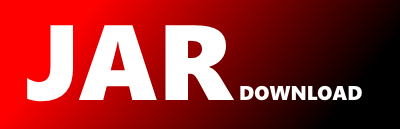
dev.langchain4j.service.Result Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of langchain4j Show documentation
Show all versions of langchain4j Show documentation
Java implementation of LangChain: Integrate your Java application with countless AI
tools and services smoothly
package dev.langchain4j.service;
import dev.langchain4j.model.output.FinishReason;
import dev.langchain4j.model.output.TokenUsage;
import dev.langchain4j.rag.content.Content;
import dev.langchain4j.service.tool.ToolExecution;
import java.util.List;
import static dev.langchain4j.internal.Utils.copyIfNotNull;
import static dev.langchain4j.internal.ValidationUtils.ensureNotNull;
/**
* Represents the result of an AI Service invocation.
* It contains actual content (LLM response) and additional information associated with it,
* such as {@link TokenUsage} and sources ({@link Content}s retrieved during RAG).
*
* @param The type of the content. Can be of any return type supported by AI Services,
* such as String, Enum, MyCustomPojo, etc.
*/
public class Result {
private final T content;
private final TokenUsage tokenUsage;
private final List sources;
private final FinishReason finishReason;
private final List toolExecutions;
public Result(T content, TokenUsage tokenUsage, List sources, FinishReason finishReason, List toolExecutions) {
this.content = ensureNotNull(content, "content");
this.tokenUsage = tokenUsage;
this.sources = copyIfNotNull(sources);
this.finishReason = finishReason;
this.toolExecutions = copyIfNotNull(toolExecutions);
}
public static ResultBuilder builder() {
return new ResultBuilder();
}
public T content() {
return content;
}
public TokenUsage tokenUsage() {
return tokenUsage;
}
public List sources() {
return sources;
}
public FinishReason finishReason() {
return finishReason;
}
public List toolExecutions() {
return toolExecutions;
}
public static class ResultBuilder {
private T content;
private TokenUsage tokenUsage;
private List sources;
private FinishReason finishReason;
private List toolExecutions;
ResultBuilder() {
}
public ResultBuilder content(T content) {
this.content = content;
return this;
}
public ResultBuilder tokenUsage(TokenUsage tokenUsage) {
this.tokenUsage = tokenUsage;
return this;
}
public ResultBuilder sources(List sources) {
this.sources = sources;
return this;
}
public ResultBuilder finishReason(FinishReason finishReason) {
this.finishReason = finishReason;
return this;
}
public ResultBuilder toolExecutions(List toolExecutions) {
this.toolExecutions = toolExecutions;
return this;
}
public Result build() {
return new Result(this.content, this.tokenUsage, this.sources, this.finishReason, this.toolExecutions);
}
public String toString() {
return "Result.ResultBuilder(content=" + this.content + ", tokenUsage=" + this.tokenUsage + ", sources=" + this.sources + ", finishReason=" + this.finishReason + ", toolExecutions=" + this.toolExecutions + ")";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy