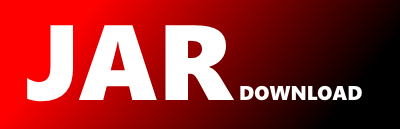
commonMain.dev.inmo.micro_utils.repos.cache.KVCache.kt Maven / Gradle / Ivy
package dev.inmo.micro_utils.repos.cache
import dev.inmo.micro_utils.repos.*
import dev.inmo.micro_utils.pagination.utils.getAllWithNextPaging
import kotlinx.coroutines.sync.Mutex
import kotlinx.coroutines.sync.withLock
interface KVCache : KeyValueRepo
open class SimpleKVCache(
protected val cachedValuesCount: Int,
private val kvParent: KeyValueRepo = MapKeyValueRepo()
) : KVCache, KeyValueRepo by kvParent {
protected open val cacheStack = ArrayList(cachedValuesCount)
protected val syncMutex = Mutex()
protected suspend fun makeUnset(toUnset: List) {
cacheStack.removeAll(toUnset)
kvParent.unset(toUnset)
}
override suspend fun set(toSet: Map) {
syncMutex.withLock {
if (toSet.size > cachedValuesCount) {
cacheStack.clear()
kvParent.unset(getAllWithNextPaging { kvParent.keys(it) })
val keysToInclude = toSet.keys.drop(toSet.size - cachedValuesCount)
cacheStack.addAll(keysToInclude)
kvParent.set(keysToInclude.associateWith { toSet.getValue(it) })
} else {
makeUnset(cacheStack.take(toSet.size))
}
}
}
override suspend fun unset(toUnset: List) {
syncMutex.withLock { makeUnset(toUnset) }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy