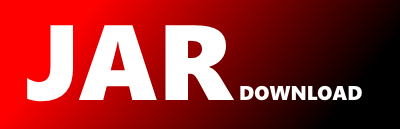
commonMain.entity.GuildOnboarding.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kord-core-jvm Show documentation
Show all versions of kord-core-jvm Show documentation
Idiomatic Kotlin Wrapper for The Discord API
The newest version!
package dev.kord.core.entity
import dev.kord.common.entity.*
import dev.kord.common.exception.RequestException
import dev.kord.core.Kord
import dev.kord.core.KordObject
import dev.kord.core.behavior.GuildBehavior
import dev.kord.core.behavior.RoleBehavior
import dev.kord.core.behavior.channel.TopGuildChannelBehavior
import dev.kord.core.cache.data.toData
import dev.kord.core.entity.channel.TopGuildChannel
import dev.kord.core.exception.EntityNotFoundException
import dev.kord.core.hash
import dev.kord.core.supplier.EntitySupplier
import dev.kord.core.supplier.EntitySupplyStrategy
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.emptyFlow
import kotlinx.coroutines.flow.filter
/**
* Represents the [onboarding](https://support.discord.com/hc/en-us/articles/11074987197975-Community-Onboarding-FAQ)
* flow for a [Guild].
*/
public class GuildOnboarding(
public val data: DiscordGuildOnboarding,
override val kord: Kord,
override val supplier: EntitySupplier = kord.defaultSupplier,
) : KordObject, Strategizable {
/** The ID of the [Guild] this onboarding is part of. */
public val guildId: Snowflake get() = data.guildId
/** The behavior of the [Guild] this onboarding is part of. */
public val guild: GuildBehavior get() = GuildBehavior(guildId, kord)
/** The [Prompt]s shown during onboarding and in customize community. */
public val prompts: List get() = data.prompts.map { Prompt(it, guildId, kord) }
/** The IDs of the [channels][TopGuildChannel] that [Member]s get opted into automatically. */
public val defaultChannelIds: List get() = data.defaultChannelIds
/** The behaviors of the [channels][TopGuildChannel] that [Member]s get opted into automatically. */
public val defaultChannelBehaviors: List
get() = defaultChannelIds.map { channelId -> TopGuildChannelBehavior(guildId, id = channelId, kord) }
/** Whether onboarding is enabled in the [guild]. */
public val isEnabled: Boolean get() = data.enabled
/** Current [mode][OnboardingMode] of onboarding. */
public val mode: OnboardingMode get() = data.mode
/**
* Requests the [Guild] this onboarding is part of.
*
* @throws RequestException if something went wrong while retrieving the guild.
* @throws EntityNotFoundException if the guild is null.
*/
public suspend fun getGuild(): Guild = supplier.getGuild(guildId)
/**
* Requests the [Guild] this onboarding is part of, returns `null` when the guild isn't present.
*
* @throws RequestException if something went wrong while retrieving the guild.
*/
public suspend fun getGuildOrNull(): Guild? = supplier.getGuildOrNull(guildId)
/**
* Requests the [channels][TopGuildChannel] that [Member]s get opted into automatically.
*
* The returned [Flow] is lazily executed, any [RequestException] will be thrown on
* [terminal operators](https://kotlinlang.org/docs/reference/coroutines/flow.html#terminal-flow-operators) instead.
*/
public val defaultChannels: Flow
get() {
val ids = defaultChannelIds
return if (ids.isEmpty()) emptyFlow() else supplier.getGuildChannels(guildId).filter { it.id in ids }
}
override fun withStrategy(strategy: EntitySupplyStrategy<*>): GuildOnboarding =
GuildOnboarding(data, kord, strategy.supply(kord))
override fun toString(): String = "GuildOnboarding(data=$data, kord=$kord, supplier=$supplier)"
/**
* Represents a prompt for a [GuildOnboarding].
*
* @property guildId The ID of the [Guild] the [onboarding][GuildOnboarding] is part of.
*/
public class Prompt(
public val data: DiscordOnboardingPrompt,
public val guildId: Snowflake,
override val kord: Kord,
) : KordEntity {
override val id: Snowflake get() = data.id
/** The [type][OnboardingPromptType] of this prompt. */
public val type: OnboardingPromptType get() = data.type
/** The [Option]s available within this prompt. */
public val options: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy