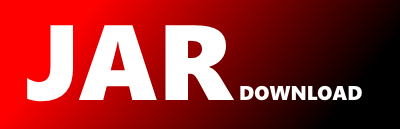
dev.openfga.sdk.telemetry.Metrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openfga-sdk Show documentation
Show all versions of openfga-sdk Show documentation
This is an autogenerated Java SDK for OpenFGA. It provides a wrapper around the [OpenFGA API definition](https://openfga.dev/api).
package dev.openfga.sdk.telemetry;
import dev.openfga.sdk.api.configuration.Configuration;
import io.opentelemetry.api.OpenTelemetry;
import io.opentelemetry.api.metrics.DoubleHistogram;
import io.opentelemetry.api.metrics.LongCounter;
import io.opentelemetry.api.metrics.Meter;
import java.util.HashMap;
import java.util.Map;
/**
* The Metrics class provides methods for creating and publishing metrics using OpenTelemetry.
*/
public class Metrics {
private final Meter meter;
private final Map counters;
private final Map histograms;
private final Configuration configuration;
public Metrics() {
this.meter = OpenTelemetry.noop().getMeterProvider().get("openfga-sdk/0.7.0");
this.counters = new HashMap<>();
this.histograms = new HashMap<>();
this.configuration = new Configuration();
}
public Metrics(Configuration configuration) {
this.meter = OpenTelemetry.noop().getMeterProvider().get("openfga-sdk/0.7.0");
this.counters = new HashMap<>();
this.histograms = new HashMap<>();
this.configuration = configuration;
}
/**
* Returns the Meter associated with this Metrics session.
*
* @return The Meter object.
*/
public Meter getMeter() {
return meter;
}
/**
* Returns a LongCounter metric instance.
*
* @param counter The Counter enum representing the metric.
* @param value The value to be added to the counter.
* @param attributes A map of attributes associated with the metric.
*
* @return The LongCounter metric instance.
*/
public LongCounter getCounter(Counter counter, Long value, Map attributes) {
if (!counters.containsKey(counter.getName())) {
counters.put(
counter.getName(),
meter.counterBuilder(counter.getName())
.setDescription(counter.getDescription())
.build());
}
LongCounter counterInstance = counters.get(counter.getName());
if (value != null) {
counterInstance.add(value, Attributes.prepare(attributes, counter, configuration));
}
return counterInstance;
}
/**
* Returns a DoubleHistogram metric instance.
*
* @param histogram The Histogram enum representing the metric.
* @param value The value to be recorded in the histogram.
* @param attributes A map of attributes associated with the metric.
*/
public DoubleHistogram getHistogram(Histogram histogram, Double value, Map attributes) {
if (!histograms.containsKey(histogram.getName())) {
histograms.put(
histogram.getName(),
meter.histogramBuilder(histogram.getName())
.setDescription(histogram.getDescription())
.setUnit(histogram.getUnit())
.build());
}
DoubleHistogram histogramInstance = histograms.get(histogram.getName());
if (value != null) {
histogramInstance.record(value, Attributes.prepare(attributes, histogram, configuration));
}
return histogramInstance;
}
/**
* Returns a LongCounter counter for tracking the number of times an access token is requested through ClientCredentials.
*
* @param value The value to be added to the counter.
* @param attributes A map of attributes associated with the metric.
*
* @return The LongCounter metric instance for credentials request.
*/
public LongCounter credentialsRequest(Long value, Map attributes) {
return getCounter(Counters.CREDENTIALS_REQUEST, value, attributes);
}
/**
* Returns a DoubleHistogram histogram for measuring the total roundtrip time it took to process a request, including the time it took to send the request and receive the response.
*
* @param value The value to be recorded in the histogram.
* @param attributes A map of attributes associated with the metric.
*/
public DoubleHistogram requestDuration(Double value, Map attributes) {
return getHistogram(Histograms.REQUEST_DURATION, value, attributes);
}
/**
* Returns a DoubleHistogram for measuring how long the FGA server took to process and evaluate a request.
*
* @param value The value to be recorded in the histogram.
* @param attributes A map of attributes associated with the metric.
*/
public DoubleHistogram queryDuration(Double value, Map attributes) {
return getHistogram(Histograms.QUERY_DURATION, value, attributes);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy