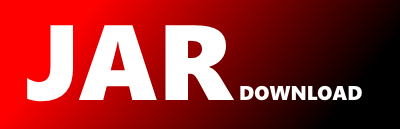
dev.sixpack.client.HmacHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK to develop generators part of the Sixpack solution
The newest version!
package dev.sixpack.client;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import static java.nio.charset.StandardCharsets.UTF_8;
public class HmacHelper {
public static Mac macFactory(String apiKey) {
if (apiKey == null) {
throw new IllegalArgumentException("The API Key provided is null");
}
if (apiKey.isEmpty()) {
throw new IllegalArgumentException("The API Key provided is empty");
}
try {
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(new SecretKeySpec(apiKey.getBytes(UTF_8), "HmacSHA256"));
return mac;
} catch (NoSuchAlgorithmException | InvalidKeyException e) {
throw new RuntimeException("Failed to initialize HMAC-SHA256", e);
}
}
private static final char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
public static String bytesToHex(byte[] bytes) {
char[] hexChars = new char[bytes.length * 2];
for (int j = 0; j < bytes.length; j++) {
int v = bytes[j] & 0xFF;
hexChars[j * 2] = HEX_ARRAY[v >>> 4];
hexChars[j * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
return new String(hexChars);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy