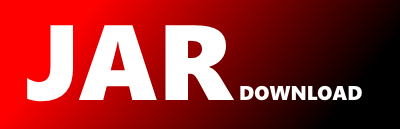
dev.sixpack.generator.GeneratorRecordBuilder Maven / Gradle / Ivy
package dev.sixpack.generator;
import dev.sixpack.api.data.Context;
import dev.sixpack.api.data.Template;
import dev.sixpack.api.exception.SixpackFatalException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import static dev.sixpack.utils.FormatUtils.mFormat;
public class GeneratorRecordBuilder {
private final String environment;
private final String supplier;
final private Generator generator;
public GeneratorRecordBuilder(String environment,
String supplier,
Generator generator) {
this.environment = environment;
this.supplier = supplier;
this.generator = generator;
}
public FactoryRecord build() {
try {
return new FactoryRecord(
environment,
supplier,
itemNameAnnotation(),
inputType(),
null, // TODO: implement
null, // TODO: implement
outputType(),
generator,
null,
templates());
} catch (IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException("Error calling method to construct generator's manifest", e);
}
}
private String itemNameAnnotation() {
ItemName annotation = generator.getClass().getAnnotation(ItemName.class);
if (annotation == null) {
throw new IllegalArgumentException(mFormat(
"The generator class {} is not annotated with @ItemName. This annotation is used to uniquely identify item within the scope of this supplier on the given test environment. Please carefully chose the value and add the annotation @ItemName.",
generator.getClass().getSimpleName()));
}
return annotation.value();
}
private Class> inputType() {
return getMethodGenerate().getParameterTypes()[0];
}
private Class> outputType() {
return getMethodGenerate().getReturnType();
}
private List templates() throws IllegalAccessException, InvocationTargetException {
//noinspection unchecked
return (List) methodTemplates().invoke(generator);
}
private Method getMethodGenerate() {
Method methodGenerateWithContext = Arrays.stream(generator.getClass().getDeclaredMethods())
.filter(method -> method.getName().equals("generate"))
.filter(method -> method.getParameterCount() == 2
&& Context.class.isAssignableFrom(method.getParameterTypes()[1]))
.findFirst()
.orElse(null);
if (methodGenerateWithContext != null) return methodGenerateWithContext;
return Arrays.stream(generator.getClass().getDeclaredMethods())
.filter(method -> method.getName().equals("generate"))
.filter(method -> method.getParameterCount() == 1)
.findFirst()
.orElseThrow(() -> new SixpackFatalException("Method generate not found"));
}
private Method methodTemplates() {
try {
return generator.getClass().getMethod("templates");
} catch (NoSuchMethodException e) {
throw new SixpackFatalException("Method templates not found");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy