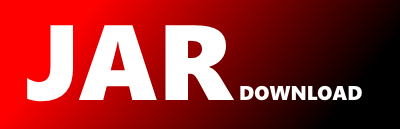
dev.restate.sdk.testing.RestateExtension Maven / Gradle / Ivy
The newest version!
// Copyright (c) 2023 - Restate Software, Inc., Restate GmbH
//
// This file is part of the Restate Java SDK,
// which is released under the MIT license.
//
// You can find a copy of the license in file LICENSE in the root
// directory of this repository or package, or at
// https://github.com/restatedev/sdk-java/blob/main/LICENSE
package dev.restate.sdk.testing;
import java.util.List;
import java.util.regex.Pattern;
import org.junit.jupiter.api.extension.*;
import org.junit.platform.commons.support.AnnotationSupport;
/**
* @see RestateTest
*/
public class RestateExtension implements BeforeAllCallback, ParameterResolver {
static final ExtensionContext.Namespace NAMESPACE =
ExtensionContext.Namespace.create(RestateExtension.class);
static final String RUNNER = "Runner";
@Override
public void beforeAll(ExtensionContext extensionContext) {
extensionContext
.getStore(NAMESPACE)
.getOrComputeIfAbsent(
RUNNER, ignored -> initializeRestateRunner(extensionContext), RestateRunner.class)
.beforeAll(extensionContext);
}
@Override
public boolean supportsParameter(
ParameterContext parameterContext, ExtensionContext extensionContext)
throws ParameterResolutionException {
return extensionContext
.getStore(NAMESPACE)
.getOrComputeIfAbsent(
RUNNER, ignored -> initializeRestateRunner(extensionContext), RestateRunner.class)
.supportsParameter(parameterContext, extensionContext);
}
@Override
public Object resolveParameter(
ParameterContext parameterContext, ExtensionContext extensionContext)
throws ParameterResolutionException {
return extensionContext
.getStore(NAMESPACE)
.getOrComputeIfAbsent(
RUNNER, ignored -> initializeRestateRunner(extensionContext), RestateRunner.class)
.resolveParameter(parameterContext, extensionContext);
}
private RestateRunner initializeRestateRunner(ExtensionContext extensionContext) {
// Discover services
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy