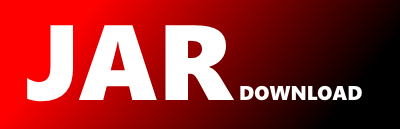
dev.tauri.choam.internal.mcas.PaddedMemoryLocation Maven / Gradle / Ivy
The newest version!
/*
* SPDX-License-Identifier: Apache-2.0
* Copyright 2016-2024 Daniel Urban and contributors listed in NOTICE.txt
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dev.tauri.choam.internal.mcas;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.ref.WeakReference;
import dev.tauri.choam.internal.VarHandleHelper;
final class PaddedMemoryLocation
extends PaddedMemoryLocationPadding
implements MemoryLocation {
private static final VarHandle VALUE;
private static final VarHandle VERSION;
private static final VarHandle MARKER;
static {
try {
MethodHandles.Lookup l = MethodHandles.lookup();
VALUE = VarHandleHelper.withInvokeExactBehavior(l.findVarHandle(PaddedMemoryLocation.class, "value", Object.class));
VERSION = VarHandleHelper.withInvokeExactBehavior(l.findVarHandle(PaddedMemoryLocation.class, "version", long.class));
MARKER = VarHandleHelper.withInvokeExactBehavior(l.findVarHandle(PaddedMemoryLocation.class, "marker", WeakReference.class));
} catch (ReflectiveOperationException e) {
throw new ExceptionInInitializerError(e);
}
}
private final long _id;
private volatile A value;
private volatile long version = Version.Start;
private volatile WeakReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy