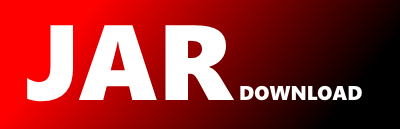
org.hl7.fhir.r4.utils.client.ResourceAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.hl7.fhir.r4 Show documentation
Show all versions of org.hl7.fhir.r4 Show documentation
Builds the hapi fhir r4. Requires hapi-fhir-base and hapi-fhir-utilities be built first and be
excluded from any other poms requiring it.
The newest version!
package org.hl7.fhir.r4.utils.client;
import java.net.URI;
import java.net.URISyntaxException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/*
Copyright (c) 2011+, HL7, Inc.
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
* Neither the name of HL7 nor the names of its contributors may be used to
endorse or promote products derived from this software without specific
prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
POSSIBILITY OF SUCH DAMAGE.
*/
import org.apache.commons.lang3.StringUtils;
import org.apache.http.client.utils.URIBuilder;
import org.hl7.fhir.r4.model.Resource;
import org.hl7.fhir.r4.model.ResourceType;
import org.hl7.fhir.utilities.Utilities;
//Make resources address subclass of URI
/**
* Helper class to manage FHIR Resource URIs
*
* @author Claude Nanjo
*
*/
public class ResourceAddress {
public static final String REGEX_ID_WITH_HISTORY = "(.*)(/)([a-zA-Z0-9]*)(/)([a-z0-9\\-\\.]{1,64})(/_history/)([a-z0-9\\-\\.]{1,64})$";
private URI baseServiceUri;
public ResourceAddress(String endpointPath) throws URISyntaxException {// TODO Revisit this exception
this.baseServiceUri = ResourceAddress.buildAbsoluteURI(endpointPath);
}
public ResourceAddress(URI baseServiceUri) {
this.baseServiceUri = baseServiceUri;
}
public URI getBaseServiceUri() {
return this.baseServiceUri;
}
public URI resolveOperationURLFromClass(Class resourceClass, String name, String parameters) {
return baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "$" + name + "?" + parameters);
}
public URI resolveSearchUri(Class resourceClass, Map parameters) {
return appendHttpParameters(baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "_search"), parameters);
}
private String nameForClassWithSlash(Class resourceClass) {
String n = nameForClass(resourceClass);
return n == null ? "" : n + "/";
}
public URI resolveOperationUri(Class resourceClass, String opName) {
return baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "$" + opName);
}
public URI resolveGetResource(Class resourceClass, String id) {
return baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "/" + id);
}
public URI resolveOperationUri(Class resourceClass, String opName,
Map parameters) {
return appendHttpParameters(baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "$" + opName),
parameters);
}
public URI resolveValidateUri(Class resourceClass, String id) {
return baseServiceUri.resolve(nameForClassWithSlash(resourceClass) + "$validate/" + id);
}
public URI resolveGetUriFromResourceClass(Class resourceClass) {
return baseServiceUri.resolve(nameForClass(resourceClass));
}
public URI resolveGetUriFromResourceClassAndId(Class resourceClass, String id) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id);
}
public URI resolveGetUriFromResourceClassAndId(String resourceClass, String id) {
return baseServiceUri.resolve(resourceClass + "/" + id);
}
public URI resolveGetUriFromResourceClassAndIdAndVersion(Class resourceClass, String id,
String version) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id + "/_history/" + version);
}
public URI resolveGetUriFromResourceClassAndCanonical(Class resourceClass,
String canonicalUrl) {
if (canonicalUrl.contains("|"))
return baseServiceUri
.resolve(nameForClass(resourceClass) + "?url=" + canonicalUrl.substring(0, canonicalUrl.indexOf("|"))
+ "&version=" + canonicalUrl.substring(canonicalUrl.indexOf("|") + 1));
else
return baseServiceUri.resolve(nameForClass(resourceClass) + "?url=" + canonicalUrl);
}
public URI resolveGetHistoryForAllResources(int count) {
if (count > 0) {
return appendHttpParameter(baseServiceUri.resolve("_history"), "_count", "" + count);
} else {
return baseServiceUri.resolve("_history");
}
}
public URI resolveGetHistoryForResourceId(Class resourceClass, String id, int count) {
return resolveGetHistoryUriForResourceId(resourceClass, id, null, count);
}
protected URI resolveGetHistoryUriForResourceId(Class resourceClass, String id, Object since,
int count) {
Map parameters = getHistoryParameters(since, count);
return appendHttpParameters(baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id + "/_history"),
parameters);
}
public URI resolveGetHistoryForResourceType(Class resourceClass, int count) {
Map parameters = getHistoryParameters(null, count);
return appendHttpParameters(baseServiceUri.resolve(nameForClass(resourceClass) + "/_history"), parameters);
}
public URI resolveGetHistoryForResourceType(Class resourceClass, Object since, int count) {
Map parameters = getHistoryParameters(since, count);
return appendHttpParameters(baseServiceUri.resolve(nameForClass(resourceClass) + "/_history"), parameters);
}
public URI resolveGetHistoryForAllResources(Calendar since, int count) {
Map parameters = getHistoryParameters(since, count);
return appendHttpParameters(baseServiceUri.resolve("_history"), parameters);
}
public URI resolveGetHistoryForAllResources(Date since, int count) {
Map parameters = getHistoryParameters(since, count);
return appendHttpParameters(baseServiceUri.resolve("_history"), parameters);
}
public Map getHistoryParameters(Object since, int count) {
Map parameters = new HashMap();
if (since != null) {
parameters.put("_since", since.toString());
}
if (count > 0) {
parameters.put("_count", "" + count);
}
return parameters;
}
public URI resolveGetHistoryForResourceId(Class resourceClass, String id, Calendar since,
int count) {
return resolveGetHistoryUriForResourceId(resourceClass, id, since, count);
}
public URI resolveGetHistoryForResourceId(Class resourceClass, String id, Date since,
int count) {
return resolveGetHistoryUriForResourceId(resourceClass, id, since, count);
}
public URI resolveGetHistoryForResourceType(Class resourceClass, Calendar since, int count) {
return resolveGetHistoryForResourceType(resourceClass, getCalendarDateInIsoTimeFormat(since), count);
}
public URI resolveGetHistoryForResourceType(Class resourceClass, Date since, int count) {
return resolveGetHistoryForResourceType(resourceClass, since.toString(), count);
}
public URI resolveGetAllTags() {
return baseServiceUri.resolve("_tags");
}
public URI resolveGetAllTagsForResourceType(Class resourceClass) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/_tags");
}
public URI resolveGetTagsForReference(Class resourceClass, String id) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id + "/_tags");
}
public URI resolveGetTagsForResourceVersion(Class resourceClass, String id, String version) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id + "/_history/" + version + "/_tags");
}
public URI resolveDeleteTagsForResourceVersion(Class resourceClass, String id,
String version) {
return baseServiceUri.resolve(nameForClass(resourceClass) + "/" + id + "/_history/" + version + "/_tags/_delete");
}
public String nameForClass(Class resourceClass) {
if (resourceClass == null)
return null;
String res = resourceClass.getSimpleName();
if (res.equals("List_"))
return "List";
else
return res;
}
public URI resolveMetadataUri(boolean quick) {
return baseServiceUri.resolve(quick ? "metadata?_summary=true" : "metadata");
}
public URI resolveMetadataTxCaps() {
return baseServiceUri.resolve("metadata?mode=terminology");
}
/**
* For now, assume this type of location header structure. Generalize later:
* http://hl7connect.healthintersections.com.au/svc/fhir/318/_history/1
*/
public static ResourceVersionedIdentifier parseCreateLocation(String locationResponseHeader) {
Pattern pattern = Pattern.compile(REGEX_ID_WITH_HISTORY);
Matcher matcher = pattern.matcher(locationResponseHeader);
ResourceVersionedIdentifier parsedHeader = null;
if (matcher.matches()) {
String serviceRoot = matcher.group(1);
String resourceType = matcher.group(3);
String id = matcher.group(5);
String version = matcher.group(7);
parsedHeader = new ResourceVersionedIdentifier(serviceRoot, resourceType, id, version);
}
return parsedHeader;
}
public static URI buildAbsoluteURI(String absoluteURI) {
if (StringUtils.isBlank(absoluteURI)) {
throw new EFhirClientException("Invalid URI", new URISyntaxException(absoluteURI, "URI/URL cannot be blank"));
}
String endpoint = appendForwardSlashToPath(absoluteURI);
return buildEndpointUriFromString(endpoint);
}
public static String appendForwardSlashToPath(String path) {
if (path.lastIndexOf('/') != path.length() - 1) {
path += "/";
}
return path;
}
public static URI buildEndpointUriFromString(String endpointPath) {
URI uri = null;
try {
URIBuilder uriBuilder = new URIBuilder(endpointPath);
uri = uriBuilder.build();
String scheme = uri.getScheme();
String host = uri.getHost();
if (!scheme.equalsIgnoreCase("http") && !scheme.equalsIgnoreCase("https")) {
throw new EFhirClientException("Scheme must be 'http' or 'https': " + uri);
}
if (StringUtils.isBlank(host)) {
throw new EFhirClientException("host cannot be blank: " + uri);
}
} catch (URISyntaxException e) {
throw new EFhirClientException("Invalid URI", e);
}
return uri;
}
public static URI appendQueryStringToUri(URI uri, String parameterName, String parameterValue) {
URI modifiedUri = null;
try {
URIBuilder uriBuilder = new URIBuilder(uri);
uriBuilder.setQuery(parameterName + "=" + parameterValue);
modifiedUri = uriBuilder.build();
} catch (Exception e) {
throw new EFhirClientException(
"Unable to append query parameter '" + parameterName + "=" + parameterValue + " to URI " + uri, e);
}
return modifiedUri;
}
public static String buildRelativePathFromResourceType(ResourceType resourceType) {
// return resourceType.toString().toLowerCase()+"/";
return resourceType.toString() + "/";
}
public static String buildRelativePathFromResourceType(ResourceType resourceType, String id) {
return buildRelativePathFromResourceType(resourceType) + "@" + id;
}
public static String buildRelativePathFromReference(Resource resource) {
return buildRelativePathFromResourceType(resource.getResourceType());
}
public static String buildRelativePathFromReference(Resource resource, String id) {
return buildRelativePathFromResourceType(resource.getResourceType(), id);
}
public static class ResourceVersionedIdentifier {
private String serviceRoot;
private String resourceType;
private String id;
private String version;
private URI resourceLocation;
public ResourceVersionedIdentifier(String serviceRoot, String resourceType, String id, String version,
URI resourceLocation) {
this.serviceRoot = serviceRoot;
this.resourceType = resourceType;
this.id = id;
this.version = version;
this.resourceLocation = resourceLocation;
}
public ResourceVersionedIdentifier(String resourceType, String id, String version, URI resourceLocation) {
this(null, resourceType, id, version, resourceLocation);
}
public ResourceVersionedIdentifier(String serviceRoot, String resourceType, String id, String version) {
this(serviceRoot, resourceType, id, version, null);
}
public ResourceVersionedIdentifier(String resourceType, String id, String version) {
this(null, resourceType, id, version, null);
}
public ResourceVersionedIdentifier(String resourceType, String id) {
this.id = id;
}
public String getId() {
return this.id;
}
protected void setId(String id) {
this.id = id;
}
public String getVersionId() {
return this.version;
}
protected void setVersionId(String version) {
this.version = version;
}
public String getResourceType() {
return resourceType;
}
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
public String getServiceRoot() {
return serviceRoot;
}
public void setServiceRoot(String serviceRoot) {
this.serviceRoot = serviceRoot;
}
public String getResourcePath() {
return this.serviceRoot + "/" + this.resourceType + "/" + this.id;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public URI getResourceLocation() {
return this.resourceLocation;
}
public void setResourceLocation(URI resourceLocation) {
this.resourceLocation = resourceLocation;
}
}
public static String getCalendarDateInIsoTimeFormat(Calendar calendar) {
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd'T'hh:mm:ss", new Locale("en", "US"));// TODO Move out
format.setTimeZone(TimeZone.getTimeZone("GMT"));
return format.format(calendar.getTime());
}
public static URI appendHttpParameter(URI basePath, String httpParameterName, String httpParameterValue) {
Map parameters = new HashMap();
parameters.put(httpParameterName, httpParameterValue);
return appendHttpParameters(basePath, parameters);
}
public static URI appendHttpParameters(URI basePath, Map parameters) {
try {
Set httpParameterNames = parameters.keySet();
String query = basePath.getQuery();
for (String httpParameterName : httpParameterNames) {
if (query != null) {
query += "&";
} else {
query = "";
}
query += httpParameterName + "=" + Utilities.encodeUri(parameters.get(httpParameterName));
}
return new URI(basePath.getScheme(), basePath.getUserInfo(), basePath.getHost(), basePath.getPort(),
basePath.getPath(), query, basePath.getFragment());
} catch (Exception e) {
throw new EFhirClientException("Error appending http parameter", e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy