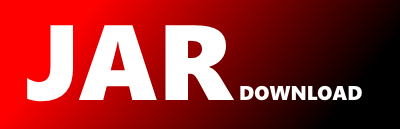
dev.sanda.apifi.generator.client.ApifiClientFactory Maven / Gradle / Ivy
package dev.sanda.apifi.generator.client;
import lombok.val;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
public class ApifiClientFactory {
private List queries = new ArrayList<>();
private String fileName = "apifiClient";
public ApifiClientFactory() {
}
public void addQuery(GraphQLQueryBuilder graphQLQueryBuilder){
if(queries == null) queries = new ArrayList<>();
queries.add(graphQLQueryBuilder);
}
public void generate(){
StringBuilder builder = new StringBuilder();
builder.append("let apiUrl = location.origin + '/graphql';\n");
builder.append("let bearerToken = undefined;\n\n");
builder.append("export default{\n");
builder.append("\n\tsetBearerToken(token){\n\t\tbearerToken = token;\n\t},\n");
builder.append("\n\tsetApiUrl(url){\n\t\tapiUrl = url;\n\t},\n");
queries.forEach(query -> builder.append(generateQueryFetcher(query)));
builder.append("\n}");
val finalContent = builder.toString();
try {
Path path = Paths.get(fileName + ".js");
if(Files.exists(path)) Files.delete(path);
Files.createFile(path);
Files.write(path, finalContent.getBytes());
}catch (Exception e){
e.printStackTrace();
}
}
private String generateQueryFetcher(GraphQLQueryBuilder query) {
return "\n\tasync " + query.getQueryName() + "(" + query.args() + "customHeaders)" +
"{\n" +
"\t\t\tlet requestHeaders = { \"Content-Type\": \"application/json\" }\n" +
"\t\t\tif(customHeaders !== undefined) requestHeaders = Object.assign({}, requestHeaders, customHeaders);\n" +
"\t\t\tif(bearerToken !== undefined) requestHeaders[\"Authorization\"] = bearerToken;\n" +
"\t\t\tlet opts = {\n" +
"\t\t\t\tmethod: \"POST\",\n" +
"\t\t\t\tcredentials: \"include\",\n" +
"\t\t\t\theaders: requestHeaders,\n" +
"\t\t\t\tbody: JSON.stringify({" + query.buildQueryString() + "\t})" +
"\n\t\t\t};\n" +
"\t\t\treturn await (await fetch(apiUrl, opts)).json();" +
"\n\t},\n";
}
public List getQueries() {
return this.queries;
}
public String getFileName() {
return this.fileName;
}
public void setQueries(List queries) {
this.queries = queries;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ApifiClientFactory)) return false;
final ApifiClientFactory other = (ApifiClientFactory) o;
if (!other.canEqual((Object) this)) return false;
final Object this$queries = this.getQueries();
final Object other$queries = other.getQueries();
if (this$queries == null ? other$queries != null : !this$queries.equals(other$queries)) return false;
final Object this$fileName = this.getFileName();
final Object other$fileName = other.getFileName();
if (this$fileName == null ? other$fileName != null : !this$fileName.equals(other$fileName)) return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof ApifiClientFactory;
}
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $queries = this.getQueries();
result = result * PRIME + ($queries == null ? 43 : $queries.hashCode());
final Object $fileName = this.getFileName();
result = result * PRIME + ($fileName == null ? 43 : $fileName.hashCode());
return result;
}
public String toString() {
return "ApifiClientFactory(queries=" + this.getQueries() + ", fileName=" + this.getFileName() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy