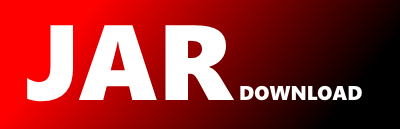
dev.monosoul.jooq.settings.PropertiesReader.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jooq-gradle-plugin Show documentation
Show all versions of jooq-gradle-plugin Show documentation
Generates jOOQ classes using dockerized database
package dev.monosoul.jooq.settings
import dev.monosoul.jooq.settings.JooqDockerPluginSettings.WithContainer
import dev.monosoul.jooq.settings.JooqDockerPluginSettings.WithoutContainer
import org.gradle.api.Action
import kotlin.reflect.KFunction2
import kotlin.reflect.KMutableProperty0
internal object PropertiesReader {
const val PREFIX = "dev.monosoul.jooq."
private val WITH_CONTAINER = "${PREFIX}${functionName(SettingsAware::withContainer)}."
private val WITHOUT_CONTAINER = "${PREFIX}${functionName(SettingsAware::withoutContainer)}."
private val IMAGE_PREFIX = "${functionName(ImageAware::image)}."
private val DATABASE_PREFIX = "${functionName(DbAware::db)}."
private val JDBC_PREFIX = "${functionName(JdbcAware::jdbc)}."
fun WithContainer.applyPropertiesFrom(pluginProperties: Map): JooqDockerPluginSettings =
if (pluginProperties.keys.any { it.startsWith(WITHOUT_CONTAINER) }) {
WithoutContainer {
applyPropertiesFrom(pluginProperties)
}
} else {
onlyApplyPropertiesFrom(pluginProperties)
}
private fun WithContainer.onlyApplyPropertiesFrom(pluginProperties: Map) =
apply {
image.applyPropertiesFrom(pluginProperties)
database.applyPropertiesFrom(pluginProperties)
}
fun WithoutContainer.applyPropertiesFrom(pluginProperties: Map): JooqDockerPluginSettings =
apply {
database.applyPropertiesFrom(pluginProperties)
}
private fun Jdbc.applyPropertiesFrom(
pluginProperties: Map,
namespace: String,
) {
val prefix = "$namespace$JDBC_PREFIX"
pluginProperties.findAndSetProperty(prefix, ::schema)
pluginProperties.findAndSetProperty(prefix, ::driverClassName)
pluginProperties.findAndSetProperty(prefix, ::urlQueryParams)
}
private fun Database.External.applyPropertiesFrom(pluginProperties: Map) {
val prefix = "$WITHOUT_CONTAINER$DATABASE_PREFIX"
pluginProperties.findAndSetProperty(prefix, ::host)
pluginProperties.findAndSetProperty(prefix, ::port) { it.toInt() }
pluginProperties.findAndSetProperty(prefix, ::name)
pluginProperties.findAndSetProperty(prefix, ::username)
pluginProperties.findAndSetProperty(prefix, ::password)
jdbc.applyPropertiesFrom(pluginProperties, prefix)
}
private fun Database.Internal.applyPropertiesFrom(pluginProperties: Map) {
val prefix = "$WITH_CONTAINER$DATABASE_PREFIX"
pluginProperties.findAndSetProperty(prefix, ::port) { it.toInt() }
pluginProperties.findAndSetProperty(prefix, ::name)
pluginProperties.findAndSetProperty(prefix, ::username)
pluginProperties.findAndSetProperty(prefix, ::password)
jdbc.applyPropertiesFrom(pluginProperties, prefix)
}
private fun Image.applyPropertiesFrom(pluginProperties: Map) {
val prefix = "$WITH_CONTAINER$IMAGE_PREFIX"
pluginProperties.findAndSetProperty(prefix, ::name)
pluginProperties.findAndSetProperty(prefix, ::command)
pluginProperties.findAndSetProperty(prefix, ::testQuery)
val envVarsPrefix = "$prefix${::envVars.name}."
pluginProperties
.filterKeys { it.startsWith(envVarsPrefix) }
.map { (key, value) ->
key.removePrefix(envVarsPrefix) to value.toString()
}.takeIf { it.isNotEmpty() }
?.also {
envVars = it.toMap()
}
}
@Suppress("UNCHECKED_CAST")
private fun Map.findAndSetProperty(
prefix: String,
property: KMutableProperty0,
mapper: (String) -> T = { it as T },
) {
get("$prefix${property.name}")?.also {
property.set(mapper(it))
}
}
private fun functionName(ref: KFunction2, Unit>) = ref.name
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy