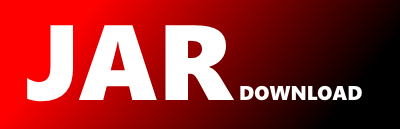
commonMain.entity.component.ButtonComponent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kord-core Show documentation
Show all versions of kord-core Show documentation
Idiomatic Kotlin Wrapper for The Discord API
The newest version!
package dev.kord.core.entity.component
import dev.kord.common.entity.ButtonStyle
import dev.kord.common.entity.ComponentType
import dev.kord.common.entity.Snowflake
import dev.kord.common.entity.optional.value
import dev.kord.core.cache.data.ChatComponentData
import dev.kord.core.entity.ReactionEmoji
import dev.kord.core.entity.interaction.ButtonInteraction
import dev.kord.core.entity.interaction.ComponentInteraction
import dev.kord.core.entity.monetization.Sku
import dev.kord.core.event.interaction.InteractionCreateEvent
/**
* An interactive component rendered on a Message.
* If this button contains a [customId] and is clicked by a user,
* a [InteractionCreateEvent] with a [ComponentInteraction] will fire.
*/
public class ButtonComponent(override val data: ChatComponentData) : Component {
override val type: ComponentType.Button
get() = ComponentType.Button
/**
* The style of this button, [ButtonStyle.Link] buttons will always have a [url], [ButtonStyle.Premium] buttons will
* always have an [skuId].
*/
public val style: ButtonStyle get() = data.style.value!!
/**
* The text that appears on the button, if present.
*/
public val label: String? get() = data.label.value
/**
* The emoji that appears on the button, if present.
*/
public val emoji: ReactionEmoji?
get() = with(data.emoji.value) {
if (this == null) return@with null
when (id) {
null -> ReactionEmoji.Unicode(name!!)
else -> ReactionEmoji.Custom(
id!!, name ?: "", animated.value!!
)
}
}
/**
* The custom identifier for any [ComponentInteractions][ButtonInteraction] this button will trigger. Present if
* this button is not a link or premium button.
*/
public val customId: String? get() = data.customId.value
/**
* The ID of the [Sku] this button will prompt to purchase. Present if this button is a premium button.
*/
public val skuId: Snowflake? get() = data.skuId.value
/**
* The url the button will link to. Present if this button is a link button.
*/
public val url: String? get() = data.url.value
/**
* Whether this button can be clicked.
*/
public val disabled: Boolean get() = data.disabled.discordBoolean
override fun toString(): String = "ButtonComponent(data=$data)"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy