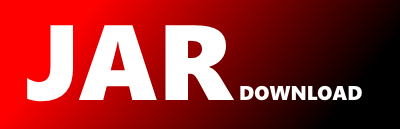
dev.openfga.sdk.api.model.Condition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openfga-sdk Show documentation
Show all versions of openfga-sdk Show documentation
This is an autogenerated Java SDK for OpenFGA. It provides a wrapper around the [OpenFGA API definition](https://openfga.dev/api).
/*
* OpenFGA
* A high performance and flexible authorization/permission engine built for developers and inspired by Google Zanzibar.
*
* The version of the OpenAPI document: 1.x
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package dev.openfga.sdk.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.StringJoiner;
/**
* Condition
*/
@JsonPropertyOrder({
Condition.JSON_PROPERTY_NAME,
Condition.JSON_PROPERTY_EXPRESSION,
Condition.JSON_PROPERTY_PARAMETERS,
Condition.JSON_PROPERTY_METADATA
})
public class Condition {
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_EXPRESSION = "expression";
private String expression;
public static final String JSON_PROPERTY_PARAMETERS = "parameters";
private Map parameters = new HashMap<>();
public static final String JSON_PROPERTY_METADATA = "metadata";
private ConditionMetadata metadata;
public Condition() {}
public Condition name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public Condition expression(String expression) {
this.expression = expression;
return this;
}
/**
* A Google CEL expression, expressed as a string.
* @return expression
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_EXPRESSION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getExpression() {
return expression;
}
@JsonProperty(JSON_PROPERTY_EXPRESSION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setExpression(String expression) {
this.expression = expression;
}
public Condition parameters(Map parameters) {
this.parameters = parameters;
return this;
}
public Condition putParametersItem(String key, ConditionParamTypeRef parametersItem) {
if (this.parameters == null) {
this.parameters = new HashMap<>();
}
this.parameters.put(key, parametersItem);
return this;
}
/**
* A map of parameter names to the parameter's defined type reference.
* @return parameters
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PARAMETERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Map getParameters() {
return parameters;
}
@JsonProperty(JSON_PROPERTY_PARAMETERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setParameters(Map parameters) {
this.parameters = parameters;
}
public Condition metadata(ConditionMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
* @return metadata
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ConditionMetadata getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(ConditionMetadata metadata) {
this.metadata = metadata;
}
/**
* Return true if this Condition object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Condition condition = (Condition) o;
return Objects.equals(this.name, condition.name)
&& Objects.equals(this.expression, condition.expression)
&& Objects.equals(this.parameters, condition.parameters)
&& Objects.equals(this.metadata, condition.metadata);
}
@Override
public int hashCode() {
return Objects.hash(name, expression, parameters, metadata);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Condition {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" expression: ").append(toIndentedString(expression)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `name` to the URL query string
if (getName() != null) {
joiner.add(String.format(
"%sname%s=%s",
prefix,
suffix,
URLEncoder.encode(String.valueOf(getName()), StandardCharsets.UTF_8)
.replaceAll("\\+", "%20")));
}
// add `expression` to the URL query string
if (getExpression() != null) {
joiner.add(String.format(
"%sexpression%s=%s",
prefix,
suffix,
URLEncoder.encode(String.valueOf(getExpression()), StandardCharsets.UTF_8)
.replaceAll("\\+", "%20")));
}
// add `parameters` to the URL query string
if (getParameters() != null) {
for (String _key : getParameters().keySet()) {
if (getParameters().get(_key) != null) {
joiner.add(getParameters()
.get(_key)
.toUrlQueryString(String.format(
"%sparameters%s%s",
prefix,
suffix,
"".equals(suffix)
? ""
: String.format("%s%d%s", containerPrefix, _key, containerSuffix))));
}
}
}
// add `metadata` to the URL query string
if (getMetadata() != null) {
joiner.add(getMetadata().toUrlQueryString(prefix + "metadata" + suffix));
}
return joiner.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy