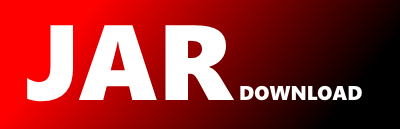
dev.sixpack.generator.OrchestratorRecordBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK to develop generators part of the Sixpack solution
The newest version!
package dev.sixpack.generator;
import dev.sixpack.api.data.Template;
import dev.sixpack.api.exception.SixpackFatalException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import static dev.sixpack.utils.FormatUtils.mFormat;
public class OrchestratorRecordBuilder {
private final String environment;
private final String supplier;
final private Class extends Orchestrator> orchestratorClass;
public OrchestratorRecordBuilder(String environment,
String supplier,
Class extends Orchestrator> orchestratorClass) {
this.environment = environment;
this.supplier = supplier;
this.orchestratorClass = orchestratorClass;
}
public FactoryRecord build() {
return new FactoryRecord(
environment,
supplier,
itemNameAnnotation(),
inputType(),
null, // TODO: implement
null, // TODO: implement
outputType(),
null,
orchestratorClass,
templates());
}
private String itemNameAnnotation() {
ItemName annotation = orchestratorClass.getAnnotation(ItemName.class);
if (annotation == null) {
throw new IllegalArgumentException(mFormat(
"The generator class {} is not annotated with @ItemName. This annotation is used to uniquely identify item within the scope of this supplier on the given test environment. Please carefully chose the value and add the annotation @ItemName.",
orchestratorClass.getSimpleName()));
}
return annotation.value();
}
private Class> inputType() {
return getMethodGenerate().getParameterTypes()[0];
}
private Class> outputType() {
return getMethodGenerate().getReturnType();
}
private List templates() {
try {
//noinspection unchecked
return (List) methodTemplates().invoke(
orchestratorClass.getDeclaredConstructor().newInstance());
} catch (NoSuchMethodException | InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
}
private Method getMethodGenerate() {
return Arrays.stream(orchestratorClass.getDeclaredMethods())
.filter(method -> method.getName().equals("generate"))
.filter(method -> method.getParameterCount() == 1)
.findFirst()
.orElseThrow(() -> new SixpackFatalException("Method generate not found"));
}
private Method methodTemplates() {
try {
return orchestratorClass.getMethod("templates");
} catch (NoSuchMethodException e) {
throw new SixpackFatalException("Method templates not found");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy