
return false;
}
public Reference addReference() { // 3
Reference t = new Reference();
if (this.reference == null)
this.reference = new ArrayList();
this.reference.add(t);
return t;
}
public ContractPartyComponent addReference(Reference t) { // 3
if (t == null)
return this;
if (this.reference == null)
this.reference = new ArrayList();
this.reference.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #reference}, creating
* it if it does not already exist
*/
public Reference getReferenceFirstRep() {
if (getReference().isEmpty()) {
addReference();
}
return getReference().get(0);
}
/**
* @deprecated Use Reference#setResource(IBaseResource) instead
*/
@Deprecated
public List getReferenceTarget() {
if (this.referenceTarget == null)
this.referenceTarget = new ArrayList();
return this.referenceTarget;
}
/**
* @return {@link #role} (How the party participates in the offer.)
*/
public CodeableConcept getRole() {
if (this.role == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractPartyComponent.role");
else if (Configuration.doAutoCreate())
this.role = new CodeableConcept(); // cc
return this.role;
}
public boolean hasRole() {
return this.role != null && !this.role.isEmpty();
}
/**
* @param value {@link #role} (How the party participates in the offer.)
*/
public ContractPartyComponent setRole(CodeableConcept value) {
this.role = value;
return this;
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("reference",
"Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)",
"Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference));
children.add(new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, role));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -925155509:
/* reference */ return new Property("reference",
"Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)",
"Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference);
case 3506294:
/* role */ return new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1,
role);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case -925155509:
/* reference */ return this.reference == null ? new Base[0]
: this.reference.toArray(new Base[this.reference.size()]); // Reference
case 3506294:
/* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case -925155509: // reference
this.getReference().add(castToReference(value)); // Reference
return value;
case 3506294: // role
this.role = castToCodeableConcept(value); // CodeableConcept
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("reference")) {
this.getReference().add(castToReference(value));
} else if (name.equals("role")) {
this.role = castToCodeableConcept(value); // CodeableConcept
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("reference")) {
this.getReference().remove(castToReference(value));
} else if (name.equals("role")) {
this.role = null;
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -925155509:
return addReference();
case 3506294:
return getRole();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -925155509:
/* reference */ return new String[] { "Reference" };
case 3506294:
/* role */ return new String[] { "CodeableConcept" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("reference")) {
return addReference();
} else if (name.equals("role")) {
this.role = new CodeableConcept();
return this.role;
} else
return super.addChild(name);
}
public ContractPartyComponent copy() {
ContractPartyComponent dst = new ContractPartyComponent();
copyValues(dst);
return dst;
}
public void copyValues(ContractPartyComponent dst) {
super.copyValues(dst);
if (reference != null) {
dst.reference = new ArrayList();
for (Reference i : reference)
dst.reference.add(i.copy());
}
;
dst.role = role == null ? null : role.copy();
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof ContractPartyComponent))
return false;
ContractPartyComponent o = (ContractPartyComponent) other_;
return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof ContractPartyComponent))
return false;
ContractPartyComponent o = (ContractPartyComponent) other_;
return true;
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role);
}
public String fhirType() {
return "Contract.term.offer.party";
}
}
@Block()
public static class AnswerComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Response to an offer clause or question text, which enables selection of
* values to be agreed to, e.g., the period of participation, the date of
* occupancy of a rental, warrently duration, or whether biospecimen may be used
* for further research.
*/
@Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class,
DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class,
Quantity.class, Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "The actual answer response", formalDefinition = "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.")
protected Type value;
private static final long serialVersionUID = -732981989L;
/**
* Constructor
*/
public AnswerComponent() {
super();
}
/**
* Constructor
*/
public AnswerComponent(Type value) {
super();
this.value = value;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public Type getValue() {
return this.value;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public BooleanType getValueBooleanType() throws FHIRException {
if (this.value == null)
this.value = new BooleanType();
if (!(this.value instanceof BooleanType))
throw new FHIRException("Type mismatch: the type BooleanType was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (BooleanType) this.value;
}
public boolean hasValueBooleanType() {
return this != null && this.value instanceof BooleanType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public DecimalType getValueDecimalType() throws FHIRException {
if (this.value == null)
this.value = new DecimalType();
if (!(this.value instanceof DecimalType))
throw new FHIRException("Type mismatch: the type DecimalType was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (DecimalType) this.value;
}
public boolean hasValueDecimalType() {
return this != null && this.value instanceof DecimalType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public IntegerType getValueIntegerType() throws FHIRException {
if (this.value == null)
this.value = new IntegerType();
if (!(this.value instanceof IntegerType))
throw new FHIRException("Type mismatch: the type IntegerType was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (IntegerType) this.value;
}
public boolean hasValueIntegerType() {
return this != null && this.value instanceof IntegerType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public DateType getValueDateType() throws FHIRException {
if (this.value == null)
this.value = new DateType();
if (!(this.value instanceof DateType))
throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName()
+ " was encountered");
return (DateType) this.value;
}
public boolean hasValueDateType() {
return this != null && this.value instanceof DateType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public DateTimeType getValueDateTimeType() throws FHIRException {
if (this.value == null)
this.value = new DateTimeType();
if (!(this.value instanceof DateTimeType))
throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (DateTimeType) this.value;
}
public boolean hasValueDateTimeType() {
return this != null && this.value instanceof DateTimeType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public TimeType getValueTimeType() throws FHIRException {
if (this.value == null)
this.value = new TimeType();
if (!(this.value instanceof TimeType))
throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName()
+ " was encountered");
return (TimeType) this.value;
}
public boolean hasValueTimeType() {
return this != null && this.value instanceof TimeType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public StringType getValueStringType() throws FHIRException {
if (this.value == null)
this.value = new StringType();
if (!(this.value instanceof StringType))
throw new FHIRException("Type mismatch: the type StringType was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (StringType) this.value;
}
public boolean hasValueStringType() {
return this != null && this.value instanceof StringType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public UriType getValueUriType() throws FHIRException {
if (this.value == null)
this.value = new UriType();
if (!(this.value instanceof UriType))
throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName()
+ " was encountered");
return (UriType) this.value;
}
public boolean hasValueUriType() {
return this != null && this.value instanceof UriType;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public Attachment getValueAttachment() throws FHIRException {
if (this.value == null)
this.value = new Attachment();
if (!(this.value instanceof Attachment))
throw new FHIRException("Type mismatch: the type Attachment was expected, but "
+ this.value.getClass().getName() + " was encountered");
return (Attachment) this.value;
}
public boolean hasValueAttachment() {
return this != null && this.value instanceof Attachment;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public Coding getValueCoding() throws FHIRException {
if (this.value == null)
this.value = new Coding();
if (!(this.value instanceof Coding))
throw new FHIRException(
"Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered");
return (Coding) this.value;
}
public boolean hasValueCoding() {
return this != null && this.value instanceof Coding;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public Quantity getValueQuantity() throws FHIRException {
if (this.value == null)
this.value = new Quantity();
if (!(this.value instanceof Quantity))
throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName()
+ " was encountered");
return (Quantity) this.value;
}
public boolean hasValueQuantity() {
return this != null && this.value instanceof Quantity;
}
/**
* @return {@link #value} (Response to an offer clause or question text, which
* enables selection of values to be agreed to, e.g., the period of
* participation, the date of occupancy of a rental, warrently duration,
* or whether biospecimen may be used for further research.)
*/
public Reference getValueReference() throws FHIRException {
if (this.value == null)
this.value = new Reference();
if (!(this.value instanceof Reference))
throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName()
+ " was encountered");
return (Reference) this.value;
}
public boolean hasValueReference() {
return this != null && this.value instanceof Reference;
}
public boolean hasValue() {
return this.value != null && !this.value.isEmpty();
}
/**
* @param value {@link #value} (Response to an offer clause or question text,
* which enables selection of values to be agreed to, e.g., the
* period of participation, the date of occupancy of a rental,
* warrently duration, or whether biospecimen may be used for
* further research.)
*/
public AnswerComponent setValue(Type value) {
if (value != null
&& !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType
|| value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType
|| value instanceof StringType || value instanceof UriType || value instanceof Attachment
|| value instanceof Coding || value instanceof Quantity || value instanceof Reference))
throw new Error("Not the right type for Contract.term.offer.answer.value[x]: " + value.fhirType());
this.value = value;
return this;
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -1410166417:
/* value[x] */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case 111972721:
/* value */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case 733421943:
/* valueBoolean */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -2083993440:
/* valueDecimal */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -1668204915:
/* valueInteger */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -766192449:
/* valueDate */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case 1047929900:
/* valueDateTime */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -765708322:
/* valueTime */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -1424603934:
/* valueString */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -1410172357:
/* valueUri */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -475566732:
/* valueAttachment */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -1887705029:
/* valueCoding */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case -2029823716:
/* valueQuantity */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
case 1755241690:
/* valueReference */ return new Property("value[x]",
"boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)",
"Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.",
0, 1, value);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case 111972721:
/* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case 111972721: // value
this.value = castToType(value); // Type
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("value[x]")) {
this.value = castToType(value); // Type
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("value[x]")) {
this.value = null;
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -1410166417:
return getValue();
case 111972721:
return getValue();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case 111972721:
/* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri",
"Attachment", "Coding", "Quantity", "Reference" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("valueBoolean")) {
this.value = new BooleanType();
return this.value;
} else if (name.equals("valueDecimal")) {
this.value = new DecimalType();
return this.value;
} else if (name.equals("valueInteger")) {
this.value = new IntegerType();
return this.value;
} else if (name.equals("valueDate")) {
this.value = new DateType();
return this.value;
} else if (name.equals("valueDateTime")) {
this.value = new DateTimeType();
return this.value;
} else if (name.equals("valueTime")) {
this.value = new TimeType();
return this.value;
} else if (name.equals("valueString")) {
this.value = new StringType();
return this.value;
} else if (name.equals("valueUri")) {
this.value = new UriType();
return this.value;
} else if (name.equals("valueAttachment")) {
this.value = new Attachment();
return this.value;
} else if (name.equals("valueCoding")) {
this.value = new Coding();
return this.value;
} else if (name.equals("valueQuantity")) {
this.value = new Quantity();
return this.value;
} else if (name.equals("valueReference")) {
this.value = new Reference();
return this.value;
} else
return super.addChild(name);
}
public AnswerComponent copy() {
AnswerComponent dst = new AnswerComponent();
copyValues(dst);
return dst;
}
public void copyValues(AnswerComponent dst) {
super.copyValues(dst);
dst.value = value == null ? null : value.copy();
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof AnswerComponent))
return false;
AnswerComponent o = (AnswerComponent) other_;
return compareDeep(value, o.value, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof AnswerComponent))
return false;
AnswerComponent o = (AnswerComponent) other_;
return true;
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value);
}
public String fhirType() {
return "Contract.term.offer.answer";
}
}
@Block()
public static class ContractAssetComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Differentiates the kind of the asset .
*/
@Child(name = "scope", type = {
CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Range of asset", formalDefinition = "Differentiates the kind of the asset .")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetscope")
protected CodeableConcept scope;
/**
* Target entity type about which the term may be concerned.
*/
@Child(name = "type", type = {
CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Asset category", formalDefinition = "Target entity type about which the term may be concerned.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assettype")
protected List type;
/**
* Associated entities.
*/
@Child(name = "typeReference", type = {
Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Associated entities", formalDefinition = "Associated entities.")
protected List typeReference;
/**
* The actual objects that are the target of the reference (Associated
* entities.)
*/
protected List typeReferenceTarget;
/**
* May be a subtype or part of an offered asset.
*/
@Child(name = "subtype", type = {
CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Asset sub-category", formalDefinition = "May be a subtype or part of an offered asset.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetsubtype")
protected List subtype;
/**
* Specifies the applicability of the term to an asset resource instance, and
* instances it refers to orinstances that refer to it, and/or are owned by the
* offeree.
*/
@Child(name = "relationship", type = {
Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Kinship of the asset", formalDefinition = "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-content-class")
protected Coding relationship;
/**
* Circumstance of the asset.
*/
@Child(name = "context", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Circumstance of the asset", formalDefinition = "Circumstance of the asset.")
protected List context;
/**
* Description of the quality and completeness of the asset that imay be a
* factor in its valuation.
*/
@Child(name = "condition", type = {
StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Quality desctiption of asset", formalDefinition = "Description of the quality and completeness of the asset that imay be a factor in its valuation.")
protected StringType condition;
/**
* Type of Asset availability for use or ownership.
*/
@Child(name = "periodType", type = {
CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Asset availability types", formalDefinition = "Type of Asset availability for use or ownership.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/asset-availability")
protected List periodType;
/**
* Asset relevant contractual time period.
*/
@Child(name = "period", type = {
Period.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Time period of the asset", formalDefinition = "Asset relevant contractual time period.")
protected List period;
/**
* Time period of asset use.
*/
@Child(name = "usePeriod", type = {
Period.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Time period", formalDefinition = "Time period of asset use.")
protected List usePeriod;
/**
* Clause or question text (Prose Object) concerning the asset in a linked form,
* such as a QuestionnaireResponse used in the formation of the contract.
*/
@Child(name = "text", type = { StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Asset clause or question text", formalDefinition = "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.")
protected StringType text;
/**
* Id [identifier??] of the clause or question text about the asset in the
* referenced form or QuestionnaireResponse.
*/
@Child(name = "linkId", type = {
StringType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Pointer to asset text", formalDefinition = "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.")
protected List linkId;
/**
* Response to assets.
*/
@Child(name = "answer", type = {
AnswerComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Response to assets", formalDefinition = "Response to assets.")
protected List answer;
/**
* Security labels that protects the asset.
*/
@Child(name = "securityLabelNumber", type = {
UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Asset restriction numbers", formalDefinition = "Security labels that protects the asset.")
protected List securityLabelNumber;
/**
* Contract Valued Item List.
*/
@Child(name = "valuedItem", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item List", formalDefinition = "Contract Valued Item List.")
protected List valuedItem;
private static final long serialVersionUID = -1080398792L;
/**
* Constructor
*/
public ContractAssetComponent() {
super();
}
/**
* @return {@link #scope} (Differentiates the kind of the asset .)
*/
public CodeableConcept getScope() {
if (this.scope == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractAssetComponent.scope");
else if (Configuration.doAutoCreate())
this.scope = new CodeableConcept(); // cc
return this.scope;
}
public boolean hasScope() {
return this.scope != null && !this.scope.isEmpty();
}
/**
* @param value {@link #scope} (Differentiates the kind of the asset .)
*/
public ContractAssetComponent setScope(CodeableConcept value) {
this.scope = value;
return this;
}
/**
* @return {@link #type} (Target entity type about which the term may be
* concerned.)
*/
public List getType() {
if (this.type == null)
this.type = new ArrayList();
return this.type;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setType(List theType) {
this.type = theType;
return this;
}
public boolean hasType() {
if (this.type == null)
return false;
for (CodeableConcept item : this.type)
if (!item.isEmpty())
return true;
return false;
}
public CodeableConcept addType() { // 3
CodeableConcept t = new CodeableConcept();
if (this.type == null)
this.type = new ArrayList();
this.type.add(t);
return t;
}
public ContractAssetComponent addType(CodeableConcept t) { // 3
if (t == null)
return this;
if (this.type == null)
this.type = new ArrayList();
this.type.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #type}, creating it if
* it does not already exist
*/
public CodeableConcept getTypeFirstRep() {
if (getType().isEmpty()) {
addType();
}
return getType().get(0);
}
/**
* @return {@link #typeReference} (Associated entities.)
*/
public List getTypeReference() {
if (this.typeReference == null)
this.typeReference = new ArrayList();
return this.typeReference;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setTypeReference(List theTypeReference) {
this.typeReference = theTypeReference;
return this;
}
public boolean hasTypeReference() {
if (this.typeReference == null)
return false;
for (Reference item : this.typeReference)
if (!item.isEmpty())
return true;
return false;
}
public Reference addTypeReference() { // 3
Reference t = new Reference();
if (this.typeReference == null)
this.typeReference = new ArrayList();
this.typeReference.add(t);
return t;
}
public ContractAssetComponent addTypeReference(Reference t) { // 3
if (t == null)
return this;
if (this.typeReference == null)
this.typeReference = new ArrayList();
this.typeReference.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #typeReference},
* creating it if it does not already exist
*/
public Reference getTypeReferenceFirstRep() {
if (getTypeReference().isEmpty()) {
addTypeReference();
}
return getTypeReference().get(0);
}
/**
* @deprecated Use Reference#setResource(IBaseResource) instead
*/
@Deprecated
public List getTypeReferenceTarget() {
if (this.typeReferenceTarget == null)
this.typeReferenceTarget = new ArrayList();
return this.typeReferenceTarget;
}
/**
* @return {@link #subtype} (May be a subtype or part of an offered asset.)
*/
public List getSubtype() {
if (this.subtype == null)
this.subtype = new ArrayList();
return this.subtype;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setSubtype(List theSubtype) {
this.subtype = theSubtype;
return this;
}
public boolean hasSubtype() {
if (this.subtype == null)
return false;
for (CodeableConcept item : this.subtype)
if (!item.isEmpty())
return true;
return false;
}
public CodeableConcept addSubtype() { // 3
CodeableConcept t = new CodeableConcept();
if (this.subtype == null)
this.subtype = new ArrayList();
this.subtype.add(t);
return t;
}
public ContractAssetComponent addSubtype(CodeableConcept t) { // 3
if (t == null)
return this;
if (this.subtype == null)
this.subtype = new ArrayList();
this.subtype.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #subtype}, creating it
* if it does not already exist
*/
public CodeableConcept getSubtypeFirstRep() {
if (getSubtype().isEmpty()) {
addSubtype();
}
return getSubtype().get(0);
}
/**
* @return {@link #relationship} (Specifies the applicability of the term to an
* asset resource instance, and instances it refers to orinstances that
* refer to it, and/or are owned by the offeree.)
*/
public Coding getRelationship() {
if (this.relationship == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractAssetComponent.relationship");
else if (Configuration.doAutoCreate())
this.relationship = new Coding(); // cc
return this.relationship;
}
public boolean hasRelationship() {
return this.relationship != null && !this.relationship.isEmpty();
}
/**
* @param value {@link #relationship} (Specifies the applicability of the term
* to an asset resource instance, and instances it refers to
* orinstances that refer to it, and/or are owned by the offeree.)
*/
public ContractAssetComponent setRelationship(Coding value) {
this.relationship = value;
return this;
}
/**
* @return {@link #context} (Circumstance of the asset.)
*/
public List getContext() {
if (this.context == null)
this.context = new ArrayList();
return this.context;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setContext(List theContext) {
this.context = theContext;
return this;
}
public boolean hasContext() {
if (this.context == null)
return false;
for (AssetContextComponent item : this.context)
if (!item.isEmpty())
return true;
return false;
}
public AssetContextComponent addContext() { // 3
AssetContextComponent t = new AssetContextComponent();
if (this.context == null)
this.context = new ArrayList();
this.context.add(t);
return t;
}
public ContractAssetComponent addContext(AssetContextComponent t) { // 3
if (t == null)
return this;
if (this.context == null)
this.context = new ArrayList();
this.context.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #context}, creating it
* if it does not already exist
*/
public AssetContextComponent getContextFirstRep() {
if (getContext().isEmpty()) {
addContext();
}
return getContext().get(0);
}
/**
* @return {@link #condition} (Description of the quality and completeness of
* the asset that imay be a factor in its valuation.). This is the
* underlying object with id, value and extensions. The accessor
* "getCondition" gives direct access to the value
*/
public StringType getConditionElement() {
if (this.condition == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractAssetComponent.condition");
else if (Configuration.doAutoCreate())
this.condition = new StringType(); // bb
return this.condition;
}
public boolean hasConditionElement() {
return this.condition != null && !this.condition.isEmpty();
}
public boolean hasCondition() {
return this.condition != null && !this.condition.isEmpty();
}
/**
* @param value {@link #condition} (Description of the quality and completeness
* of the asset that imay be a factor in its valuation.). This is
* the underlying object with id, value and extensions. The
* accessor "getCondition" gives direct access to the value
*/
public ContractAssetComponent setConditionElement(StringType value) {
this.condition = value;
return this;
}
/**
* @return Description of the quality and completeness of the asset that imay be
* a factor in its valuation.
*/
public String getCondition() {
return this.condition == null ? null : this.condition.getValue();
}
/**
* @param value Description of the quality and completeness of the asset that
* imay be a factor in its valuation.
*/
public ContractAssetComponent setCondition(String value) {
if (Utilities.noString(value))
this.condition = null;
else {
if (this.condition == null)
this.condition = new StringType();
this.condition.setValue(value);
}
return this;
}
/**
* @return {@link #periodType} (Type of Asset availability for use or
* ownership.)
*/
public List getPeriodType() {
if (this.periodType == null)
this.periodType = new ArrayList();
return this.periodType;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setPeriodType(List thePeriodType) {
this.periodType = thePeriodType;
return this;
}
public boolean hasPeriodType() {
if (this.periodType == null)
return false;
for (CodeableConcept item : this.periodType)
if (!item.isEmpty())
return true;
return false;
}
public CodeableConcept addPeriodType() { // 3
CodeableConcept t = new CodeableConcept();
if (this.periodType == null)
this.periodType = new ArrayList();
this.periodType.add(t);
return t;
}
public ContractAssetComponent addPeriodType(CodeableConcept t) { // 3
if (t == null)
return this;
if (this.periodType == null)
this.periodType = new ArrayList();
this.periodType.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #periodType}, creating
* it if it does not already exist
*/
public CodeableConcept getPeriodTypeFirstRep() {
if (getPeriodType().isEmpty()) {
addPeriodType();
}
return getPeriodType().get(0);
}
/**
* @return {@link #period} (Asset relevant contractual time period.)
*/
public List getPeriod() {
if (this.period == null)
this.period = new ArrayList();
return this.period;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setPeriod(List thePeriod) {
this.period = thePeriod;
return this;
}
public boolean hasPeriod() {
if (this.period == null)
return false;
for (Period item : this.period)
if (!item.isEmpty())
return true;
return false;
}
public Period addPeriod() { // 3
Period t = new Period();
if (this.period == null)
this.period = new ArrayList();
this.period.add(t);
return t;
}
public ContractAssetComponent addPeriod(Period t) { // 3
if (t == null)
return this;
if (this.period == null)
this.period = new ArrayList();
this.period.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #period}, creating it
* if it does not already exist
*/
public Period getPeriodFirstRep() {
if (getPeriod().isEmpty()) {
addPeriod();
}
return getPeriod().get(0);
}
/**
* @return {@link #usePeriod} (Time period of asset use.)
*/
public List getUsePeriod() {
if (this.usePeriod == null)
this.usePeriod = new ArrayList();
return this.usePeriod;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setUsePeriod(List theUsePeriod) {
this.usePeriod = theUsePeriod;
return this;
}
public boolean hasUsePeriod() {
if (this.usePeriod == null)
return false;
for (Period item : this.usePeriod)
if (!item.isEmpty())
return true;
return false;
}
public Period addUsePeriod() { // 3
Period t = new Period();
if (this.usePeriod == null)
this.usePeriod = new ArrayList();
this.usePeriod.add(t);
return t;
}
public ContractAssetComponent addUsePeriod(Period t) { // 3
if (t == null)
return this;
if (this.usePeriod == null)
this.usePeriod = new ArrayList();
this.usePeriod.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #usePeriod}, creating
* it if it does not already exist
*/
public Period getUsePeriodFirstRep() {
if (getUsePeriod().isEmpty()) {
addUsePeriod();
}
return getUsePeriod().get(0);
}
/**
* @return {@link #text} (Clause or question text (Prose Object) concerning the
* asset in a linked form, such as a QuestionnaireResponse used in the
* formation of the contract.). This is the underlying object with id,
* value and extensions. The accessor "getText" gives direct access to
* the value
*/
public StringType getTextElement() {
if (this.text == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ContractAssetComponent.text");
else if (Configuration.doAutoCreate())
this.text = new StringType(); // bb
return this.text;
}
public boolean hasTextElement() {
return this.text != null && !this.text.isEmpty();
}
public boolean hasText() {
return this.text != null && !this.text.isEmpty();
}
/**
* @param value {@link #text} (Clause or question text (Prose Object) concerning
* the asset in a linked form, such as a QuestionnaireResponse used
* in the formation of the contract.). This is the underlying
* object with id, value and extensions. The accessor "getText"
* gives direct access to the value
*/
public ContractAssetComponent setTextElement(StringType value) {
this.text = value;
return this;
}
/**
* @return Clause or question text (Prose Object) concerning the asset in a
* linked form, such as a QuestionnaireResponse used in the formation of
* the contract.
*/
public String getText() {
return this.text == null ? null : this.text.getValue();
}
/**
* @param value Clause or question text (Prose Object) concerning the asset in a
* linked form, such as a QuestionnaireResponse used in the
* formation of the contract.
*/
public ContractAssetComponent setText(String value) {
if (Utilities.noString(value))
this.text = null;
else {
if (this.text == null)
this.text = new StringType();
this.text.setValue(value);
}
return this;
}
/**
* @return {@link #linkId} (Id [identifier??] of the clause or question text
* about the asset in the referenced form or QuestionnaireResponse.)
*/
public List getLinkId() {
if (this.linkId == null)
this.linkId = new ArrayList();
return this.linkId;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setLinkId(List theLinkId) {
this.linkId = theLinkId;
return this;
}
public boolean hasLinkId() {
if (this.linkId == null)
return false;
for (StringType item : this.linkId)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #linkId} (Id [identifier??] of the clause or question text
* about the asset in the referenced form or QuestionnaireResponse.)
*/
public StringType addLinkIdElement() {// 2
StringType t = new StringType();
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return t;
}
/**
* @param value {@link #linkId} (Id [identifier??] of the clause or question
* text about the asset in the referenced form or
* QuestionnaireResponse.)
*/
public ContractAssetComponent addLinkId(String value) { // 1
StringType t = new StringType();
t.setValue(value);
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return this;
}
/**
* @param value {@link #linkId} (Id [identifier??] of the clause or question
* text about the asset in the referenced form or
* QuestionnaireResponse.)
*/
public boolean hasLinkId(String value) {
if (this.linkId == null)
return false;
for (StringType v : this.linkId)
if (v.getValue().equals(value)) // string
return true;
return false;
}
/**
* @return {@link #answer} (Response to assets.)
*/
public List getAnswer() {
if (this.answer == null)
this.answer = new ArrayList();
return this.answer;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setAnswer(List theAnswer) {
this.answer = theAnswer;
return this;
}
public boolean hasAnswer() {
if (this.answer == null)
return false;
for (AnswerComponent item : this.answer)
if (!item.isEmpty())
return true;
return false;
}
public AnswerComponent addAnswer() { // 3
AnswerComponent t = new AnswerComponent();
if (this.answer == null)
this.answer = new ArrayList();
this.answer.add(t);
return t;
}
public ContractAssetComponent addAnswer(AnswerComponent t) { // 3
if (t == null)
return this;
if (this.answer == null)
this.answer = new ArrayList();
this.answer.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #answer}, creating it
* if it does not already exist
*/
public AnswerComponent getAnswerFirstRep() {
if (getAnswer().isEmpty()) {
addAnswer();
}
return getAnswer().get(0);
}
/**
* @return {@link #securityLabelNumber} (Security labels that protects the
* asset.)
*/
public List getSecurityLabelNumber() {
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
return this.securityLabelNumber;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setSecurityLabelNumber(List theSecurityLabelNumber) {
this.securityLabelNumber = theSecurityLabelNumber;
return this;
}
public boolean hasSecurityLabelNumber() {
if (this.securityLabelNumber == null)
return false;
for (UnsignedIntType item : this.securityLabelNumber)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #securityLabelNumber} (Security labels that protects the
* asset.)
*/
public UnsignedIntType addSecurityLabelNumberElement() {// 2
UnsignedIntType t = new UnsignedIntType();
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
this.securityLabelNumber.add(t);
return t;
}
/**
* @param value {@link #securityLabelNumber} (Security labels that protects the
* asset.)
*/
public ContractAssetComponent addSecurityLabelNumber(int value) { // 1
UnsignedIntType t = new UnsignedIntType();
t.setValue(value);
if (this.securityLabelNumber == null)
this.securityLabelNumber = new ArrayList();
this.securityLabelNumber.add(t);
return this;
}
/**
* @param value {@link #securityLabelNumber} (Security labels that protects the
* asset.)
*/
public boolean hasSecurityLabelNumber(int value) {
if (this.securityLabelNumber == null)
return false;
for (UnsignedIntType v : this.securityLabelNumber)
if (v.getValue().equals(value)) // unsignedInt
return true;
return false;
}
/**
* @return {@link #valuedItem} (Contract Valued Item List.)
*/
public List getValuedItem() {
if (this.valuedItem == null)
this.valuedItem = new ArrayList();
return this.valuedItem;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ContractAssetComponent setValuedItem(List theValuedItem) {
this.valuedItem = theValuedItem;
return this;
}
public boolean hasValuedItem() {
if (this.valuedItem == null)
return false;
for (ValuedItemComponent item : this.valuedItem)
if (!item.isEmpty())
return true;
return false;
}
public ValuedItemComponent addValuedItem() { // 3
ValuedItemComponent t = new ValuedItemComponent();
if (this.valuedItem == null)
this.valuedItem = new ArrayList();
this.valuedItem.add(t);
return t;
}
public ContractAssetComponent addValuedItem(ValuedItemComponent t) { // 3
if (t == null)
return this;
if (this.valuedItem == null)
this.valuedItem = new ArrayList();
this.valuedItem.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #valuedItem}, creating
* it if it does not already exist
*/
public ValuedItemComponent getValuedItemFirstRep() {
if (getValuedItem().isEmpty()) {
addValuedItem();
}
return getValuedItem().get(0);
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, scope));
children.add(new Property("type", "CodeableConcept", "Target entity type about which the term may be concerned.",
0, java.lang.Integer.MAX_VALUE, type));
children.add(new Property("typeReference", "Reference(Any)", "Associated entities.", 0,
java.lang.Integer.MAX_VALUE, typeReference));
children.add(new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 0,
java.lang.Integer.MAX_VALUE, subtype));
children.add(new Property("relationship", "Coding",
"Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.",
0, 1, relationship));
children.add(new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, context));
children.add(new Property("condition", "string",
"Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1,
condition));
children.add(new Property("periodType", "CodeableConcept", "Type of Asset availability for use or ownership.", 0,
java.lang.Integer.MAX_VALUE, periodType));
children.add(new Property("period", "Period", "Asset relevant contractual time period.", 0,
java.lang.Integer.MAX_VALUE, period));
children.add(
new Property("usePeriod", "Period", "Time period of asset use.", 0, java.lang.Integer.MAX_VALUE, usePeriod));
children.add(new Property("text", "string",
"Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.",
0, 1, text));
children.add(new Property("linkId", "string",
"Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.",
0, java.lang.Integer.MAX_VALUE, linkId));
children.add(new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0,
java.lang.Integer.MAX_VALUE, answer));
children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the asset.", 0,
java.lang.Integer.MAX_VALUE, securityLabelNumber));
children.add(
new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case 109264468:
/* scope */ return new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1,
scope);
case 3575610:
/* type */ return new Property("type", "CodeableConcept",
"Target entity type about which the term may be concerned.", 0, java.lang.Integer.MAX_VALUE, type);
case 2074825009:
/* typeReference */ return new Property("typeReference", "Reference(Any)", "Associated entities.", 0,
java.lang.Integer.MAX_VALUE, typeReference);
case -1867567750:
/* subtype */ return new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.",
0, java.lang.Integer.MAX_VALUE, subtype);
case -261851592:
/* relationship */ return new Property("relationship", "Coding",
"Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.",
0, 1, relationship);
case 951530927:
/* context */ return new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE,
context);
case -861311717:
/* condition */ return new Property("condition", "string",
"Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1,
condition);
case 384348315:
/* periodType */ return new Property("periodType", "CodeableConcept",
"Type of Asset availability for use or ownership.", 0, java.lang.Integer.MAX_VALUE, periodType);
case -991726143:
/* period */ return new Property("period", "Period", "Asset relevant contractual time period.", 0,
java.lang.Integer.MAX_VALUE, period);
case -628382168:
/* usePeriod */ return new Property("usePeriod", "Period", "Time period of asset use.", 0,
java.lang.Integer.MAX_VALUE, usePeriod);
case 3556653:
/* text */ return new Property("text", "string",
"Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.",
0, 1, text);
case -1102667083:
/* linkId */ return new Property("linkId", "string",
"Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.",
0, java.lang.Integer.MAX_VALUE, linkId);
case -1412808770:
/* answer */ return new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0,
java.lang.Integer.MAX_VALUE, answer);
case -149460995:
/* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt",
"Security labels that protects the asset.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber);
case 2046675654:
/* valuedItem */ return new Property("valuedItem", "", "Contract Valued Item List.", 0,
java.lang.Integer.MAX_VALUE, valuedItem);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case 109264468:
/* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept
case 3575610:
/* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept
case 2074825009:
/* typeReference */ return this.typeReference == null ? new Base[0]
: this.typeReference.toArray(new Base[this.typeReference.size()]); // Reference
case -1867567750:
/* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept
case -261851592:
/* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Coding
case 951530927:
/* context */ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // AssetContextComponent
case -861311717:
/* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // StringType
case 384348315:
/* periodType */ return this.periodType == null ? new Base[0]
: this.periodType.toArray(new Base[this.periodType.size()]); // CodeableConcept
case -991726143:
/* period */ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period
case -628382168:
/* usePeriod */ return this.usePeriod == null ? new Base[0]
: this.usePeriod.toArray(new Base[this.usePeriod.size()]); // Period
case 3556653:
/* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType
case -1102667083:
/* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType
case -1412808770:
/* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent
case -149460995:
/* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0]
: this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType
case 2046675654:
/* valuedItem */ return this.valuedItem == null ? new Base[0]
: this.valuedItem.toArray(new Base[this.valuedItem.size()]); // ValuedItemComponent
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case 109264468: // scope
this.scope = castToCodeableConcept(value); // CodeableConcept
return value;
case 3575610: // type
this.getType().add(castToCodeableConcept(value)); // CodeableConcept
return value;
case 2074825009: // typeReference
this.getTypeReference().add(castToReference(value)); // Reference
return value;
case -1867567750: // subtype
this.getSubtype().add(castToCodeableConcept(value)); // CodeableConcept
return value;
case -261851592: // relationship
this.relationship = castToCoding(value); // Coding
return value;
case 951530927: // context
this.getContext().add((AssetContextComponent) value); // AssetContextComponent
return value;
case -861311717: // condition
this.condition = castToString(value); // StringType
return value;
case 384348315: // periodType
this.getPeriodType().add(castToCodeableConcept(value)); // CodeableConcept
return value;
case -991726143: // period
this.getPeriod().add(castToPeriod(value)); // Period
return value;
case -628382168: // usePeriod
this.getUsePeriod().add(castToPeriod(value)); // Period
return value;
case 3556653: // text
this.text = castToString(value); // StringType
return value;
case -1102667083: // linkId
this.getLinkId().add(castToString(value)); // StringType
return value;
case -1412808770: // answer
this.getAnswer().add((AnswerComponent) value); // AnswerComponent
return value;
case -149460995: // securityLabelNumber
this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType
return value;
case 2046675654: // valuedItem
this.getValuedItem().add((ValuedItemComponent) value); // ValuedItemComponent
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("scope")) {
this.scope = castToCodeableConcept(value); // CodeableConcept
} else if (name.equals("type")) {
this.getType().add(castToCodeableConcept(value));
} else if (name.equals("typeReference")) {
this.getTypeReference().add(castToReference(value));
} else if (name.equals("subtype")) {
this.getSubtype().add(castToCodeableConcept(value));
} else if (name.equals("relationship")) {
this.relationship = castToCoding(value); // Coding
} else if (name.equals("context")) {
this.getContext().add((AssetContextComponent) value);
} else if (name.equals("condition")) {
this.condition = castToString(value); // StringType
} else if (name.equals("periodType")) {
this.getPeriodType().add(castToCodeableConcept(value));
} else if (name.equals("period")) {
this.getPeriod().add(castToPeriod(value));
} else if (name.equals("usePeriod")) {
this.getUsePeriod().add(castToPeriod(value));
} else if (name.equals("text")) {
this.text = castToString(value); // StringType
} else if (name.equals("linkId")) {
this.getLinkId().add(castToString(value));
} else if (name.equals("answer")) {
this.getAnswer().add((AnswerComponent) value);
} else if (name.equals("securityLabelNumber")) {
this.getSecurityLabelNumber().add(castToUnsignedInt(value));
} else if (name.equals("valuedItem")) {
this.getValuedItem().add((ValuedItemComponent) value);
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("scope")) {
this.scope = null;
} else if (name.equals("type")) {
this.getType().remove(castToCodeableConcept(value));
} else if (name.equals("typeReference")) {
this.getTypeReference().remove(castToReference(value));
} else if (name.equals("subtype")) {
this.getSubtype().remove(castToCodeableConcept(value));
} else if (name.equals("relationship")) {
this.relationship = null;
} else if (name.equals("context")) {
this.getContext().remove((AssetContextComponent) value);
} else if (name.equals("condition")) {
this.condition = null;
} else if (name.equals("periodType")) {
this.getPeriodType().remove(castToCodeableConcept(value));
} else if (name.equals("period")) {
this.getPeriod().remove(castToPeriod(value));
} else if (name.equals("usePeriod")) {
this.getUsePeriod().remove(castToPeriod(value));
} else if (name.equals("text")) {
this.text = null;
} else if (name.equals("linkId")) {
this.getLinkId().remove(castToString(value));
} else if (name.equals("answer")) {
this.getAnswer().remove((AnswerComponent) value);
} else if (name.equals("securityLabelNumber")) {
this.getSecurityLabelNumber().remove(castToUnsignedInt(value));
} else if (name.equals("valuedItem")) {
this.getValuedItem().remove((ValuedItemComponent) value);
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case 109264468:
return getScope();
case 3575610:
return addType();
case 2074825009:
return addTypeReference();
case -1867567750:
return addSubtype();
case -261851592:
return getRelationship();
case 951530927:
return addContext();
case -861311717:
return getConditionElement();
case 384348315:
return addPeriodType();
case -991726143:
return addPeriod();
case -628382168:
return addUsePeriod();
case 3556653:
return getTextElement();
case -1102667083:
return addLinkIdElement();
case -1412808770:
return addAnswer();
case -149460995:
return addSecurityLabelNumberElement();
case 2046675654:
return addValuedItem();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case 109264468:
/* scope */ return new String[] { "CodeableConcept" };
case 3575610:
/* type */ return new String[] { "CodeableConcept" };
case 2074825009:
/* typeReference */ return new String[] { "Reference" };
case -1867567750:
/* subtype */ return new String[] { "CodeableConcept" };
case -261851592:
/* relationship */ return new String[] { "Coding" };
case 951530927:
/* context */ return new String[] {};
case -861311717:
/* condition */ return new String[] { "string" };
case 384348315:
/* periodType */ return new String[] { "CodeableConcept" };
case -991726143:
/* period */ return new String[] { "Period" };
case -628382168:
/* usePeriod */ return new String[] { "Period" };
case 3556653:
/* text */ return new String[] { "string" };
case -1102667083:
/* linkId */ return new String[] { "string" };
case -1412808770:
/* answer */ return new String[] { "@Contract.term.offer.answer" };
case -149460995:
/* securityLabelNumber */ return new String[] { "unsignedInt" };
case 2046675654:
/* valuedItem */ return new String[] {};
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("scope")) {
this.scope = new CodeableConcept();
return this.scope;
} else if (name.equals("type")) {
return addType();
} else if (name.equals("typeReference")) {
return addTypeReference();
} else if (name.equals("subtype")) {
return addSubtype();
} else if (name.equals("relationship")) {
this.relationship = new Coding();
return this.relationship;
} else if (name.equals("context")) {
return addContext();
} else if (name.equals("condition")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.condition");
} else if (name.equals("periodType")) {
return addPeriodType();
} else if (name.equals("period")) {
return addPeriod();
} else if (name.equals("usePeriod")) {
return addUsePeriod();
} else if (name.equals("text")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.text");
} else if (name.equals("linkId")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId");
} else if (name.equals("answer")) {
return addAnswer();
} else if (name.equals("securityLabelNumber")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber");
} else if (name.equals("valuedItem")) {
return addValuedItem();
} else
return super.addChild(name);
}
public ContractAssetComponent copy() {
ContractAssetComponent dst = new ContractAssetComponent();
copyValues(dst);
return dst;
}
public void copyValues(ContractAssetComponent dst) {
super.copyValues(dst);
dst.scope = scope == null ? null : scope.copy();
if (type != null) {
dst.type = new ArrayList();
for (CodeableConcept i : type)
dst.type.add(i.copy());
}
;
if (typeReference != null) {
dst.typeReference = new ArrayList();
for (Reference i : typeReference)
dst.typeReference.add(i.copy());
}
;
if (subtype != null) {
dst.subtype = new ArrayList();
for (CodeableConcept i : subtype)
dst.subtype.add(i.copy());
}
;
dst.relationship = relationship == null ? null : relationship.copy();
if (context != null) {
dst.context = new ArrayList();
for (AssetContextComponent i : context)
dst.context.add(i.copy());
}
;
dst.condition = condition == null ? null : condition.copy();
if (periodType != null) {
dst.periodType = new ArrayList();
for (CodeableConcept i : periodType)
dst.periodType.add(i.copy());
}
;
if (period != null) {
dst.period = new ArrayList();
for (Period i : period)
dst.period.add(i.copy());
}
;
if (usePeriod != null) {
dst.usePeriod = new ArrayList();
for (Period i : usePeriod)
dst.usePeriod.add(i.copy());
}
;
dst.text = text == null ? null : text.copy();
if (linkId != null) {
dst.linkId = new ArrayList();
for (StringType i : linkId)
dst.linkId.add(i.copy());
}
;
if (answer != null) {
dst.answer = new ArrayList();
for (AnswerComponent i : answer)
dst.answer.add(i.copy());
}
;
if (securityLabelNumber != null) {
dst.securityLabelNumber = new ArrayList();
for (UnsignedIntType i : securityLabelNumber)
dst.securityLabelNumber.add(i.copy());
}
;
if (valuedItem != null) {
dst.valuedItem = new ArrayList();
for (ValuedItemComponent i : valuedItem)
dst.valuedItem.add(i.copy());
}
;
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof ContractAssetComponent))
return false;
ContractAssetComponent o = (ContractAssetComponent) other_;
return compareDeep(scope, o.scope, true) && compareDeep(type, o.type, true)
&& compareDeep(typeReference, o.typeReference, true) && compareDeep(subtype, o.subtype, true)
&& compareDeep(relationship, o.relationship, true) && compareDeep(context, o.context, true)
&& compareDeep(condition, o.condition, true) && compareDeep(periodType, o.periodType, true)
&& compareDeep(period, o.period, true) && compareDeep(usePeriod, o.usePeriod, true)
&& compareDeep(text, o.text, true) && compareDeep(linkId, o.linkId, true)
&& compareDeep(answer, o.answer, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true)
&& compareDeep(valuedItem, o.valuedItem, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof ContractAssetComponent))
return false;
ContractAssetComponent o = (ContractAssetComponent) other_;
return compareValues(condition, o.condition, true) && compareValues(text, o.text, true)
&& compareValues(linkId, o.linkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(scope, type, typeReference, subtype, relationship,
context, condition, periodType, period, usePeriod, text, linkId, answer, securityLabelNumber, valuedItem);
}
public String fhirType() {
return "Contract.term.asset";
}
}
@Block()
public static class AssetContextComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Asset context reference may include the creator, custodian, or owning Person
* or Organization (e.g., bank, repository), location held, e.g., building,
* jurisdiction.
*/
@Child(name = "reference", type = {
Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Creator,custodian or owner", formalDefinition = "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.")
protected Reference reference;
/**
* The actual object that is the target of the reference (Asset context
* reference may include the creator, custodian, or owning Person or
* Organization (e.g., bank, repository), location held, e.g., building,
* jurisdiction.)
*/
protected Resource referenceTarget;
/**
* Coded representation of the context generally or of the Referenced entity,
* such as the asset holder type or location.
*/
@Child(name = "code", type = {
CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Codeable asset context", formalDefinition = "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.")
@ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetcontext")
protected List code;
/**
* Context description.
*/
@Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Context description", formalDefinition = "Context description.")
protected StringType text;
private static final long serialVersionUID = -634115628L;
/**
* Constructor
*/
public AssetContextComponent() {
super();
}
/**
* @return {@link #reference} (Asset context reference may include the creator,
* custodian, or owning Person or Organization (e.g., bank, repository),
* location held, e.g., building, jurisdiction.)
*/
public Reference getReference() {
if (this.reference == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create AssetContextComponent.reference");
else if (Configuration.doAutoCreate())
this.reference = new Reference(); // cc
return this.reference;
}
public boolean hasReference() {
return this.reference != null && !this.reference.isEmpty();
}
/**
* @param value {@link #reference} (Asset context reference may include the
* creator, custodian, or owning Person or Organization (e.g.,
* bank, repository), location held, e.g., building, jurisdiction.)
*/
public AssetContextComponent setReference(Reference value) {
this.reference = value;
return this;
}
/**
* @return {@link #reference} The actual object that is the target of the
* reference. The reference library doesn't populate this, but you can
* use it to hold the resource if you resolve it. (Asset context
* reference may include the creator, custodian, or owning Person or
* Organization (e.g., bank, repository), location held, e.g., building,
* jurisdiction.)
*/
public Resource getReferenceTarget() {
return this.referenceTarget;
}
/**
* @param value {@link #reference} The actual object that is the target of the
* reference. The reference library doesn't use these, but you can
* use it to hold the resource if you resolve it. (Asset context
* reference may include the creator, custodian, or owning Person
* or Organization (e.g., bank, repository), location held, e.g.,
* building, jurisdiction.)
*/
public AssetContextComponent setReferenceTarget(Resource value) {
this.referenceTarget = value;
return this;
}
/**
* @return {@link #code} (Coded representation of the context generally or of
* the Referenced entity, such as the asset holder type or location.)
*/
public List getCode() {
if (this.code == null)
this.code = new ArrayList();
return this.code;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public AssetContextComponent setCode(List theCode) {
this.code = theCode;
return this;
}
public boolean hasCode() {
if (this.code == null)
return false;
for (CodeableConcept item : this.code)
if (!item.isEmpty())
return true;
return false;
}
public CodeableConcept addCode() { // 3
CodeableConcept t = new CodeableConcept();
if (this.code == null)
this.code = new ArrayList();
this.code.add(t);
return t;
}
public AssetContextComponent addCode(CodeableConcept t) { // 3
if (t == null)
return this;
if (this.code == null)
this.code = new ArrayList();
this.code.add(t);
return this;
}
/**
* @return The first repetition of repeating field {@link #code}, creating it if
* it does not already exist
*/
public CodeableConcept getCodeFirstRep() {
if (getCode().isEmpty()) {
addCode();
}
return getCode().get(0);
}
/**
* @return {@link #text} (Context description.). This is the underlying object
* with id, value and extensions. The accessor "getText" gives direct
* access to the value
*/
public StringType getTextElement() {
if (this.text == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create AssetContextComponent.text");
else if (Configuration.doAutoCreate())
this.text = new StringType(); // bb
return this.text;
}
public boolean hasTextElement() {
return this.text != null && !this.text.isEmpty();
}
public boolean hasText() {
return this.text != null && !this.text.isEmpty();
}
/**
* @param value {@link #text} (Context description.). This is the underlying
* object with id, value and extensions. The accessor "getText"
* gives direct access to the value
*/
public AssetContextComponent setTextElement(StringType value) {
this.text = value;
return this;
}
/**
* @return Context description.
*/
public String getText() {
return this.text == null ? null : this.text.getValue();
}
/**
* @param value Context description.
*/
public AssetContextComponent setText(String value) {
if (Utilities.noString(value))
this.text = null;
else {
if (this.text == null)
this.text = new StringType();
this.text.setValue(value);
}
return this;
}
protected void listChildren(List children) {
super.listChildren(children);
children.add(new Property("reference", "Reference(Any)",
"Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.",
0, 1, reference));
children.add(new Property("code", "CodeableConcept",
"Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.",
0, java.lang.Integer.MAX_VALUE, code));
children.add(new Property("text", "string", "Context description.", 0, 1, text));
}
@Override
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException {
switch (_hash) {
case -925155509:
/* reference */ return new Property("reference", "Reference(Any)",
"Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.",
0, 1, reference);
case 3059181:
/* code */ return new Property("code", "CodeableConcept",
"Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.",
0, java.lang.Integer.MAX_VALUE, code);
case 3556653:
/* text */ return new Property("text", "string", "Context description.", 0, 1, text);
default:
return super.getNamedProperty(_hash, _name, _checkValid);
}
}
@Override
public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException {
switch (hash) {
case -925155509:
/* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference
case 3059181:
/* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept
case 3556653:
/* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType
default:
return super.getProperty(hash, name, checkValid);
}
}
@Override
public Base setProperty(int hash, String name, Base value) throws FHIRException {
switch (hash) {
case -925155509: // reference
this.reference = castToReference(value); // Reference
return value;
case 3059181: // code
this.getCode().add(castToCodeableConcept(value)); // CodeableConcept
return value;
case 3556653: // text
this.text = castToString(value); // StringType
return value;
default:
return super.setProperty(hash, name, value);
}
}
@Override
public Base setProperty(String name, Base value) throws FHIRException {
if (name.equals("reference")) {
this.reference = castToReference(value); // Reference
} else if (name.equals("code")) {
this.getCode().add(castToCodeableConcept(value));
} else if (name.equals("text")) {
this.text = castToString(value); // StringType
} else
return super.setProperty(name, value);
return value;
}
@Override
public void removeChild(String name, Base value) throws FHIRException {
if (name.equals("reference")) {
this.reference = null;
} else if (name.equals("code")) {
this.getCode().remove(castToCodeableConcept(value));
} else if (name.equals("text")) {
this.text = null;
} else
super.removeChild(name, value);
}
@Override
public Base makeProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -925155509:
return getReference();
case 3059181:
return addCode();
case 3556653:
return getTextElement();
default:
return super.makeProperty(hash, name);
}
}
@Override
public String[] getTypesForProperty(int hash, String name) throws FHIRException {
switch (hash) {
case -925155509:
/* reference */ return new String[] { "Reference" };
case 3059181:
/* code */ return new String[] { "CodeableConcept" };
case 3556653:
/* text */ return new String[] { "string" };
default:
return super.getTypesForProperty(hash, name);
}
}
@Override
public Base addChild(String name) throws FHIRException {
if (name.equals("reference")) {
this.reference = new Reference();
return this.reference;
} else if (name.equals("code")) {
return addCode();
} else if (name.equals("text")) {
throw new FHIRException("Cannot call addChild on a singleton property Contract.text");
} else
return super.addChild(name);
}
public AssetContextComponent copy() {
AssetContextComponent dst = new AssetContextComponent();
copyValues(dst);
return dst;
}
public void copyValues(AssetContextComponent dst) {
super.copyValues(dst);
dst.reference = reference == null ? null : reference.copy();
if (code != null) {
dst.code = new ArrayList();
for (CodeableConcept i : code)
dst.code.add(i.copy());
}
;
dst.text = text == null ? null : text.copy();
}
@Override
public boolean equalsDeep(Base other_) {
if (!super.equalsDeep(other_))
return false;
if (!(other_ instanceof AssetContextComponent))
return false;
AssetContextComponent o = (AssetContextComponent) other_;
return compareDeep(reference, o.reference, true) && compareDeep(code, o.code, true)
&& compareDeep(text, o.text, true);
}
@Override
public boolean equalsShallow(Base other_) {
if (!super.equalsShallow(other_))
return false;
if (!(other_ instanceof AssetContextComponent))
return false;
AssetContextComponent o = (AssetContextComponent) other_;
return compareValues(text, o.text, true);
}
public boolean isEmpty() {
return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, code, text);
}
public String fhirType() {
return "Contract.term.asset.context";
}
}
@Block()
public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement {
/**
* Specific type of Contract Valued Item that may be priced.
*/
@Child(name = "entity", type = { CodeableConcept.class,
Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item Type", formalDefinition = "Specific type of Contract Valued Item that may be priced.")
protected Type entity;
/**
* Identifies a Contract Valued Item instance.
*/
@Child(name = "identifier", type = {
Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item Number", formalDefinition = "Identifies a Contract Valued Item instance.")
protected Identifier identifier;
/**
* Indicates the time during which this Contract ValuedItem information is
* effective.
*/
@Child(name = "effectiveTime", type = {
DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item Effective Tiem", formalDefinition = "Indicates the time during which this Contract ValuedItem information is effective.")
protected DateTimeType effectiveTime;
/**
* Specifies the units by which the Contract Valued Item is measured or counted,
* and quantifies the countable or measurable Contract Valued Item instances.
*/
@Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Count of Contract Valued Items", formalDefinition = "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.")
protected Quantity quantity;
/**
* A Contract Valued Item unit valuation measure.
*/
@Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item fee, charge, or cost", formalDefinition = "A Contract Valued Item unit valuation measure.")
protected Money unitPrice;
/**
* A real number that represents a multiplier used in determining the overall
* value of the Contract Valued Item delivered. The concept of a Factor allows
* for a discount or surcharge multiplier to be applied to a monetary amount.
*/
@Child(name = "factor", type = {
DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item Price Scaling Factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.")
protected DecimalType factor;
/**
* An amount that expresses the weighting (based on difficulty, cost and/or
* resource intensiveness) associated with the Contract Valued Item delivered.
* The concept of Points allows for assignment of point values for a Contract
* Valued Item, such that a monetary amount can be assigned to each point.
*/
@Child(name = "points", type = {
DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Contract Valued Item Difficulty Scaling Factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.")
protected DecimalType points;
/**
* Expresses the product of the Contract Valued Item unitQuantity and the
* unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per
* Point) * factor Number * points = net Amount. Quantity, factor and points are
* assumed to be 1 if not supplied.
*/
@Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Total Contract Valued Item Value", formalDefinition = "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.")
protected Money net;
/**
* Terms of valuation.
*/
@Child(name = "payment", type = {
StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Terms of valuation", formalDefinition = "Terms of valuation.")
protected StringType payment;
/**
* When payment is due.
*/
@Child(name = "paymentDate", type = {
DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "When payment is due", formalDefinition = "When payment is due.")
protected DateTimeType paymentDate;
/**
* Who will make payment.
*/
@Child(name = "responsible", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class,
RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Who will make payment", formalDefinition = "Who will make payment.")
protected Reference responsible;
/**
* The actual object that is the target of the reference (Who will make
* payment.)
*/
protected Resource responsibleTarget;
/**
* Who will receive payment.
*/
@Child(name = "recipient", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class,
RelatedPerson.class }, order = 12, min = 0, max = 1, modifier = false, summary = false)
@Description(shortDefinition = "Who will receive payment", formalDefinition = "Who will receive payment.")
protected Reference recipient;
/**
* The actual object that is the target of the reference (Who will receive
* payment.)
*/
protected Resource recipientTarget;
/**
* Id of the clause or question text related to the context of this valuedItem
* in the referenced form or QuestionnaireResponse.
*/
@Child(name = "linkId", type = {
StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.")
protected List linkId;
/**
* A set of security labels that define which terms are controlled by this
* condition.
*/
@Child(name = "securityLabelNumber", type = {
UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false)
@Description(shortDefinition = "Security Labels that define affected terms", formalDefinition = "A set of security labels that define which terms are controlled by this condition.")
protected List securityLabelNumber;
private static final long serialVersionUID = 1894951601L;
/**
* Constructor
*/
public ValuedItemComponent() {
super();
}
/**
* @return {@link #entity} (Specific type of Contract Valued Item that may be
* priced.)
*/
public Type getEntity() {
return this.entity;
}
/**
* @return {@link #entity} (Specific type of Contract Valued Item that may be
* priced.)
*/
public CodeableConcept getEntityCodeableConcept() throws FHIRException {
if (this.entity == null)
this.entity = new CodeableConcept();
if (!(this.entity instanceof CodeableConcept))
throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "
+ this.entity.getClass().getName() + " was encountered");
return (CodeableConcept) this.entity;
}
public boolean hasEntityCodeableConcept() {
return this != null && this.entity instanceof CodeableConcept;
}
/**
* @return {@link #entity} (Specific type of Contract Valued Item that may be
* priced.)
*/
public Reference getEntityReference() throws FHIRException {
if (this.entity == null)
this.entity = new Reference();
if (!(this.entity instanceof Reference))
throw new FHIRException("Type mismatch: the type Reference was expected, but "
+ this.entity.getClass().getName() + " was encountered");
return (Reference) this.entity;
}
public boolean hasEntityReference() {
return this != null && this.entity instanceof Reference;
}
public boolean hasEntity() {
return this.entity != null && !this.entity.isEmpty();
}
/**
* @param value {@link #entity} (Specific type of Contract Valued Item that may
* be priced.)
*/
public ValuedItemComponent setEntity(Type value) {
if (value != null && !(value instanceof CodeableConcept || value instanceof Reference))
throw new Error("Not the right type for Contract.term.asset.valuedItem.entity[x]: " + value.fhirType());
this.entity = value;
return this;
}
/**
* @return {@link #identifier} (Identifies a Contract Valued Item instance.)
*/
public Identifier getIdentifier() {
if (this.identifier == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.identifier");
else if (Configuration.doAutoCreate())
this.identifier = new Identifier(); // cc
return this.identifier;
}
public boolean hasIdentifier() {
return this.identifier != null && !this.identifier.isEmpty();
}
/**
* @param value {@link #identifier} (Identifies a Contract Valued Item
* instance.)
*/
public ValuedItemComponent setIdentifier(Identifier value) {
this.identifier = value;
return this;
}
/**
* @return {@link #effectiveTime} (Indicates the time during which this Contract
* ValuedItem information is effective.). This is the underlying object
* with id, value and extensions. The accessor "getEffectiveTime" gives
* direct access to the value
*/
public DateTimeType getEffectiveTimeElement() {
if (this.effectiveTime == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime");
else if (Configuration.doAutoCreate())
this.effectiveTime = new DateTimeType(); // bb
return this.effectiveTime;
}
public boolean hasEffectiveTimeElement() {
return this.effectiveTime != null && !this.effectiveTime.isEmpty();
}
public boolean hasEffectiveTime() {
return this.effectiveTime != null && !this.effectiveTime.isEmpty();
}
/**
* @param value {@link #effectiveTime} (Indicates the time during which this
* Contract ValuedItem information is effective.). This is the
* underlying object with id, value and extensions. The accessor
* "getEffectiveTime" gives direct access to the value
*/
public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) {
this.effectiveTime = value;
return this;
}
/**
* @return Indicates the time during which this Contract ValuedItem information
* is effective.
*/
public Date getEffectiveTime() {
return this.effectiveTime == null ? null : this.effectiveTime.getValue();
}
/**
* @param value Indicates the time during which this Contract ValuedItem
* information is effective.
*/
public ValuedItemComponent setEffectiveTime(Date value) {
if (value == null)
this.effectiveTime = null;
else {
if (this.effectiveTime == null)
this.effectiveTime = new DateTimeType();
this.effectiveTime.setValue(value);
}
return this;
}
/**
* @return {@link #quantity} (Specifies the units by which the Contract Valued
* Item is measured or counted, and quantifies the countable or
* measurable Contract Valued Item instances.)
*/
public Quantity getQuantity() {
if (this.quantity == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.quantity");
else if (Configuration.doAutoCreate())
this.quantity = new Quantity(); // cc
return this.quantity;
}
public boolean hasQuantity() {
return this.quantity != null && !this.quantity.isEmpty();
}
/**
* @param value {@link #quantity} (Specifies the units by which the Contract
* Valued Item is measured or counted, and quantifies the countable
* or measurable Contract Valued Item instances.)
*/
public ValuedItemComponent setQuantity(Quantity value) {
this.quantity = value;
return this;
}
/**
* @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.)
*/
public Money getUnitPrice() {
if (this.unitPrice == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice");
else if (Configuration.doAutoCreate())
this.unitPrice = new Money(); // cc
return this.unitPrice;
}
public boolean hasUnitPrice() {
return this.unitPrice != null && !this.unitPrice.isEmpty();
}
/**
* @param value {@link #unitPrice} (A Contract Valued Item unit valuation
* measure.)
*/
public ValuedItemComponent setUnitPrice(Money value) {
this.unitPrice = value;
return this;
}
/**
* @return {@link #factor} (A real number that represents a multiplier used in
* determining the overall value of the Contract Valued Item delivered.
* The concept of a Factor allows for a discount or surcharge multiplier
* to be applied to a monetary amount.). This is the underlying object
* with id, value and extensions. The accessor "getFactor" gives direct
* access to the value
*/
public DecimalType getFactorElement() {
if (this.factor == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.factor");
else if (Configuration.doAutoCreate())
this.factor = new DecimalType(); // bb
return this.factor;
}
public boolean hasFactorElement() {
return this.factor != null && !this.factor.isEmpty();
}
public boolean hasFactor() {
return this.factor != null && !this.factor.isEmpty();
}
/**
* @param value {@link #factor} (A real number that represents a multiplier used
* in determining the overall value of the Contract Valued Item
* delivered. The concept of a Factor allows for a discount or
* surcharge multiplier to be applied to a monetary amount.). This
* is the underlying object with id, value and extensions. The
* accessor "getFactor" gives direct access to the value
*/
public ValuedItemComponent setFactorElement(DecimalType value) {
this.factor = value;
return this;
}
/**
* @return A real number that represents a multiplier used in determining the
* overall value of the Contract Valued Item delivered. The concept of a
* Factor allows for a discount or surcharge multiplier to be applied to
* a monetary amount.
*/
public BigDecimal getFactor() {
return this.factor == null ? null : this.factor.getValue();
}
/**
* @param value A real number that represents a multiplier used in determining
* the overall value of the Contract Valued Item delivered. The
* concept of a Factor allows for a discount or surcharge
* multiplier to be applied to a monetary amount.
*/
public ValuedItemComponent setFactor(BigDecimal value) {
if (value == null)
this.factor = null;
else {
if (this.factor == null)
this.factor = new DecimalType();
this.factor.setValue(value);
}
return this;
}
/**
* @param value A real number that represents a multiplier used in determining
* the overall value of the Contract Valued Item delivered. The
* concept of a Factor allows for a discount or surcharge
* multiplier to be applied to a monetary amount.
*/
public ValuedItemComponent setFactor(long value) {
this.factor = new DecimalType();
this.factor.setValue(value);
return this;
}
/**
* @param value A real number that represents a multiplier used in determining
* the overall value of the Contract Valued Item delivered. The
* concept of a Factor allows for a discount or surcharge
* multiplier to be applied to a monetary amount.
*/
public ValuedItemComponent setFactor(double value) {
this.factor = new DecimalType();
this.factor.setValue(value);
return this;
}
/**
* @return {@link #points} (An amount that expresses the weighting (based on
* difficulty, cost and/or resource intensiveness) associated with the
* Contract Valued Item delivered. The concept of Points allows for
* assignment of point values for a Contract Valued Item, such that a
* monetary amount can be assigned to each point.). This is the
* underlying object with id, value and extensions. The accessor
* "getPoints" gives direct access to the value
*/
public DecimalType getPointsElement() {
if (this.points == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.points");
else if (Configuration.doAutoCreate())
this.points = new DecimalType(); // bb
return this.points;
}
public boolean hasPointsElement() {
return this.points != null && !this.points.isEmpty();
}
public boolean hasPoints() {
return this.points != null && !this.points.isEmpty();
}
/**
* @param value {@link #points} (An amount that expresses the weighting (based
* on difficulty, cost and/or resource intensiveness) associated
* with the Contract Valued Item delivered. The concept of Points
* allows for assignment of point values for a Contract Valued
* Item, such that a monetary amount can be assigned to each
* point.). This is the underlying object with id, value and
* extensions. The accessor "getPoints" gives direct access to the
* value
*/
public ValuedItemComponent setPointsElement(DecimalType value) {
this.points = value;
return this;
}
/**
* @return An amount that expresses the weighting (based on difficulty, cost
* and/or resource intensiveness) associated with the Contract Valued
* Item delivered. The concept of Points allows for assignment of point
* values for a Contract Valued Item, such that a monetary amount can be
* assigned to each point.
*/
public BigDecimal getPoints() {
return this.points == null ? null : this.points.getValue();
}
/**
* @param value An amount that expresses the weighting (based on difficulty,
* cost and/or resource intensiveness) associated with the Contract
* Valued Item delivered. The concept of Points allows for
* assignment of point values for a Contract Valued Item, such that
* a monetary amount can be assigned to each point.
*/
public ValuedItemComponent setPoints(BigDecimal value) {
if (value == null)
this.points = null;
else {
if (this.points == null)
this.points = new DecimalType();
this.points.setValue(value);
}
return this;
}
/**
* @param value An amount that expresses the weighting (based on difficulty,
* cost and/or resource intensiveness) associated with the Contract
* Valued Item delivered. The concept of Points allows for
* assignment of point values for a Contract Valued Item, such that
* a monetary amount can be assigned to each point.
*/
public ValuedItemComponent setPoints(long value) {
this.points = new DecimalType();
this.points.setValue(value);
return this;
}
/**
* @param value An amount that expresses the weighting (based on difficulty,
* cost and/or resource intensiveness) associated with the Contract
* Valued Item delivered. The concept of Points allows for
* assignment of point values for a Contract Valued Item, such that
* a monetary amount can be assigned to each point.
*/
public ValuedItemComponent setPoints(double value) {
this.points = new DecimalType();
this.points.setValue(value);
return this;
}
/**
* @return {@link #net} (Expresses the product of the Contract Valued Item
* unitQuantity and the unitPriceAmt. For example, the formula: unit
* Quantity * unit Price (Cost per Point) * factor Number * points = net
* Amount. Quantity, factor and points are assumed to be 1 if not
* supplied.)
*/
public Money getNet() {
if (this.net == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.net");
else if (Configuration.doAutoCreate())
this.net = new Money(); // cc
return this.net;
}
public boolean hasNet() {
return this.net != null && !this.net.isEmpty();
}
/**
* @param value {@link #net} (Expresses the product of the Contract Valued Item
* unitQuantity and the unitPriceAmt. For example, the formula:
* unit Quantity * unit Price (Cost per Point) * factor Number *
* points = net Amount. Quantity, factor and points are assumed to
* be 1 if not supplied.)
*/
public ValuedItemComponent setNet(Money value) {
this.net = value;
return this;
}
/**
* @return {@link #payment} (Terms of valuation.). This is the underlying object
* with id, value and extensions. The accessor "getPayment" gives direct
* access to the value
*/
public StringType getPaymentElement() {
if (this.payment == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.payment");
else if (Configuration.doAutoCreate())
this.payment = new StringType(); // bb
return this.payment;
}
public boolean hasPaymentElement() {
return this.payment != null && !this.payment.isEmpty();
}
public boolean hasPayment() {
return this.payment != null && !this.payment.isEmpty();
}
/**
* @param value {@link #payment} (Terms of valuation.). This is the underlying
* object with id, value and extensions. The accessor "getPayment"
* gives direct access to the value
*/
public ValuedItemComponent setPaymentElement(StringType value) {
this.payment = value;
return this;
}
/**
* @return Terms of valuation.
*/
public String getPayment() {
return this.payment == null ? null : this.payment.getValue();
}
/**
* @param value Terms of valuation.
*/
public ValuedItemComponent setPayment(String value) {
if (Utilities.noString(value))
this.payment = null;
else {
if (this.payment == null)
this.payment = new StringType();
this.payment.setValue(value);
}
return this;
}
/**
* @return {@link #paymentDate} (When payment is due.). This is the underlying
* object with id, value and extensions. The accessor "getPaymentDate"
* gives direct access to the value
*/
public DateTimeType getPaymentDateElement() {
if (this.paymentDate == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.paymentDate");
else if (Configuration.doAutoCreate())
this.paymentDate = new DateTimeType(); // bb
return this.paymentDate;
}
public boolean hasPaymentDateElement() {
return this.paymentDate != null && !this.paymentDate.isEmpty();
}
public boolean hasPaymentDate() {
return this.paymentDate != null && !this.paymentDate.isEmpty();
}
/**
* @param value {@link #paymentDate} (When payment is due.). This is the
* underlying object with id, value and extensions. The accessor
* "getPaymentDate" gives direct access to the value
*/
public ValuedItemComponent setPaymentDateElement(DateTimeType value) {
this.paymentDate = value;
return this;
}
/**
* @return When payment is due.
*/
public Date getPaymentDate() {
return this.paymentDate == null ? null : this.paymentDate.getValue();
}
/**
* @param value When payment is due.
*/
public ValuedItemComponent setPaymentDate(Date value) {
if (value == null)
this.paymentDate = null;
else {
if (this.paymentDate == null)
this.paymentDate = new DateTimeType();
this.paymentDate.setValue(value);
}
return this;
}
/**
* @return {@link #responsible} (Who will make payment.)
*/
public Reference getResponsible() {
if (this.responsible == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.responsible");
else if (Configuration.doAutoCreate())
this.responsible = new Reference(); // cc
return this.responsible;
}
public boolean hasResponsible() {
return this.responsible != null && !this.responsible.isEmpty();
}
/**
* @param value {@link #responsible} (Who will make payment.)
*/
public ValuedItemComponent setResponsible(Reference value) {
this.responsible = value;
return this;
}
/**
* @return {@link #responsible} The actual object that is the target of the
* reference. The reference library doesn't populate this, but you can
* use it to hold the resource if you resolve it. (Who will make
* payment.)
*/
public Resource getResponsibleTarget() {
return this.responsibleTarget;
}
/**
* @param value {@link #responsible} The actual object that is the target of the
* reference. The reference library doesn't use these, but you can
* use it to hold the resource if you resolve it. (Who will make
* payment.)
*/
public ValuedItemComponent setResponsibleTarget(Resource value) {
this.responsibleTarget = value;
return this;
}
/**
* @return {@link #recipient} (Who will receive payment.)
*/
public Reference getRecipient() {
if (this.recipient == null)
if (Configuration.errorOnAutoCreate())
throw new Error("Attempt to auto-create ValuedItemComponent.recipient");
else if (Configuration.doAutoCreate())
this.recipient = new Reference(); // cc
return this.recipient;
}
public boolean hasRecipient() {
return this.recipient != null && !this.recipient.isEmpty();
}
/**
* @param value {@link #recipient} (Who will receive payment.)
*/
public ValuedItemComponent setRecipient(Reference value) {
this.recipient = value;
return this;
}
/**
* @return {@link #recipient} The actual object that is the target of the
* reference. The reference library doesn't populate this, but you can
* use it to hold the resource if you resolve it. (Who will receive
* payment.)
*/
public Resource getRecipientTarget() {
return this.recipientTarget;
}
/**
* @param value {@link #recipient} The actual object that is the target of the
* reference. The reference library doesn't use these, but you can
* use it to hold the resource if you resolve it. (Who will receive
* payment.)
*/
public ValuedItemComponent setRecipientTarget(Resource value) {
this.recipientTarget = value;
return this;
}
/**
* @return {@link #linkId} (Id of the clause or question text related to the
* context of this valuedItem in the referenced form or
* QuestionnaireResponse.)
*/
public List getLinkId() {
if (this.linkId == null)
this.linkId = new ArrayList();
return this.linkId;
}
/**
* @return Returns a reference to this
for easy method chaining
*/
public ValuedItemComponent setLinkId(List theLinkId) {
this.linkId = theLinkId;
return this;
}
public boolean hasLinkId() {
if (this.linkId == null)
return false;
for (StringType item : this.linkId)
if (!item.isEmpty())
return true;
return false;
}
/**
* @return {@link #linkId} (Id of the clause or question text related to the
* context of this valuedItem in the referenced form or
* QuestionnaireResponse.)
*/
public StringType addLinkIdElement() {// 2
StringType t = new StringType();
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return t;
}
/**
* @param value {@link #linkId} (Id of the clause or question text related to
* the context of this valuedItem in the referenced form or
* QuestionnaireResponse.)
*/
public ValuedItemComponent addLinkId(String value) { // 1
StringType t = new StringType();
t.setValue(value);
if (this.linkId == null)
this.linkId = new ArrayList();
this.linkId.add(t);
return this;
}
/**
* @param value {@link #linkId} (Id of the clause or question text related to
* the context of this valuedItem in the referenced form or
* QuestionnaireResponse.)
*/
public boolean hasLinkId(String value) {
if (this.linkId == null)
return false;
for (StringType v : this.linkId)
if (v.getValue().equals(value)) // string
return true;
return false;
}
/**
* @return {@link #securityLabelNumber} (A set of security labels that define
* which terms are controlled by this condition.)
*/
public List