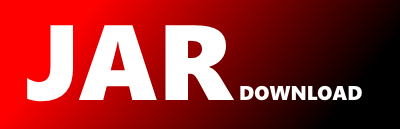
dev.sixpack.generator.Factory Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package dev.sixpack.generator;
import dev.sixpack.api.data.Context;
import dev.sixpack.api.exception.IterateRequest;
import dev.sixpack.api.data.Template;
import dev.sixpack.api.exception.NonRetryableException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.InvocationTargetException;
import java.time.Duration;
import java.util.List;
public class Factory implements FactoryInterface {
protected final Logger LOGGER = LoggerFactory.getLogger(getClass()); // TODO: to replay-aware logger
private String environment;
/**
* Returns the environment name that was passed to the generator.
*/
final protected String getEnvironment() {
return environment;
}
final void setEnvironment(String environment) {
this.environment = environment;
}
/**
* Main method that generates data based on a single input object and an optional Context as a second parameter.
* It returns references to the generated data.
*
* The context object allows to get additional information including custom details that can be updated between
* iterations. In normal scenario iterations are not used. Please check @link{#haltAndIterateAfter(Duration)}
* for more details about iterations.
*
* Note: Instead of overriding this method, create one with specific input and output types. This method will invoke
* your method. In case you declare both methods with Context and without Context the one with Context will be invoked.
*/
final public Object generate(Object input, Context context) {
try {
try {
return getClass().getDeclaredMethod("generate", input.getClass(), Context.class)
.invoke(this, input, context);
} catch (NoSuchMethodException e) {
try {
return getClass().getDeclaredMethod("generate", input.getClass())
.invoke(this, input);
} catch (NoSuchMethodException e1) {
throw new RuntimeException("No method generated was found", e1);
}
}
} catch (IllegalAccessException e) {
throw new RuntimeException("The SDK could not access the method generate", e);
} catch (InvocationTargetException e) {
if (IterateRequest.class.isAssignableFrom(e.getTargetException().getClass())) {
throw (IterateRequest) e.getTargetException();
} else if (NonRetryableException.class.isAssignableFrom(e.getTargetException().getClass())) {
throw (NonRetryableException) e.getTargetException();
} else if (RuntimeException.class.isAssignableFrom(e.getTargetException().getClass())) {
throw (RuntimeException) e.getTargetException();
} else if (Error.class.isAssignableFrom(e.getTargetException().getClass())) {
throw (Error) e.getTargetException();
} else {
throw new RuntimeException("The method generate threw an exception", e.getTargetException());
}
}
}
/**
* Throws an unchecked exception that will be caught by the SDK and will cause the generator to be re-invoked after
* the specified timeout. In case the method generate takes Context as second argument the next iteration will
* receive the Context that might have been modified by the previous iteration. This allows to share data between
* iterations.
*
* For example this may be used as polling mechanism to verify that test data were processed by some system before
* returning them to Sixpack.
*
* @param timeout the specified timeout
*/
final protected void haltAndIterateAfter(Duration timeout) {
throw new IterateRequest(timeout);
}
/**
* see {@link #haltAndIterateAfter(Duration)}
*
* @param message A message that will be displayed in the UI as progress information
*/
final protected void haltAndIterateAfter(Duration timeout, String message) {
throw new IterateRequest(timeout, message);
}
/**
* Allows to specify a list of templates representing datasets that should be pre-generated with minimum counts.
* You can or not override the method from the super class Generator, the result will be the same.
*
* To not generate any data upfront, return an empty list.
*/
public List templates() {
return null;
}
}