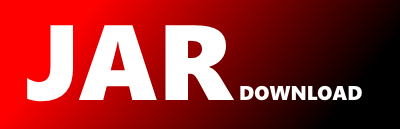
commonMain.entity.Role.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kord-core-jvm Show documentation
Show all versions of kord-core-jvm Show documentation
Idiomatic Kotlin Wrapper for The Discord API
The newest version!
package dev.kord.core.entity
import dev.kord.common.Color
import dev.kord.common.entity.Permissions
import dev.kord.common.entity.RoleFlags
import dev.kord.common.entity.Snowflake
import dev.kord.common.entity.optional.unwrap
import dev.kord.core.Kord
import dev.kord.core.behavior.RoleBehavior
import dev.kord.core.cache.data.RoleData
import dev.kord.core.supplier.EntitySupplier
import dev.kord.core.supplier.EntitySupplyStrategy
import dev.kord.core.hash
public data class Role(
val data: RoleData,
override val kord: Kord,
override val supplier: EntitySupplier = kord.defaultSupplier
) : RoleBehavior {
override val id: Snowflake
get() = data.id
override val guildId: Snowflake
get() = data.guildId
public val color: Color get() = Color(data.color)
public val hoisted: Boolean get() = data.hoisted
val iconHash: String? get() = data.icon.value
val icon: Asset? get() = iconHash?.let { Asset.roleIcon(id, it, kord) }
val unicodeEmoji: String? = data.unicodeEmoji.value
public val managed: Boolean get() = data.managed
public val mentionable: Boolean get() = data.mentionable
public val name: String get() = data.name
public val permissions: Permissions get() = data.permissions
public val rawPosition: Int get() = data.position
override suspend fun asRole(): Role = this
override suspend fun asRoleOrNull(): Role = this
/**
* The tags of this role, if present.
*/
public val tags: RoleTags? get() = data.tags.unwrap { RoleTags(it, guildId, kord) }
/** The [RoleFlags] of this role. */
public val flags: RoleFlags get() = data.flags
override fun compareTo(other: Entity): Int = when (other) {
is Role -> compareBy { it.rawPosition }.thenBy { it.guildId }.compare(this, other)
else -> super.compareTo(other)
}
/**
* Returns a new [Role] with the given [strategy].
*/
override fun withStrategy(strategy: EntitySupplyStrategy<*>): Role = Role(data, kord, strategy.supply(kord))
override fun hashCode(): Int = hash(id, guildId)
override fun equals(other: Any?): Boolean = when (other) {
is RoleBehavior -> other.id == id && other.guildId == guildId
else -> false
}
override fun toString(): String {
return "Role(data=$data, kord=$kord, supplier=$supplier)"
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy