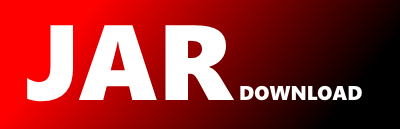
commonMain.dev.kord.cache.map.MapDataCache.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cache-map-jvm Show documentation
Show all versions of cache-map-jvm Show documentation
Adaptable cache with query-like operations
The newest version!
package dev.kord.cache.map
import dev.kord.cache.api.DataCache
import dev.kord.cache.api.DataEntryCache
import dev.kord.cache.api.data.DataDescription
import dev.kord.cache.api.delegate.DelegatingDataCache
import dev.kord.cache.api.delegate.EntrySupplier
import dev.kord.cache.map.internal.MapEntryCache
import dev.kord.cache.api.ConcurrentHashMap
import kotlin.reflect.KType
import kotlin.reflect.typeOf
typealias Supplier = MapLikeCollection.Companion.(description: DataDescription) -> MapLikeCollection
object MapDataCache {
private class MapSupplier(private val default: Supplier, private val suppliers: MutableMap>) : EntrySupplier {
@Suppress("UNCHECKED_CAST")
override suspend fun supply(cache: DataCache, description: DataDescription): DataEntryCache {
val supplier = (suppliers[description.type] ?: default) as Supplier
val map = supplier(MapLikeCollection, description)
return MapEntryCache(cache, description as DataDescription, map as MapLikeCollection)
}
}
class Builder {
val suppliers: MutableMap> = mutableMapOf()
private var default: Supplier = { concurrentHashMap() }
/**
* Assigns the [supplier] to the specific type.
*/
@Suppress("UNCHECKED_CAST")
inline fun forType(noinline supplier: Supplier?) {
if (supplier == null) suppliers.remove(typeOf())
suppliers[typeOf()] = supplier as Supplier<*>
}
/**
* Sets the [supplier] for types that weren't defined by [forType].
*/
fun default(supplier: Supplier) {
default = supplier
}
fun build(): DataCache = DelegatingDataCache(MapSupplier(default, suppliers))
}
/**
* Creates a new [DataCache] configured by [builder].
* [Builder.default] will use a [ConcurrentHashMap] to store entries.
*/
inline operator fun invoke(builder: Builder.() -> Unit = {}) = Builder().apply(builder).build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy