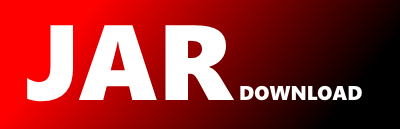
commonMain.supplier.EntitySupplyStrategy.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kord-core-jvm Show documentation
Show all versions of kord-core-jvm Show documentation
Idiomatic Kotlin Wrapper for The Discord API
package dev.kord.core.supplier
import dev.kord.core.Kord
import dev.kord.core.supplier.EntitySupplyStrategy.Companion.cache
/**
* A supplier that accepts a [Kord] instance and returns an [EntitySupplier] of type [T].
*/
public interface EntitySupplyStrategy {
/**
* Returns an [EntitySupplier] of type [T] that operates on the [kord] instance.
*/
public fun supply(kord: Kord): T
public companion object {
/**
* A supplier providing a strategy which exclusively uses REST calls to fetch entities.
* See [RestEntitySupplier] for more details.
*/
public val rest: EntitySupplyStrategy = object : EntitySupplyStrategy {
override fun supply(kord: Kord): RestEntitySupplier = RestEntitySupplier(kord)
override fun toString(): String = "EntitySupplyStrategy.rest"
}
/**
* A supplier providing a strategy which exclusively uses cache to fetch entities.
* See [CacheEntitySupplier] for more details.
*/
public val cache: EntitySupplyStrategy =
object : EntitySupplyStrategy {
override fun supply(kord: Kord): CacheEntitySupplier = CacheEntitySupplier(kord)
override fun toString(): String = "EntitySupplyStrategy.cache"
}
/**
* A supplier providing a strategy which exclusively uses REST calls to fetch entities.
* fetched entities are stored in [Kord's cache][Kord.cache].
* See [StoreEntitySupplier] for more details.
*/
public val cachingRest: EntitySupplyStrategy = object : EntitySupplyStrategy {
override fun supply(kord: Kord): EntitySupplier {
return StoreEntitySupplier(rest.supply(kord), kord.cache)
}
override fun toString(): String = "EntitySupplyStrategy.cachingRest"
}
/**
* A supplier providing a strategy which will first operate on the [cache] supplier. When an entity
* is not present from cache it will be fetched from [rest] instead. Operations that return flows
* will only fall back to rest when the returned flow contained no elements.
*/
public val cacheWithRestFallback: EntitySupplyStrategy =
object : EntitySupplyStrategy {
override fun supply(kord: Kord): EntitySupplier = cache.supply(kord).withFallback(rest.supply(kord))
override fun toString(): String = "EntitySupplyStrategy.cacheWithRestFallback"
}
/**
* A supplier providing a strategy which will first operate on the [cache] supplier. When an entity
* is not present from cache it will be fetched from [cachingRest] instead which will update [cache] with fetched elements.
* Operations that return flows will only fall back to rest when the returned flow contained no elements.
*/
public val cacheWithCachingRestFallback: EntitySupplyStrategy =
object : EntitySupplyStrategy {
override fun supply(kord: Kord): EntitySupplier =
cache.supply(kord).withFallback(cachingRest.supply(kord))
override fun toString(): String = "EntitySupplyStrategy.cacheWithCachingRestFallback"
}
/**
* Create an [EntitySupplyStrategy] from the given [supplier].
*/
public operator fun invoke(
supplier: (Kord) -> T
): EntitySupplyStrategy = object : EntitySupplyStrategy {
override fun supply(kord: Kord): T = supplier(kord)
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy