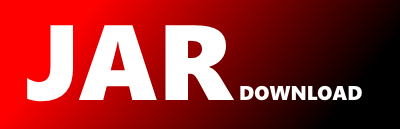
dev.soffa.foundation.model.DataPoint Maven / Gradle / Ivy
package dev.soffa.foundation.model;
import lombok.Data;
import java.time.Instant;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
@Data
public class DataPoint {
private final String metric;
private final Map tags = new HashMap<>();
private final Map fields = new HashMap<>();
private Date time;
public DataPoint(String metric) {
this.time = Date.from(Instant.now());
this.metric = metric;
}
public static DataPoint metric(String name) {
return new DataPoint(name);
}
public DataPoint addTags(Map tags) {
this.tags.putAll(tags);
return this;
}
public DataPoint addFields(Map fields) {
this.fields.putAll(fields);
return this;
}
public DataPoint addTag(String name, String value) {
tags.put(name, value);
return this;
}
public DataPoint addField(String name, long value) {
fields.put(name, value);
return this;
}
public DataPoint addField(String name, double value) {
fields.put(name, value);
return this;
}
public DataPoint addField(String name, boolean value) {
fields.put(name, value);
return this;
}
public DataPoint addField(String name, CharSequence value) {
fields.put(name, value);
return this;
}
public DataPoint time(Date value) {
this.time = value;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy