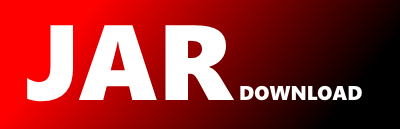
dev.langchain4j.service.spring.AiServiceFactory Maven / Gradle / Ivy
package dev.langchain4j.service.spring;
import dev.langchain4j.memory.ChatMemory;
import dev.langchain4j.memory.chat.ChatMemoryProvider;
import dev.langchain4j.model.chat.ChatLanguageModel;
import dev.langchain4j.model.chat.StreamingChatLanguageModel;
import dev.langchain4j.model.moderation.ModerationModel;
import dev.langchain4j.rag.RetrievalAugmentor;
import dev.langchain4j.rag.content.retriever.ContentRetriever;
import dev.langchain4j.service.AiServices;
import org.springframework.beans.factory.FactoryBean;
import java.util.List;
import static dev.langchain4j.internal.Utils.isNullOrEmpty;
class AiServiceFactory implements FactoryBean
© 2015 - 2025 Weber Informatics LLC | Privacy Policy