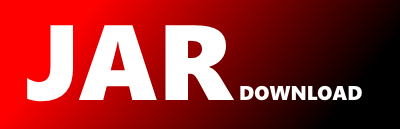
dev.sixpack.api.data.Dataset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sixpack-sdk Show documentation
Show all versions of sixpack-sdk Show documentation
SDK to develop generators part of the Sixpack solution
The newest version!
package dev.sixpack.api.data;
import dev.sixpack.client.Sixpack;
import lombok.*;
import java.time.Instant;
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@Builder
public class Dataset {
@EqualsAndHashCode.Include
private String id;
private DatasetStatus status;
private String progress;
private String parent;
private String user;
private String environment;
private String supplier;
private String item;
private Instant requestDate;
private Instant generationDate;
private Instant allocationDate;
private Instant configurationDate;
private Instant cleanupDate;
private Configuration input;
private Configuration configuration;
private Configuration output;
/**
* Refreshes the dataset from the server in place.
*
* @return the same object but with updated fields
*/
@SuppressWarnings("unused")
public Dataset refreshFromServerNow() {
Sixpack.refreshDataset(this, System.currentTimeMillis());
return this;
}
/**
* Refreshes the dataset from the server in place. The server will wait until the deadline specified or until the dataset is fulfilled. If the dataset is not ready by the deadline, the method will return the dataset in the latest state. This can be checked by the status field.
*
* @param waitUntilMillis The time in milliseconds to wait for the dataset to be ready, maximum 30s ahead.
* @return The same object but with updated fields
*/
@SuppressWarnings("UnusedReturnValue")
public Dataset refreshFromServerWaitUntil(long waitUntilMillis) {
Sixpack.refreshDataset(this, waitUntilMillis);
return this;
}
@SuppressWarnings("UnusedReturnValue")
public Dataset refreshFromServerWaitFor(long waitForMillis) {
Sixpack.refreshDataset(this, System.currentTimeMillis() + waitForMillis);
return this;
}
@SuppressWarnings("unused")
public T outputAs(Class clazz) {
return Sixpack.Utils.mapConfigurationToObject(output, clazz);
}
public void updateFrom(Dataset dataset) {
this.status = dataset.status;
this.progress = dataset.progress;
this.parent = dataset.parent;
this.user = dataset.user;
this.environment = dataset.environment;
this.supplier = dataset.supplier;
this.item = dataset.item;
this.requestDate = dataset.requestDate;
this.generationDate = dataset.generationDate;
this.allocationDate = dataset.allocationDate;
this.configurationDate = dataset.configurationDate;
this.cleanupDate = dataset.cleanupDate;
this.input = dataset.input;
this.configuration = dataset.configuration;
this.output = dataset.output;
}
public enum DatasetStatus {
NEW,
ACCEPTED,
AWAITED,
STOCKED,
REQUESTED,
FULFILLED,
ENVIRONMENT_DISCONTINUED,
SUPPLIER_DISCONTINUED,
ITEM_DISCONTINUED,
TIMED_OUT,
CANCELLED,
NOT_FOUND,
FAILED;
public boolean isFinal() {
return this == FULFILLED
|| this == ENVIRONMENT_DISCONTINUED
|| this == SUPPLIER_DISCONTINUED
|| this == ITEM_DISCONTINUED
|| this == TIMED_OUT
|| this == CANCELLED
|| this == NOT_FOUND
|| this == FAILED;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy