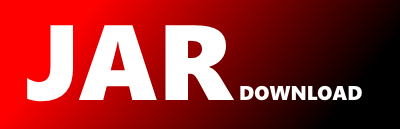
dev.sixpack.generator.Orchestrator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK to develop generators part of the Sixpack solution
The newest version!
package dev.sixpack.generator;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import dev.sixpack.api.data.Configuration;
import dev.sixpack.api.data.Dataset;
import dev.sixpack.api.exception.ObtainFailedException;
import dev.sixpack.api.rpc.ObtainW;
import io.temporal.workflow.Workflow;
import java.util.Map;
import static dev.sixpack.utils.Deterministic.deterministicNow;
public class Orchestrator extends Factory implements OrchestratorInterface {
private static final ObjectMapper MAPPER = new ObjectMapper();
static {
MAPPER.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
MAPPER.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
MAPPER.registerModule(new JavaTimeModule());
}
private String datasetId;
void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
@Override
final public T obtain(String supplier,
String item,
Class returnType,
Object configuration) {
ObtainW obtainW = Workflow.newChildWorkflowStub(
ObtainW.class,
ObtainW.getChildOptions());
LOGGER.debug("Requesting dataset {}/{} with input {}",
supplier, item, configuration);
Dataset result = obtainW.obtain(Dataset.builder()
.parent(datasetId)
.environment(getEnvironment())
.supplier(supplier)
.item(item)
.input(MAPPER.convertValue(configuration, Configuration.class))
.requestDate(deterministicNow())
.build());
LOGGER.debug("Obtained output {}", result);
if (result.getStatus().equals(Dataset.DatasetStatus.FAILED)) {
LOGGER.debug("Dataset {}/{} with input {} obtain FAILED due to item's generator failure.", supplier, item, configuration);
throw new ObtainFailedException(result.getProgress(), supplier, item);
}
return MAPPER.convertValue(result.getOutput(), returnType);
}
@Override
final public Map obtain(String supplier,
String item,
Object configuration) {
return obtain(supplier, item, Map.class, configuration); // TODO: handle unchecked assignment
}
// TODO: use that in the workflow interceptor:
// catch (NonDeterministicException e1) {
// LOGGER.error("Your orchestrator has non-deterministic code accessing some external system or using time or random functions. Abandoning the whole workflow. Please documentation at https://docs.sixpack.dev to see how to maintain determinism of your orchestration code while still being able to access external systems or date and random functions.");
// throw ApplicationFailure.newNonRetryableFailure("Abandoning workflow due to non-determinism", "Determinism issue");
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy