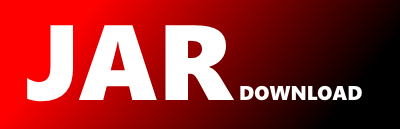
dev.logchange.md.table.MarkdownTableBuilder Maven / Gradle / Ivy
package dev.logchange.md.table;
import lombok.extern.java.Log;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
@Log
public class MarkdownTableBuilder {
private MarkdownTable table;
/**
* Creates instance of {@code MarkdownTableBuilder} without initialization of underneath {@code MarkdownTable}.
* First call of {@link #addRow(Object... objects) addRow} method will try to initialize {@code MarkdownTable} field.
*/
public MarkdownTableBuilder() {
}
/**
* Creates instance of {@code MarkdownTableBuilder} and try to initialize underneath {@code MarkdownTable}.
* Successful initialization will create {@code MarkdownTable} with header {@code MarkdownTableRow}
* which specifies the number of columns in the table.
*
* Every other row will be adjusted, excess cells will be trimmed and the missing ones will be filled
* with empty cells, to the number of cells in header.
*
* In case of {@code MarkdownTableValidationException} initialization is skipped and the next call of
* {@link #addRow(Object... objects) addRow} method will also try to initialize {@code MarkdownTable} field.
* @param objects {@code Object...} arguments to be converted into header {@code MarkdownTableRow}
*/
public MarkdownTableBuilder(Object... objects) {
initializeTable(objects);
}
private void initializeTable(Object... objects) {
try {
this.table = MarkdownTable.of(MarkdownTableRow.of(Arrays.asList(objects)));
} catch (MarkdownTableValidationException exception) {
log.warning("Creation of MarkdownTable caused exception with following message: " + exception.getMessage());
}
}
/**
* Tries to add new {@code MarkdownTableRow} to the {@code MarkdownTable}.
* If the {@code MarkdownTable} filed has not been initialized yet this method will try to create {@code MarkdownTable}
* with header {@code MarkdownTableRow} which specifies the number of columns in the table.
*
* Every other added row will be adjusted, excess cells will be trimmed and the missing ones will be filled
* with empty cells, to the number of cells in header.
*
* In case of {@code MarkdownTableValidationException} initialization of {@code MarkdownTableRow} is skipped
* and the next call of this method will also try to initialize this field.
*
* @param objects {@code Object...} arguments to be converted into {@code MarkdownTableRow} and added to {@code MarkdownTable}
* @return this {@code MarkdownTableBuilder} instance of builder.
*/
public MarkdownTableBuilder addRow(Object... objects) {
if (table == null) {
initializeTable(objects);
} else {
table.addRow(adjustNumberOfColumns(objects));
}
return this;
}
private MarkdownTableRow adjustNumberOfColumns(Object... objects) {
List