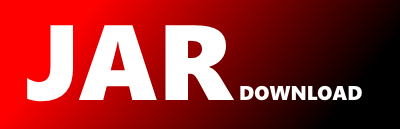
commonMain.cache.KordCache.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kord-core-jvm Show documentation
Show all versions of kord-core-jvm Show documentation
Idiomatic Kotlin Wrapper for The Discord API
The newest version!
package dev.kord.core.cache
import dev.kord.cache.api.DataCache
import dev.kord.cache.api.DataEntryCache
import dev.kord.cache.api.data.DataDescription
import dev.kord.cache.api.delegate.DelegatingDataCache
import dev.kord.cache.api.delegate.EntrySupplier
import dev.kord.cache.map.MapLikeCollection
import dev.kord.cache.map.internal.MapEntryCache
import dev.kord.common.concurrentHashMap
import dev.kord.common.entity.Snowflake
import dev.kord.core.cache.data.*
public typealias Generator = (cache: DataCache, description: DataDescription) -> DataEntryCache
public class KordCacheBuilder {
/**
* The default behavior for all types not explicitly configured, by default a [concurrentHashMap] is supplied.
*/
public var defaultGenerator: Generator = { cache, description ->
MapEntryCache(cache, description, MapLikeCollection.concurrentHashMap())
}
private val descriptionGenerators: MutableMap, Generator<*, *>> = mutableMapOf()
init {
messages { _, _ -> DataEntryCache.none() }
}
/**
* Disables caching for all entries, clearing all custom generators and setting the default to [none].
*/
public fun disableAll() {
descriptionGenerators.clear()
defaultGenerator = none()
}
/**
* A Generator creating [DataEntryCaches][DataEntryCache] that won't store any entities, can be used to disable caching.
*/
public fun none(): Generator = { _, _ -> DataEntryCache.none() }
@Suppress("UNCHECKED_CAST")
public fun forDescription(description: DataDescription, generator: Generator?) {
if (generator == null) return run {
descriptionGenerators.remove(description)
}
descriptionGenerators[description] = generator as Generator<*, *>
}
/**
* Configures the caching for [MessageData].
* Your application will generally handle a lot of messages during its lifetime, as such it's advised to
* limit the amount of messages cached in some way.
*
* ```kotlin
* cache {
* messages(none()) //disable caching entirely
* messages(lruCache(100)) //only keep the latest 100 messages
* }
* ```
*/
public fun messages(generator: Generator): Unit = forDescription(MessageData.description, generator)
/**
* Configures the caching for [RoleData].
*/
public fun roles(generator: Generator): Unit = forDescription(RoleData.description, generator)
/**
* Configures the caching for [ChannelData].
*/
public fun channels(generator: Generator): Unit = forDescription(ChannelData.description, generator)
/**
* Configures the caching for [GuildData].
*/
public fun guilds(generator: Generator): Unit = forDescription(GuildData.description, generator)
/**
* Configures the caching for [MemberData].
* It's advised to configure user and member data similarly, so that every member in cache also has its user data cached.
* Failing to do so would result in a performance hit when fetching members.
*/
public fun members(generator: Generator): Unit = forDescription(MemberData.description, generator)
/**
* Configures the caching for [UserData].
* It's advised to configure user and member data similarly, so that every member in cache also has its user data cached.
* Failing to do so would result in a performance hit when fetching members.
*/
public fun users(generator: Generator): Unit = forDescription(UserData.description, generator)
public fun stickers(generator: Generator): Unit = forDescription(StickerData.description, generator)
/**
* Configures the caching for [EmojiData].
*/
public fun emojis(generator: Generator): Unit = forDescription(EmojiData.description, generator)
/**
* Configures the caching for [WebhookData].
*/
public fun webhooks(generator: Generator): Unit = forDescription(WebhookData.description, generator)
/**
* Configures the caching for [PresenceData].
*/
public fun presences(generator: Generator): Unit = forDescription(PresenceData.description, generator)
/**
* Configures the caching for [VoiceStateData].
*/
public fun voiceState(generator: Generator): Unit = forDescription(VoiceStateData.description, generator)
/** Configures the caching for [AutoModerationRuleData]. */
public fun autoModerationRules(generator: Generator): Unit =
forDescription(AutoModerationRuleData.description, generator)
/** Configures the caching for [EntitlementData]. */
public fun entitlements(generator: Generator): Unit =
forDescription(EntitlementData.description, generator)
/** Configures the caching for [SubscriptionData]. */
public fun subscriptions(generator: Generator): Unit =
forDescription(SubscriptionData.description, generator)
public fun build(): DataCache = DelegatingDataCache(EntrySupplier.invoke { cache, description ->
val generator = descriptionGenerators[description] ?: defaultGenerator
generator(cache, description)
})
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy